|
2023-2024 College Catalog [ARCHIVED CATALOG]
Course Descriptions
|
|
Legend for Courses
HC/HN: Honors Course IN/IH: Integrated lecture/lab LB: Lab LC: Clinical Lab LS: Skills Lab WK: Co-op Work
SUN#: is a prefix and number assigned to certain courses that represent course equivalency at all Arizona community colleges and the three public universities. Learn more at www.aztransfer.com/sun.
|
|
Computer Information Systems |
|
-
CIS 278 - C++ and Object-Oriented Programming 4 Credits, 4 Contact Hours 4 lecture periods 0 lab periods
Concepts and implementation of object-oriented programming and design using C++ Includes the language syntax of C++ applications using C++ objects to solve information systems problems, and class libraries created for reuse and inheritance.
Gen-Ed: Meets AGEC Options requirement; Meets CTE - Options requirement
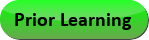.png)
Course Learning Outcomes
- Develop programs using both C++ built-in classes and user-defined classes.
- Integrate the concepts of abstraction, inheritance, composition and polymorphism into C++ programs.
- Write programs in C++ which solve information systems problems and which show increased productivity by taking an object-oriented approach.
- Create and use collections (arrays and vectors) of user-defined objects.
Outline:
- Introduction and Overview
- Benefits of object-oriented (O-O) methods
- Structured vs. O-O approaches
- O-O design improvements
- Reusability
- Reliability
- Maintainability
- Encapsulation: integrating object state data, as represented by instance variables, with the code that operates upon it
- Features of object-oriented programs
- Strong typing and type hierarchies
- Classes for encapsulation and information hiding
- Using C++
- Introduction to C++
- Design goals of C++
- C++ = C + strong typing + classes
- C++ syntax
- C++ as a better C (small enhancements)
- C++ structs as a step on the path towards classes
- Classes as user-defined types in C++
- O-O programming in C++
- Using C++ built-in classes such as strings
- C++ types, references, and friends
- Object creation (constructors, copy constructors, destructors)
- Inheritance and derived classes
- Composition: using objects of other classes as instance variables within a class
- Dynamic storage allocation of objects
- Polymorphism
- Collections of objects
- Dynamic function binding using virtual and pure virtual functions
- Advanced C++ Features and the Future of O-O
- Reusable libraries
- Files, stream, and I/O libraries
- Designing a library
- The Standard Template Library
- Container classes
- Iterators
- Algorithms
- Generic Libraries
- Using a container class
- Class categories as mechanisms
- Database operations
- Connecting to an external database
- Executing SQL statements from within a program
Effective Term: Fall 2023 |
|
|
|
-
CIS 280 - Systems Analysis and Design: Concepts and Tools 3 Credits, 3 Contact Hours 3 lecture periods 0 lab periods
Concepts of systems analysis and design for all phases of the systems development life cycle. Includes problem identification, project initiation and planning, analysis, logical design, physical design, implementation and testing, and operations and maintenance. Also includes specific tools used by systems analysts, introduction and use of CASE (computer-aided software engineering) tools, and project management software.
Prerequisite(s): CIS 131 or CIS 162 . Gen-Ed: Meets AGEC Options requirement; Meets CTE - Options requirement
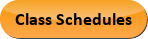
Course Learning Outcomes
- Identify the elements of the SDLC (System Development Life Cycle).
- Identify the major tasks of each phase of the SDLC.
- Explain the process of managing an information systems project.
Outline:
- Systems Development Overview
- Types of information systems
- Systems development life cycle (SDLC)
- The Systems Analyst
- Skills of systems analysts
- Systems analysis as a profession
- Information Systems Project Management
- Project management
- Project planning
- Critical path scheduling
- Gantt and PERT charts
- Overview of CASE Tools
- CASE tools and the SDLC
- CASE tool types
- Selecting Information Systems Projects
- The project identification and selection process
- Relationship between corporate strategic planning IS planning
- Planning Systems Development Projects
- Feasibility studies
- Cost-benefit analysis
- Determining System Requirements
- Interviews
- Questionnaires
- Automated data collection
- Joint application design
- Process Modeling: Data Flow Diagrams (DFDs)
- Logic Modeling: Decision Tables and Trees
- Conceptual Data Modeling: Entity Relationship (E-R) Diagrams
- Selecting Alternative Designs
- Rapid Application Development
- Designing Forms and Reports Design
- User Interface Design
- Logical Database Design
- Physical Database Design
- Program and Process Design
- Coupling and cohesion
- Representing Logic with Pseudocode
- Distributed System Design
- System Implementation
- Coding, testing, and conversion
- Test planning
- Installation strategies
- System Maintenance
Effective Term: Fall 2023 |
|
-
CIS 281 - Systems Analysis and Design: Applications 3 Credits, 5 Contact Hours 1 lecture periods 4 lab periods
Systems analysis concepts applied to specific software projects. Includes completing a software project from beginning to end, from problem identification to project implementation, using current methodologies and appropriate software development tools.
Prerequisite(s): CIS 280 Gen-Ed: Meets AGEC Options requirement; Meets CTE - Options requirement
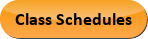
Course Learning Outcomes
- Build a software prototype that solves real-world problems.
- Deliver a presentation that solves a real-world problem including the deliverables from each phase of SDLC (Software Development Life Cycle).
Outline:
- Review of Concepts and Tools of Systems Analysis and Design
- Project Selection
- Project Planning
- Analysis
- Process Model Using DFDs
- Data Model Using E-R Diagrams
- Object-Oriented Modeling
- Design
- Complete Design-Phase Diagrams
- Database Design
- Security Considerations
- Prototyping
- Implementation and Testing
- Maintenance
Effective Term: Fall 2023 |
|
-
CIS 284 - Information Technology Capstone 1-3 Credits, 1.5-5.0 Contact Hours .5-1 lecture periods 1-4 lab period
Capstone experience for the CyberSecurity and Networking/Cyber Defense Associates of Applied Science. Provides an opportunity to reflect on and integrate the knowledge gained from previous courses into a final hands-on project.
Information: This course should be taken within the final two semesters of the degree and requires an understanding of operating systems, networking, and cyber security. Course activities may take place in a simulated work setting. Gen-Ed: Meets AGEC Options requirement; Meets CTE - Options requirement
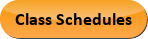
Course Learning Outcomes
- Develop a team-based project plan in the area of systems, networks, or security.
- Implement a technical project based on business requirements.
- Demonstrate an understanding of the system lifecycle.
Outline:
- Project Design & Research
- Identify an enterprise-level data center or security project.
- Determine business requirements for the project, including outcomes, stakeholders & budget.
- Determine technical, procedural, communication and training tasks to be completed.
- Work with team members to assign tasks and set timelines.
- Present project management plan.
- Project Implementation
- Communicate project status on a regular basis through status updates, documentation, and presentations.
- Work with other teams to ensure technical resources are available when needed.
- Develop documentation covering implementation and maintenance.
- Develop training materials for students who would be supporting the system in the future.
- Project Reporting
- Develop a final reflection report which includes an introspective summary of technical and non-technical experiences during the course.
- Deliver final presentation covering:
- Original scope and requirements
- Summary of the project implementation
- Changes to the scope or requirements
- Lessons learned during implementation
Effective Term: Fall 2023 |
|
-
CIS 288 - Fundamentals of Cybersecurity 4 Credits, 4 Contact Hours 4 lecture periods 0 lab periods
Introduction to cyber security policy, doctrine, and operational constraints. Includes a broad survey of networking principles, cybersecurity concepts, tools, technologies, and best practices. Also includes hands-on activities to enhance familiarity with networking concepts and practice cybersecurity techniques and procedures.
Information: This course is designed to meet the University of Arizona South CYBV 301 requirement and is preparatory coursework for the UA South Cyber Operations program. Please see a financial aid or Veteran’s Affairs advisor to determine funding eligibility as appropriate. Gen-Ed: Meets AGEC Options requirement; Meets CTE - Options requirement
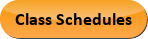
Course Learning Outcomes
- Compare the different types of cyberattacks.
- Explain the major U.S, and International laws governing cyberspace, the restrictions they place on cyber operations, and how they impact an organization’s overall cyber defensive strategy.
- Describe the concepts and best practices of a Defense in Depth strategy.
- Explain the Vulnerability-Threat-Control Paradigm.
- Describe Confidentiality-Integrity-Availability (C-I-A) security triad.
- Explain the similarities and differences between OSI and TCP/IP Model.
- Explain security shortcomings and flaws in networking hardware and devices.
- Demonstrate methods to secure infrastructure, hosts, networks, and the perimeter.
Performance Objectives:
- Define and explain the Vulnerability-Threat-Control Paradigm.
- Identify and describe the trade-offs in the (CIA) Confidentiality-Integrity-Availability security triad.
- Identify and describe the concepts and best practices of a Defense in Depth strategy.
- Identify and describe the types of malware, how malware spreads, and how to mitigate its effects.
- Identify and describe the types of networks to include LAN, WAN, MAN, PAN, NAN, WLAN, and the internet.
- Describe and explain the capabilities, characteristics and security flaws of network hardware devices and their operating systems.
- Identify and explain how to design basic network architectures.
- Identify and explain the similarities and differences between the OSI & the TCP/IP Model.
- Identify and describe the basic functions, uses, and characteristics of network protocols.
- Identify and describe the history and fundamentals of cryptography.
- Identify and describe the different types of cyberattacks.
- Describe and explain the active cyber defense cycle’s techniques and mitigation strategies.
- Identify and explain the major U.S, and International laws governing cyberspace, the restrictions they place on cyber operations, and how they can impact an organizations overall defensive strategy.
Outline:
- Vulnerability-Threat-Control Paradigm
- Vulnerability-Threat-Control Paradigm
- CIA triad
- Types of threats and threat actors
- Threat Method-Opportunity-Motive
- Identify and mitigate harm through risk management
- Concepts and best practices of a Defense in Depth strategy
- Uniform Protection
- Protected Enclaves
- Information Centric
- Threat Vector Analysis
- Viruses, Worms, Trojans & other Malware
- Types of malware, how it spreads
- Viruses
- Worms
- Trojans
- Ransomware
- Capabilities and goals of different types of malware
- Data harvesting
- Unauthorized system access
- Denial of Service, Distributed Denial of Service (DOS, DDOS) and its effects on availability
- Data destruction
- Malware mitigation and prevention strategies
- System scanning and monitoring
- Data integrity checks
- Program execution blocking
- System patching & hardening
- User education and security culture (e.g., social engineering techniques)
- Network Fundamentals
- Types of networks to include LAN, WAN, MAN, PAN, NAN, WLAN, and the Internet
- Capabilities, characteristics and security limitations of network hardware devices and their operating systems
- Hubs
- Bridges
- Switches
- Routers
- Firewalls
- Techniques, methods, and systems for fighting malware
- Intrusion Detection Systems (IDS)
- Intrusion Prevention Systems (IPS)
- Honeypots
- Designing basic network architectures
a. Identifying functionality
b. modularity of design
c. hierarchical design principles in network architectures
d. using VLANs (virtual local area networks) to limit broadcasts
- Protocol Stacks and IP Concepts
- Introduction and Use of the 7-layered Open Systems Interconnect (OSI) model for Networking
- Physical Layer
- Data Link Layer
- Network Layer
- Transport Layer
- Session Layer
- Presentation Layer
- Application Layer
- Relation of the 7-layered OSI Model to the four layers of the TCP/IP (Transmission Control Protocol/Internet Protocol) Model
- Protocol functions, uses, and their operation in the OSI Model
- Media Access Control (MAC) Addressing in local area networks
- ARP (Address Resolution Protocol)
- Internet Protocol (IP)
- Transmission Control Protocol (TCP)
- User Datagram Protocol (UDP)
- Internet Control Message Protocol (ICMP)
- Simple Network Management Protocol (SNMP)
- Border Gateway Protocol (BGP)
- Similarities and differences between the IPv4 vs. IPv6 standards.
- Function of the Domain Name System (DNS) and its support of network communications.
- Cryptography & Securing data at rest and on the move
- History and fundamentals of cryptography
- Communications challenges and cryptographic goals
- Plain text vs. Cipher text
- History
- Ciphers and Cryptanalysis systems
- One-Time Pads
- Cryptography vs. Cryptology
- Cryptosystems
- Keys
- Key Exchange and Protection
- Define and explain the types of Cryptographic Systems
- Symmetric Encryption (Private Key Encryption)
- Asymmetric Encryption (Public Key Encryption)
- Diffie-Hellman Key Exchange
- Hash Functions
- Digital Signatures
- Major capabilities, limitations, characteristics, and usages of common cryptographic algorithms.
- Capabilities, characteristics, and security vulnerabilities presented by Steganography techniques and tools.
- History of steganography
- Steganography techniques
- Open-Source tools for studying steganography
- Cyber Attacks, Defenses, and Law
- Classification of Cyber Attacks
- Network based attacks
- Client side attacks
- Social Engineering attacks
- Active cyber defense cycle’s techniques and mitigation strategies
- Proper network architectures
- Implementation of passive defenses
- Cyber Threat Intelligence (CTI)
- Network Security Monitoring (NSM)
- Incident Response (IR)
- Threat and Environment Manipulation (TEM)
- Introduction to U.S. Federal/State laws governing cyberspace, and relating these laws to international laws.
- Restrictions on cyber operations
- Organizational impact
- Organizational defensive strategies
Effective Term: Fall 2023 |
|
-
CIS 299 - Advanced Co-op: Computer Information Systems 1 Credits, 1 Contact Hours 1 lecture period 0 lab periods
A supervised cooperative work program for students in related occupation area. Teacher-coordinators work with students and their supervisors. Variable credit is available by special arrangement.
Information: May be taken two times for a maximum of two credit hours. If this course is repeated, see a financial aid or Veteran’s Affairs advisor to determine funding eligibility as appropriate.
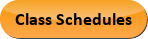
Course Learning Outcomes
- Discuss the need for skills in oral and written communication and the importance to job success.
- Describe the techniques for managing time and energy for job efficiency.
- Identify stress in work situations and begin to develop techniques for coping with stress.
- Find and related information on some career field to career goals.
- Write a resume and plan an employment interview (real or simulated) and observe (real or filmed) or successfully complete such experiences (s), where available.
- Identify some basic principles and theories learned in courses completed, and apply them to problems encountered in real work situations.
- Identify problems which arise in work situations and develop some techniques for successful solution to them.
Outline:
- Communication Skills
- Importance in job success
- Oral skills developed
- Written skills developed
- Time and Energy Management
- Identifying resources and their uses
- Techniques for managing, for job efficiency
- Stress and Its Management
- Types of job stress
- Causes of stress
- Characteristics of stress
- Techniques for managing job stress
- Careers: Information and Its Uses
- Review of careers in field of study
- Sources of career information
- Uses of career information
- Career objectives
- Career plans
- Placing Yourself on the Job Market
- Identifying varied job markets
- Selecting job markets appropriate to your potential
- Aspects of presenting oneself on the market
- Job information
- Resume writing
- Backing up the resume
- References
- The interview
- Principles, Theories, and Practices in the Career Field
- Application in the work situation
- Ongoing discussion
- Understanding through application
- Problems in the Work Situation
- Problem (types) identified
- Ways of dealing with problems encountered – ongoing discussion each session
Effective Term: Fall 2023 |
|
-
CIS 299WK - Adv Co-op Work: Comp Info Sys 1-8 Credits, 5-40 Contact Hours 0 lecture periods 5-40 lab periods
Advanced Co-op Work: Computer Information Systems Introduction to Cooperative Education for second-year students (instruction which provides for success in securing and retaining a training job related to subject area). Includes communication skills, time and energy management, stress and its management, careers: information and its uses, job market, principles, theories, and practices in the career field, and problems in the work situation.
Corequisite(s): CIS 299 Information: May be taken two times for a maximum of sixteen credit hours. If this course is repeated, see a financial aid or Veteran’s Affairs advisor to determine funding eligibility as appropriate. Gen-Ed: Meets AGEC Options requirement; Meets CTE - Options requirement
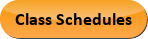
Course Learning Outcomes
- Apply some of the principles, knowledge, and skills learned in classroom and laboratory in real work situations.
- Demonstrate improved skills, competence, and levels of accuracy in handling responsibility and work assignments.
- Demonstrate improved self-confidence in handling work assignments.
- Demonstrate skills in managing human relations: peers (children, customers, clients, etc.) and supervisors.
- Deal responsibly with the world of work: reporting promptly and management of time, energy, and stress.
- Demonstrate improved understanding of the career field.
Outline: Students are assigned to work (5 hours per credit per week) in a selected field experience job, which is appropriate to their program of study and their level of readiness to enter the world of work. They will meet with the instructor and on-site supervisor to enhance growth and evaluate progress. Weekly seminars with other students in the Cooperative Education/field experience or practicum will provide further insights and growth. Evaluation will be based on each student’s planned objectives and activities for the experience.
Effective Term: Fall 2023 |
Computer Software Applications |
|
-
CSA 100 - Computer Literacy 1 Credits, 1.5 Contact Hours .5 lecture periods 1 lab period
Overview of computer applications and functions. Includes components of a computer system, spreadsheet, database, and word processing. Also includes using online information responsibly and securely.
Recommendation: Completion of basic computer and keyboard skills, completion of REA 091 or satisfactory score on the reading assessment test before enrolling in this course. If any recommended course is taken, see a financial aid or Veteran’s Affairs advisor to determine funding eligibility as appropriate. Information: CSA 100 is a one credit version of CIS 104
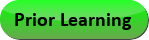.png)
Course Learning Outcomes
- Describe the essential components of digital systems.
- Explain how to manage and protect a digital identity.
- Demonstrate basic operating system skills.
- Create documents, spreadsheets, and presentations.
- Use the Internet for e-mail and basic research using the World Wide Web.
Outline:
- Components of a Computer System
- Hardware
- Peripheral, Input and Output devices
- Operating System Basics
- File management
- Using windows
- Starting applications
- Word Processing Fundamentals
- Functions of a word processor
- Composing documents on the computer
- Saving and opening files
- Correcting errors and moving text
- Spreadsheet Fundamentals
- Functions of spreadsheet software
- Basics of spreadsheet operations
- Formats for computerized data displays
- Accuracy in entering and retrieving data
- Presentation Graphics Fundamentals
- Functions of presentation software
- Presentation basic principles
- Creating presentations
- Saving and opening files
- Internet Fundamentals
- Using E-mail and online communities
- Searching for information
- Critical evaluation of Internet information
- Communicating online
- Safety, security and privacy while online
Effective Term: Fall 2023 |
|
-
CSA 110 - Spreadsheets: Microsoft Excel 3 Credits, 4 Contact Hours 2 lecture periods 2 lab periods
Fundamentals of spreadsheet applications using Microsoft Excel. Includes spreadsheet concepts, formulas and functions, formatting worksheets and cells, working with charts and graphics. Also includes Excel lists, managing multiple worksheets and workbooks, collaborating on a workbook, developing an Excel application, data tables and Scenario management, using Solver, importing data, and advanced functions and filtering.
Prerequisite(s): MAT 086 or required score on the Mathematics assessment test. Recommendation: Basic computer skills, completion of WRT 101 , REA 091 or required score on the assessment test before enrolling in this course. If any recommended course is taken, see a financial aid or Veteran’s Affairs advisor to determine funding eligibility as appropriate.
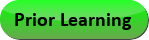.png)
Course Learning Outcomes
- Describe and demonstrate spreadsheet concepts in Excel.
- Construct formulas using Excel functions.
- Format and print worksheets, data, charts and graphics
- Manage multiple worksheets and workbooks.
- Develop an Excel application including data validation and macros.
- Conduct data analysis using data tables.
- Demonstrate the use of advanced logical functions,filtering and lists.
Outline:
- Spreadsheet Concepts and Microsoft Excel
- Using Excel to manage data
- Worksheet navigation and design
- Developing a worksheet
- Entering and editing data
- Working with ranges, rows and columns
- Headers and footers
- Previewing and printing formulas and worksheets
- Formulas and Functions
- Excel functions
- Relative and absolute references
- Paste options
- Insert function dialog box
- Formatting Worksheets and Cells
- Font types and styles
- Cells and cell content
- Cell borders and backgrounds
- Rows and columns
- Background images and sheet tabs
- The printed worksheet
- Working with Charts and Graphics
- Creating charts
- Embedded charts
- Chart styles and types
- Modifying a chart
- Formatting chart elements
- Adding sparklines
- Working with Excel Lists
- Introduction to lists
- Planning and creating a list
- Creating a list range
- Sorting data
- Maintaining a list
- Auto filters and custom filters
- PivotTables, PivotChart, Slicer
- Managing Multiple Worksheets and Workbooks
- Grouping worksheets
- Referencing cells and ranges
- Data consolidation
- Using templates
- Working with linked workbooks
- Using LookUp Tables
- Collaborating on a Workbook and Web Page
- Auditing formulas
- Tracing and fixing errors
- Creating a shared workbook
- Tracking changes
- Merging workbooks
- Non-interactive Web pages
- Creating and using hyperlinks
- Saving a workbook as an XML file
- Developing an Excel Application
- Validating data entry
- Protecting a worksheet and workbook
- Naming cells and ranges
- Working with macros
- Recording and running a macro
- Securing considerations for macros
- Data Tables and Scenario Management
- Cost-volume-profit analysis
- One and two-variable data tables
- Using array formulas
- Scenario manager
- Creating scenario reports
- Using Solver for Complex Problems
- Finding a solution
- Creating a Solver answer report
- Solver models
- Importing Data into Excel
- Importing text files
- Databases and queries
- Advanced Functions and Filtering
- Excel’s logical functions
- Calculating conditional counts and sums
- Advanced filtering
- Working with financial functions
Effective Term: Fall 2023 |
Corrections Academy |
|
-
COR 110 - County Correctional Officer Training Academy 18 Credits, 18 Contact Hours 18 lecture periods 0 lab periods
Training for county correctional officers. Includes ethics, human relations, law, investigations, operations, stress management, proficiency skills, reports and records, defensive tactics, inmate mental health, and juveniles.
Information: This course is open only to those sponsored by the Pima County Sheriff’s Department.
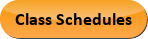
Course Learning Outcomes
- Complete all proficiency skills successfully.
- List the various Federal and State courts corrections fall under.
- List the essential elements of an effective corrections officer.
- Transport inmates between facilities without any issues.
- Write the key characteristics that should be included in a report.
- List the essential elements of an effective corrections officer.
- Demonstrate an understanding of juvenile legal mandates, psychiatric issues, and juvenile behaviors.
- Demonstrate knowledge and elements of force.
|
|
-
COR 115 - Corrections Training Officer 3 Credits, 3 Contact Hours 3 lecture periods 0 lab periods
Application of training and evaluation of recruit corrections officers. Includes operations on the first floor and front desk, in the identification and holding area, and for visitation. Also includes operations of recreation yards, general population pods, and special unit areas.
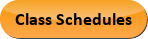
Course Learning Outcomes
- Handle a hostage situation effectively & safely.
- Transport multiple inmates according to custody levels without issue and in a professional manner between 2 different worksites.
- Demonstrate de-escalation skills with the inmate population and comprehensive knowledge of conflict resolution in the jail/prison system.
- Demonstrate a basic knowledge of how to implement life saving measures.
- Demonstrate knowledge assessing inmate mental health.
- Demonstrate knowledge of inmate recidivism and understand how to prevent their return to being in custody.
|
Culinary Arts |
|
-
CUL 101 - Principles of Restaurant Operations 3 Credits, 3 Contact Hours 3 lecture periods 0 lab periods
Fundamentals of operating and managing a restaurant, such as concept development; menu development and food purchases; kitchen equipment; and budgeting and cost control. Includes restaurant organization, job definitions and staffing, employee training, marketing, sales and promotion, customer relations and fundamentals of managing an off-premise catering service. In accordance with UNESCO certification, also includes ethical ingredients (local produce, protein, seafood, seeds, and grains); sourcing locally based on seasonality; sustainable kitchen practices; and offering menu items that complement the Southern Arizona growing region.
Prerequisite(s): CUL 105 and CUL 140 .
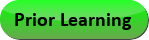.png)
Course Learning Outcomes
- Explain and apply concept development principles in restaurant operations and catering services.
- Discuss the significance of location, seating, visibility, accessibility, and design in restaurant operations.
- Demonstrate the principles of menu preparation for dine-in and catering services, including menu types, design, analysis, and layout.
- Demonstrate budget and cost control principles through practical exercises and case analysis.
- Explain the significance of employment law as it relates to recruiting, interviewing, selection, termination, and employment testing in restaurant operations.
- Describe types of kitchen equipment used in restaurant operations and discuss maintenance and sanitation of equipment.
- Prepare a simulated marketing plan with emphasis on product and service, advertising and merchandising, sales, and public relations.
- Describe considerations in food purchasing, storage, and product selection.
Outline: I. Concept Development
A. Definition
B. Life cycles
C. Utility and level of service
D. Profitability
II. Concept Location and Design
A. Seating
B. Location information check list
C. Visibility
D. Accessibility
E. Design criteria
III. Menu Development
A. Type
B. Analysis
C. Layout
D. Design
IV. Budgeting and Controlling Costs
A. Forecasting
B. Budgeting costs
C. Expense control
D. Gross profit
V. Staffing the Restaurant
A. Employment laws
B. Recruiting, interviewing, and selection
C. Termination
D. Employment testing
VI. Kitchen Equipment
A. Categories
B. Specialty cooking equipment
C. Kitchen maintenance
D. Sanitation considerations
VII. Marketing Plan, Sales, and Promotion
A. Marketing Plan
B. Sales
C. Promotion
VIII. Food Purchasing
A. Systems
B. Storage
C. Selected product purchasing
Effective Term: Full Academic Year 2019/20 |
|
-
CUL 105 - Food Service Nutrition and Sanitation 3 Credits, 3 Contact Hours 3 lecture periods 0 lab periods
Basic nutrition concepts with emphasis on the nutritional concerns of restaurants and other types of food service operations. Includes the theory of nutritional label reading; nutritional food values; and the effects food has on the body. Also includes optimal sanitation policies and procedures; maintaining a clean work environment safe from food-borne illnesses; Hazard Analysis Critical Control Points (HACCP); safety and accident prevention; storage, preparation, and cleaning of work surfaces; and legal requirements based on regulations of the local municipality.
Corequisite(s): CUL 140 Information: Consent of Culinary Arts Department is required before enrolling in this course. Students are required to pass the National ServSafe Exam prior to enrolling in additional Hospitality or Culinary classes.
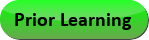.png)
Course Learning Outcomes 1. Identify and describe basic food safety concerns in a commercial food service facility.
2. Identify and describe food handling and storage techniques necessary for the prevention of food-borne illnesses.
3. Explain and apply the Hazard Analysis Critical Control Points (HACCP) concept to a commercial food service facility.
4. Describe proper cleaning and sanitizing techniques for equipment and utensils.
5. Explain the legal requirements for food service safety and sanitation, including the role of governmental agencies in maintaining safe food service facilities.
6. Select foods and plan menus that promote individual health and fitness for a variety of age groups using standard food guides and guidelines.
7. Describe the role of nutrition in promoting optimal health and fitness.
8. List the major types, functions, and food sources of carbohydrates, lipids, proteins, vitamins, minerals and water. Outline:
- Creating a Safe Food Service Environment
- Occupational safety hazards
- Food service worker personal habits
- Managerial and supervisory responsibilities
- Food-borne Illnesses
- Potential hazards
- Cross contamination
- Common causes of food borne illnesses
- Hazard Analysis Critical Control Points (HACCP): A Food Protection System
- Introduction to the HACCP system
- Hazards
- Analysis
- Critical control points
- Time and temperature charting
- Sanitation in the Purchasing, Receiving and Storage of Food
- Food suppliers
- Receiving fresh foods
- Receiving processed foods
- Storing food and supplies
- Sanitation in the Preparation and Service of Food
- Time and temperature principles
- Thawing food
- Preparing food
- Cooking food
- Cooking temperatures for meat
- Holding temperatures
- Preventing cross-contamination
- Transporting food
- Using leftover food
- Maintaining Sanitary Facilities and Equipment
- Facilities design
- Cleaning and sanitizing equipment and utensils
- Manual dishwashing
- Mechanical dishwashing
- Pest control
- Garbage and trash disposal
- Safety and Accident Prevention
- Preventing accidents
- First aid
- Fire safety
- Emergency procedures and crisis management
- Legal Requirements for Food Service Safety and Sanitation
- Regulatory agencies
- Federal
- State
- Local
- Pima County Health Department procedures and requirements
- Health and Nutrition
- Dietary factors involved in promotion of health
- Basic nutrition guidelines
- Evaluation and Use of Popular and Commercial Nutrition Information in Planning Menus
- Guidelines for evaluating contemporary nutrition information.
- Extracting information from food labels
- United States Recommended Daily Allowances (RDAs)
- Menu label requirements
- Evaluating ingredients
- Nutrient analysis
- Application of nutrition information to menu planning
- U.S. food guides and guidelines
- Tables of food composition
- Food labels
- Exchanges
- Recommended Daily Allowances (RDAs)
- Nutrition Principles and the Life Cycle
- Infants and toddlers
- Early childhood
- Adolescence
- Adults
- Seniors
Effective Term: Full Academic Year 2019/20 |
|
|
|
-
CUL 140 - Culinary Principles 3 Credits, 3 Contact Hours 3 lecture period 0 lab periods
Introduction to the hospitality and culinary arts profession. Includes professionalism; kitchen operations and culinary techniques; kitchen skills; cutting skills and proper knife use; equipment and utensil identification; use and storage of ingredients; and safety precautions. Also includes demonstrations of various cooking methods, such as dry heat cooking (roasting, grilling, sautÈing, pan frying), moist heat cooking (braising, shallow poaching, deep poaching, steaming), baking (techniques and production), and other sauces. Also includes herb and spice identification, along with scaling of a recipe, portion yields, and costing.
Corequisite(s): CUL 105 Information: Consent of Culinary Arts Department is required before enrolling in this course.
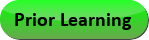.png)
Course Learning Outcomes
- Demonstrate and highlight key sanitation practices of a professional kitchen.
- Demonstrate proper beginning knife skills.
- Define cooking and food service terminology, to include both English and non-English vocabulary.
- Identify equipment and small-ware used in a professional kitchen.
- Properly identify various foods: herbs and spices, vegetables, chocolates, etc.
- Identify and apply cooking tools and equipment.
- Describe the process of sensory evaluation and conduct such an evaluation of various foods.
- Identify and describe classic stocks and sauces and their use in modern and classic food service.
Outline:
- The Culinary Profession
- History of food preparation
- History of the culinary profession
- Great chefs
- Kitchen brigade
- Hierarchy of positions
- Job Responsibilities
- Food Service Vocabulary
- Value and use of terminology
- Professional jargon
- The Menu
- Elements
- Design
- Principles of Cooking
- Heat transfer
- Cooking media
- Cooking methods
- Dry heat
- Moist heat
- Combination
- Tools and Equipment
- Hand tools
- Identification and selection
- Use and maintenance
- Heavy Equipment
- Identification and selection
- Use and maintenance
- Knives and Knife Skills
- Selecting and storing
- Sharpening and steeling
- Classic cuts
- Food Tasting
- Biological and physical components
- Cultural impact
- Sensory evaluations
- Stocks
- Stock Making
- Brown
- White
- Fish
- Vegetable
- Reductions and glaces
- Use in classic and contemporary food service
- Sauces
- Mother/Leading sauces
- Brown
- Veloute
- Bechamel
- Hollandaise
- Tomato
- Small/compound sauces
- Beurre Blanc and Beurre Rouge
- Reduction sauces
- Herbs and Spices
- History
- Tasting and sensory evaluation
- Culinary Usage
- Chocolate
- History
- Tasting and sensory evaluation
- Culinary usage
- Vegetables
- Identification
- Selection and storage
- Preparation and use
Effective Term: Full Academic Year 2019/2020 |
|
|
|
-
CUL 153 - Cakes 1 Credits, 1.5 Contact Hours .5 lecture periods 1 lab period
Introduction to the art of cake baking. Includes the ingredients, preparation, and baking of cakes. Also includes icings, decorations, and fillings.
Prerequisite(s): CUL 105 and CUL 140 .
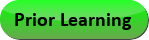.png)
Course Learning Outcomes 1. Explain the process, tools, and ingredients used to prepare cakes.
2. Identify basic types of cake ingredients, preparation tools, and mixing methods.
3. Prepare cakes using commercial baking equipment.
4. Prepare various icings, fillings, and decorations.
5. Evaluate cakes to determine quality levels.
6. Demonstrate proper production plating and presentation techniques. Outline:
- Cake Preparation
- Baking terms
- Baking ingredients and function
- Baking tools, utensils, and equipment
- Preparation principles
- Cakes
- Basic cake mixing methods
- Application of mixes and other value added products
- Cake Batter and Baking
- Panning cake batter
- Baking and cooling procedures
- Icing the Cake
- Basic types of icing, decorations, and fillings
- Icing, decorations, and filling preparation
- Evaluation of Cakes, Icings, Decorations, and Fillings
- Plating and Presentation Techniques
Effective Term: Full Academic Year 2017/18 |
|
-
CUL 156 - Pies 1 Credits, 1.5 Contact Hours .5 lecture periods 1 lab period
Introduction to the art of baking pies. Includes a variety of pastry dough, fillings, and other ingredients for creating pies and tarts. Also includes mixing; shaping; baking; and plating and presentation.
Prerequisite(s): CUL 105 and CUL 140 .
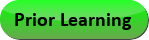.png)
Course Learning Outcomes 1. Identify basic types of pie ingredients, preparation tools, and mixing methods.
2. Prepare pies using commercial baking equipment.
3. Evaluate pies to determine quality levels.
4. Demonstrate proper production plating and presentation techniques. Outline:
- Pie Preparation
- Define baking terms
- Baking ingredients and functions
- Baking tools, utensils, and equipment
- Preparation principles
- Pie Dough
- Basic pie dough and crusts
- Mixing methods
- Pie shapes
- Application of mixes and other value added products
- Baking
- Pie filling
- Baking and cooling procedures
IV. Evaluation of Pies to Determine Quality Levels
V. Presenting Pies
- Basic types of pie toppings and appropriate uses
- Plating Techniques
Effective Term: Full Academic Year 2017/18 |
|
-
CUL 160 - Bakery and Pastry Production I 3 Credits, 5 Contact Hours 1 lecture period 4 lab periods
A comprehensive introduction to preparing an array of baked goods and sweets. Includes yeast breads; quick breads; creams and custards; cakes; filling and frostings; cookies and brownies; elementary plating; and decorating and garnishing techniques. Also includes ingredients; bakery and pastry vocabulary; and safety and sanitation.
Prerequisite(s): CUL 105 and CUL 140 . Recommendation: For students pursuing the Hospitality AAS, it is recommended that all CORE courses are completed prior to enrolling in this course.
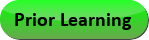.png)
Course Learning Outcomes
- Use baking and pastry terminology, including English and non-English vocabulary.
- Describe the functions of various ingredients in baked products.
- Produce a variety of bakery and pastry items to include but not limited to: yeast breads, quick breads, creams and custards, dough, cakes, fillings, icing, cookies & brownies, etc.
- Produce basic plating, decorating and garnishing techniques.
- Produce and demonstrate proper food safety and sanitation procedures required of bakery personnel.
Outline:
- Bakery and Pastry Vocabulary
- Value and use of terminology
- Professional jargon
- International lexicon
- Ingredients
- Flour
- Sugar
- Dairy and eggs
- Leavening agents
- Fats
- Flavoring agents
- Yeast Breads
- 10-stage process
- History
- Production for restaurant and catering service
- Quick Breads
- Muffin method
- Biscuit method
- Creaming method
- Production for restaurant and catering service
- Creams and Custards
- Crème Chantilly
- Crème anglaise
- Crème patisserie
- Ice cream and sorbet
- Dough’s
- Pate Sucree
- Pate Brisee
- Pate a Choux
- Dacquoise
- Cakes, Filling and Frostings
- Cake mixing methods
- High fat
- Low fat
- Fillings and frostings
- Buttercream
- Ganache
- Meringue
- Cookies and Brownies
- Bagged cookies
- Bar cookies
- Drop cookies
- Wafer cookies
- Refrigerated cookies
- Elementary Plating, Decorating and Garnishing Techniques
- Use of piping bag
- Portioning desserts and breads
- Simple plate decorations
- Production of plated desserts for restaurant and catering service
- Safety and Sanitation
- Tools and equipment
- Food storage
- Food workers’ personal hygiene
Effective Term: Fall 2019 |
|
-
CUL 162 - Art of Chocolate 3 Credits, 5 Contact Hours 1 lecture period 4 lab periods
Introduction to chocolate in the culinary arts. Includes an introduction to the properties of chocolate and the history of chocolate. Also includes the history and preparation of truffles, dough and batter; and molded and free form chocolate art work.
Prerequisite(s): CUL 105 and CUL 140
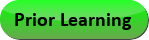.png)
Course Learning Outcomes 1. Identify types of chocolate used in the culinary arts.
2. Discuss the history of chocolate in the culinary arts.
3. Demonstrate appropriate techniques and methods used when tempering chocolate.
4. Prepare hand rolled and molded truffles of various flavors and fillings.
5. Execute recipes using batters and different types of dough with chocolate as the main ingredient.
6. Create free form and molded decorations with white, milk, and dark chocolate.
7. Perform techniques and methods used for creating and presenting chocolate artwork. Outline:
- Introduction to Chocolate
- Types of chocolate
- History of chocolate
- Tempering methods
- Ganache
- Truffles
- Hand rolled
- Molded
- Dough and Batter
- Cookie recipes
- Torte recipes
- Cake recipes
- Chocolate Art Work
- Free form decor: shapes and bows
- Chocolate plastic and marzipan: flowers and shapes
- Gelatin molds
- Boxes: molded and free form
- Molded art work
- Presentation
- Creating a plate
- Painting with cocoa butter
Effective Term: Full Academic Year 2019/20 |
|
-
CUL 163 - Sauces 3 Credits, 5 Contact Hours 1 lecture period 4 lab periods
Concepts, skills, and techniques for sauce and stock creation. Includes preparation of stocks and sauces in a traditional manner and their uses in classic and contemporary kitchens. Also includes identification of and appropriate uses for liaisons.
Prerequisite(s): CUL 105 and CUL 140 .
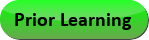.png)
Course Learning Outcomes 1. Describe four basic stocks and explain proper preparation and storage.
2. Describe five main or mother sauces and three minor sauces.
3. Discuss sauce characteristics and uses.
4. Prepare fifteen sauces using a roux, liaisons, and/or emulsions.
5. Demonstrate sanitation standards in the preparation and storage of sauces. Outline:
- Overview of Stocks
- Chicken
- Fish
- Beef and brown
- Veal
- Sauces
- Bechamel
- Roux
- Velouté
- Espagnole
- Demi-glace and secondary brown
- Tomato
- Emulsion
- Reduction
- Sauce Characteristics
- Thickening agents and compound butters
- White vs. brown
- Reduction and emulsion
- Sauce Preparation and Sanitation Standards
- Best practices
- Skills and research
- Safety and preparation
- Storage
Effective Term: Full Academic Year 2017/18 |
|
-
CUL 168 - Specialty and Hearth Breads 3 Credits, 5 Contact Hours 1 lecture period 4 lab periods
Preparation, baking, and evaluation of specialty and hearth breads. Includes the evolution of bread products, bread preparation, and the proper use of flour and yeast. Also includes preparing a variety of classic artisan bread shapes, presenting attractive finished products, and judging the quality of finished breads. Also includes health and sanitation considerations in bread making. In accordance with UNESCO certification, also includes local sourcing and sustainability of local bread making.
Prerequisite(s): CUL 105 and CUL 140 .
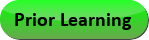.png)
Course Learning Outcomes
- Explain the process of bread preparation
- Discuss the types and use of flour and yeast
- Create a variety of classic artisan bread shapes
- Prepare an attractive bread product
- Scrutinize and judge the quality of the finished bread products
- Discuss the evolution of bread products
- Demonstrate safety and sanitation procedures in baking
Outline:
- Bread Preparation
- History of bread making
- Ingredients and processes involved in making bread
- Science of fermentation
- Science of baking
- Baker’s math
- Different types of breads
- Flour and Yeast
- Different types
- Gluten-free flours
- Dessert breads
- Quick breads
- Mixing methods
- Applications of mixes
- Starters and pre-ferments
- Preparation of pre-fermented breads
- Classic Artisan Bread Shapes
- Rye
- Pumpernickel
- German style
- Bouille
- Sticks
- Loafs
- Braids
- Rolls
- Addition of grains, fruits and specialty flours
- Rolls, breads, and decorative breads made from lean dough, sourdough, and multi-grains
- Product Appearance
- Baking procedures
- Cooling procedures
- Displaying breads
- Storing bread
- Finished Product Quality
- Taste and texture
- Bread crackle and crust
- Evaluation of product quality
- Evolution of Bread Products
- History of bread
- Evolution of products
- Yeast
- Hearth breads
- Health and Sanitation in the Kitchen
- Bacteria and cross-contamination prevention
- Storage of grains
- Washing and sanitizing equipment
- Proper storage of bread
Effective Term: Full Academic Year 2019/20 |
|
-
CUL 170 - Dining Room Operations 2 Credits, 2 Contact Hours 2 lecture periods 0 lab periods
Theory and practice of operating a casual dining room. Includes preparation for proper dining and service etiquette for staff. Also includes proper techniques for clearing tables, service of wine, beverage sales and techniques, salesmanship, and customer service.
Prerequisite(s): CUL 105 and CUL 140 .
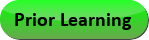.png)
Course Learning Outcomes 1. Describe the basic elements of dining room service and operations, including dining room preparation, taking guest orders, presenting checks, and other tasks.
2. Display proper etiquette for serving and clearing tables.
3. Perform wine and beverage service using proper etiquette.
4. Demonstrate effective sales techniques.
5. Describe appropriate customer relations techniques. Outline:
- Dining Room Preparation and Guest Service
- Table service
- Table set-up
- Table maintenance during meal
- Table clearing
- Opening and closing side work
- Arranging tables per reservations
- Stocking side stations
- Refilling, cleaning and stocking condiments
- Cleaning
- General rules of etiquette
- Taking guests’ orders
- Numbering systems
- Writing guest checks
- Point-of-sale systems
- Presenting guest checks for payment
- Proper Etiquette for Service and Clearing
- Set-up of china, glassware and silver
- Service procedures
- Clearing procedures
- Wine and Beverage Sales and Service
- Wine and beer
- Coffee, tea and other beverages
- Presentation and service of beverages
- Glassware selection and storage
- Salesmanship
- Upselling the product
- Understanding guests’ requirements
- Increasing profits
- Serving the Public
- Guest satisfaction
- Guest dissatisfaction
- Unruly guests
- Procedures for guest compensation
- Guest intoxication
- Legal responsibilities
- Methods of prevention
Effective Term: Fall 2023 |
|
-
CUL 174 - From Garden to Table 3 Credits, 5 Contact Hours 1 lecture period 4 lab periods
Introduction to preparing edible plants grown in the Sonoran Desert. Includes the best vegetable and herb selection for year-round harvest, as well as an investigation of optimal soil composition, composting, planting and harvesting techniques, seed saving, and preserving and storage methods. Also includes the nutritional advantage of locally grown plants and how to prepare them for optimal nutritional value. In accordance with UNESCO certification, also includes a survey of best practices for sustainability and recycling in the food service industry.
Prerequisite(s): CUL 105 and CUL 140 .
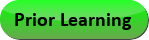.png)
Course Learning Outcomes 1. Describe the planting zone and planting seasons of the Sonoran Desert.
2. Determine appropriate selection criteria for the Sonoran Desert planting zone and list appropriate vegetables for desert seasons.
3. Identify the proper location for a garden and compost site depending on plants and herbs chosen for planting.
4. Describe how to test soil quality and add nutrients to soil that will produce flavorsome and healthy plants.
5. Determine the most appropriate system for providing water to a garden.
6. Identify plants that will attract specific pollinators and why these are important.
7. Describe the pest control methods that are environmentally friendly and maintain plant nutrition.
8. Prepare various types of desert vegetables and herbs in a nutritious and healthy manner.
9. Research and describe campus and kitchen practices to determine if they are observing best practices for creating a sustainable environment and doing all they can to enhance recycling. Outline:
- Plants in Desert Conditions
- Plants that grow and thrive in a desert environment
- Edible plants that grow in the Sonoran Desert
- Edible plants that grow in various seasons in the Sonoran Desert
- Choosing what and when to plant
- Plant Basics
- Proper locations for planting edible herbs and plants
- Soil requirements
- Identification of soil content
- Preparing the proper planting medium
- Contribution of composting to healthy, nutritious plants
- Water
- Hand watering v. irrigation
- Water harvesting from rain
- Pest Control
- Identification of garden pests
- Controlling garden pests in an environmentally friendly way that maintains a healthy garden and nutritious plants
- Natural Pollinators
- Plants that attract specific pollinators
- Importance of attracting pollinators to vegetable gardens
- Nutrition
- Nutritional benefits of desert gardening practices
- Nutritional benefits of specific plants and herbs grown
- Preparation of harvested vegetables and herbs in a nutritious and healthy manner
Effective Term: Full Academic Year 2019/20 |
|
-
CUL 180 - Food in History 3 Credits, 3 Contact Hours 3 lecture periods 0 lab periods
History of food, the story of cuisine, and the social history of eating. Includes collecting, gathering and hunting food; stock-breeding and farming; sacramental foods; the economy of food markets; the era of merchants; New World food discoveries; seed migration; and professional food preparation. Also includes local indigenous foods of the people who resided in Southern Arizona; Native cultivation and methods of desert foraging; and Spanish (Father Kino) and Mexican/Chinese influences.
Prerequisite(s): CUL 105 and CUL 140 .
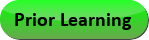.png)
Course Learning Outcomes 1. Trace the historical background of various foods.
2. Describe the role of collecting, gathering and hunting food in the development of humans.
3. Describe the development of farming and stockbreeding.
4. Describe the role of sacramental foods in various cultures.
5. Discuss the economy of food markets in history.
6. Explain the role of essential and luxury foods in merchant-based economies.
7. Trace the spread of indigenous New World foods throughout the world and describe the impact of these foods on various cuisines.
8. Trace the historical development of professional food preparation. Outline:
- Collecting, Gathering and Hunting Food
- Collecting
- Honey
- Berries and other plant-based foods
- Gathering
- Hunting
- Stock-breeding and Farming
- History of meat
- History of dairy
- History of grains and cereals
- Sacramental Foods
- Oil
- Bread
- Wine
- The Economy of Food Markets
- History of fishing
- History of poultry
- The Era of Merchants
- Essential foods
- Luxury foods
- Chocolate
- Pepper
- Spices
- Sugar
- Social Influences on Food Availability
- Coffee and tea
- Tomatoes and potatoes
- New World
- Columbus, Cortez and New World Food Discoveries
- Indigenous foods
- The lasting impact on world cuisines
- Famines
- Flavor influences
- Professional Food Preparation
- Guild systems
- Restaurants and royalty
- Chefs
Effective Term: Full Academic Year 2019/20 |
|
-
CUL 185 - Catering Operations 3 Credits, 5 Contact Hours 1 lecture period 4 lab periods
Theory and practice of planning and executing catering functions. Includes booking and planning, banquet room set-up and staffing, banquet service, guest payment and follow up, and specialized functions.
Prerequisite(s): CUL 105 and CUL 140 .
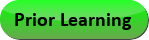.png)
Course Learning Outcomes 1. Identify and describe the basic elements of a catering function.
2. Explain the booking and planning process of a catering function.
3. Describe the appropriate methods of scheduling space, set-up and staffing of a banquet room along with the proper methods of etiquette for banquet food & beverage service.
4. Describe methods of customer contact and follow up, including menu planning and incidental arrangements and procedures for payment.
5. Identify and describe particular needs of special banquet functions.
6. Execute various catering functions. Outline:
- Catering: Booking and Planning
- Evaluation
- Number of guests and space requirements
- Time requirements
- Type of meal and service
- Incidental requirements and arrangements
- Booking Space/Function Arrangements
- Arranging space and room set-up
- Ordering incidentals
- Scheduling staff
- Banquet Room Set-up and Staffing
- Meeting arrangements
- Dining arrangements
- Customer Contact
- Menu Planning
- Incidental Arrangements
- Banquet Service
- Beverage service/cocktail parties
- Food service
- Preparation and set-up
- Guest service
- Clearing and cleaning up
- Guest Payment and Follow Up
- Methods of Payment
- Deposits and partial payments
- Billing arrangements
- Follow up
- Thank you letters
- Evaluations
- File systems for future
- Special Functions
- Weddings
- Requirements
- Service and servers
- Follow-up
- Buffets
- Requirements
- Theme parties
- Service and servers
- Follow up
- Outside Catering Functions
- Requirements/limitations
- Service and servers
- Follow-up
Effective Term: Full Academic Year 2019/2020 |
|
-
CUL 189 - Culinary Arts Capstone I 1 Credits, 1.5 Contact Hours .5 lecture periods 1 lab period
Preparation of a final culinary project that meets the learning outcomes required in the specific cooking/lab and lecture courses. Also includes review of culinary principles and demonstration of sanitation skills and safety practices.
Prerequisite(s): CUL 105 and CUL 140 or concurrent enrollment. Information: Course activities may take place in a simulated work setting. This is the capstone experience.
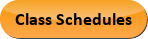
Course Learning Outcomes
- Demonstrate safety and sanitation skills in the handling, preparing, cooking, and clean-up of food learned in a combination of all previous hospitality courses.
- Exhibit professionalism in appearance, team work, and work proficiency learned in a combination of all previous hospitality courses.
- Execute a casual lunch experience using a combination of skills learned through the program to include: different cooking techniques, knife skills, cooking vocabulary, characteristics and use of specific foods, use of tools and equipment, costing, scaling, and following a recipe, preparing food products from the hot foods, cold foods, baked products and sauces categories that meets advanced commercial standards in appearance and taste
Outline: Students work on a culinary assignment that is appropriate to their program of study and their readiness to enter the workforce. They will incorporate knowledge and skills acquired in the Culinary Fundamentals or Culinary Arts Advanced Certificate program into a culinary project that will take place in the culinary lab or a simulated working kitchen. Skills and knowledge to be demonstrated may include:
- Basic Knife Cuts
- Brunoise
- Dice
- Julienne
- Jardiniere
- Roll Cut
- Chiffonade
- Bias
- Creating a Safe Food Service Environment:
- Occupational safety hazards
- Food-borne Illnesses
- Potential hazards
- Cross contamination
- Food service worker personal habits
- Managerial and supervisory responsibilities
- Hazard Analysis Critical Control Points (HACCP): A Food Protection System
- Introduction to the HACCP system
- Hazards
- Analysis
- Critical control points
- Time and temperature charting
- Sanitation in the Purchasing, Receiving and Storage of Food
- Food suppliers
- Receiving fresh foods
- Receiving processed foods
- Storing food and supplies
- Sanitation in the Preparation and Service of Food
- Time and temperature principles
- Thawing food
- Preparing food
- Cooking food
- Cooking temperatures for meat
- Holding temperatures
- Preventing cross-contamination
- Transporting food
- Using leftover food
- The Menu
- Elements
- Design
- Principles of Cooking
- Heat transfer
- Cooking media
- Cooking methods
- Dry heat
- Moist heat
- Combination
- Tools and Equipment
- Hand tools
- Identification and selection
- Use and maintenance
- Heavy Equipment
- Identification and selection
- Use and maintenance
- Knives and Knife Skills
- Selecting and storing
- Sharpening and steeling
- Classic cuts
- Cooking Techniques:
- Sauté/sweat
- Braise
- Grill
- Broil
- Roast
- Poach/simmer
- Fabrication:
- Beef
- Poultry
- Shellfish
- Fish
- Pork
- Lamb
- Egg Cookery
- Boil
- Poach
- Fry
- Omelets
- Dressings: Emulsified and Non-Emulsified
- Vinegars
- Oils
- Binders
- Mustards
- Mayonnaise
- Basic Sandwiches
- Types
- Preparation
- Presentation techniques
- XV. Herbs & Spices
- Herbs:
- Basil
- Cilantro
- Thyme
- Rosemary
- Mint
- Oregano
- Spices
- Nutmeg
- Pepper
- Cinnamon
- Allspice
- Cloves
- Ginger
- Salad Greens
- Washing and storage Types
- Romaine
- Bibb
- Endive
- Frisee
- Oakleaf
- Serving techniques
- Bakery and Pastry Vocabulary
- Professional jargon
- International lexicon
- Ingredients
- Flour
- Sugar
- Dairy and eggs
- Leavening agents
- Fats
- Flavoring agents
- Yeast Breads
- 10-stage process
- History
- Production for restaurant and catering service
- Quick Breads
- Muffin method
- Biscuit method
- Creaming method
- Production for restaurant and catering service
- Creams and Custards
- Creme chantilly
- Creme anglaise
- Creme patisserie
- Ice cream and sorbet
- Doughs
- Pate Sucree
- Pate Brisee
- Pate a Choux
- Dacquoise
- Cakes, Filling and Frostings
- Cake mixing methods
- High fat
- Low fat
- Fillings and frostings
- Buttercream
- Ganache
- Meringue
- Cookies and Brownies
- Bagged cookies
- Bar cookies
- Drop cookies
- Wafer cookies
- Refrigerated cookies
- Sauces
- Mother Sauces
- Espagnole
- Veloute
- Hollandaise
- Tomato
- Bechamel
- Liaison
- Thickening Agents:
- Roux
- White
- Brown
- Starches:
- Potato
- Rice
- Corn
- Arrowroot
- Flour
- Restaurant Operations
- Concept Development
- Location and Design
- Menus
- Budgeting
- Staffing
- Kitchen Equipment
- Marketing, Sales, Promotion
- Food Purchasing
- Garden to Table
- Plants in Desert Conditions
- Plant Basics
- Water
- Pest Control
- Natural Pollinators
- Nutrition
Effective Term: Full Academic Year 2019/20 |
|
-
CUL 244 - Confections, Show Pieces, & Plated Desserts 3 Credits, 5 Contact Hours 1 lecture period 4 lab periods
Concepts, skills, and techniques used to create chocolate and sugar decorations that embellish other desserts or function as artistic showpieces for display. Includes techniques such as applying chocolate colors with a spray gun, use of various types of molds, and making cut-out decorations and silk screens that will be applied to showpieces. Also includes an introduction to sugar techniques such as pastillage, saturated sugar, pulled sugar (e.g., ribbons and flowers), blown sugar (spun, piped, bubble, straw) to create three-dimensional shapes, and poured sugar to create showpieces.
Prerequisite(s): CUL 160
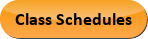
Course Learning Outcomes
- Demonstrate the following various processes for, but not limited to: tempering process for chocolate, Ganache fillings, molded and hand molded confections, aerated confections, jellies, and Crystalline sugar confections.
- Demonstrate the fundamental principles of chocolate showpieces, plate presentation for desserts, show cakes (how to make and present specialty cakes that are used for show pieces).
Outline:
- Tempering Methods: Seeding and Tabling
- Dark chocolate
- Milk chocolate
- White chocolate
- Ganache Fillings for Confections
- Cream
- Butter
- Egg
- Hand Molded and Molded Confections
- Use of plastic molds when making truffles
- Technique used for hand rolled truffles
- Non-Chocolate Confections:
- Aerated fudges/chews/marshmallow
- Jellies - gummies
- Crystalline confections - caramels and toffee
- Presentation
- Chocolate showpiece works
- Plated desserts
- Show cakes/specialty cakes
Effective Term: Full Academic Year 2019/20 |
|
-
CUL 251 - International Cuisine: World of Flavor 3 Credits, 5 Contact Hours 1 lecture period 4 lab periods
Concepts, skills, and techniques used to create global cuisine. Includes ingredients and foods from around the world. Also includes culinary techniques that incorporate culture and food traditions from Latin America, the Mediterranean, Europe, Asia, and the United States.
Prerequisite(s): CUL 130 , CUL 150 , and CUL 160 .
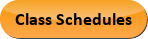
Course Learning Outcomes
- Differentiate the specific flavor profiles, essential ingredients and basic techniques used in each culture.
- Identify and handle spices, spice paste and rubs unique to each cuisine.
- Create little dishes with bold flavors known as chutneys, salsa and sambals to enhance the flavor of existing menu items.
- Explain how to build flavor profiles unique to each country in meat, poultry, seafood, vegetables and fruits.
- Demonstrate the techniques of each country studied to create an authentic flavor.
Outline: I. Central America, Latin America, and Mesoamerica
A. Geographic overview
B. History and culture
C. Pre-Columbian Food
D. Food after the Spanish and Portuguese conquest
E. Meat, soups, sauces, fish, and poultry
F. Chiles, empanadas, and sweets
II. Mediterranean and Europe
A. Geographic overview
B. Regions
C. Agriculture
1. Regional foods of France and Italy
2. Foods of the Rivera from France to Italy
3. Foods of Britain, Germany, and Netherlands
4. Foreign influences
III. Asian Cuisine
A. Geographic overview
B. History and culture
C. Interaction with the West
1. Vegetables and fruits
2. Sauces and flavors from fermented fish to vinegar
3. Rice and grains
4. Soy to soup
IV. India and its Neighbors
A. Geographic overview
B. History and culture of India, Nepal, Bhutan, Afghanistan, and Pakistan
C. Integral tastes from curry to mango and beyond
V. United States Regional Foods
A. Evolving demographics
B. Changing food scene
C. European, Asian, African and Latin immigrants and related foods
D. Changing food scenes in America from organics to grass fed beef
1. Foods of the North, Northeast and central states
2. Foods of the South and Southwest
3. Foods of the West and the Pacific Northwest
4. Soul food
Effective Term: Full Academic Year 2019/20 |
|
-
CUL 256 - Special Diets 3 Credits, 5 Contact Hours 1 lecture period 4 lab periods
Skills and techniques needed to plan and prepare special diets while providing culinary inspiration for healthy, wholesome meals. Includes a wide range of dietary challenges chefs must consider, such as nutrition, taste, and healthy ingredients while preparing gluten free, vegetarian, and vegan meals. Also includes substitutions as alternatives to prohibited ingredients.
Prerequisite(s): CUL 105 , CUL 140 , CUL 130 Recommendation: For students pursuing the Hospitality AAS, it is recommended that all CORE courses are completed prior to enrolling in this course.
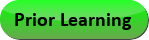.png)
Course Learning Outcomes 1. Prepare Gluten-Free, Vegan, Ovo, Lacto-Ovo, Pescan Vegetarian, and Lacto Vegetarian menu items containing dairy along with plant based products.
2. Describe the adaptation of regular dietary practices to a vegan and gluten-free diet practice.
3. Demonstrate conversion of high gluten foods, such as bread, pasta, and cookies, into appetizing gluten free versions. Outline:
- Vegetarians: Who They Are and What They Eat
- Effects of removing animal based proteins from the diet
- Practice of a vegetarian diet
- Lacto vegetarianism
- Pesco vegetarianism
- Ovo vegetarian diet
- Preparing Lacto Vegetarian, Ovo Vegetarian, and Lacto-Ovo Vegetarian Meals
- Cooking terms
- Recipes, ingredients and function
- Preparation tools, utensils and equipment
- Food preparation
- Pesco Vegetarianism
- Terms of pesco vegetarian cooking
- Effects of adding seafood to the diet
- Preparation tools, utensils and equipment
- Food preparation
- Vegans: Who They Are and What They Eat
- Defining the terms of a vegan lifestyle
- Practices of a vegan diet
- Essential ingredients required in our diet
- Essential grains for the vegan diet
- Nuts, seeds and soy
- How to Prepare a Vegan Meal
- Defining cooking terms
- Recipe ingredients and their functions
- Preparation tools, utensils and equipment
- Food preparation
- Preparing a Vegan Menu with the Broadest Appeal
- Menu Items
- Recipe ingredients and function
- Preparation tools, utensils and equipment
- Preparing the menu
- Gluten
- Character and role of gluten in food
- Effect of removing gluten from food.
- Practice of a gluten free diet
- Preparing Familiar Food as Gluten Free
- Defining cooking terms
- Recipe ingredients and function
- Preparation tools, utensils, and equipment
- Food preparation
- Preparing New Gluten Free Recipes
- Defining cooking terms
- Recipe ingredients and function
- Preparation tools, utensils, and equipment
- Food Preparation
- Preparing High Gluten Foods as Gluten Free
- Define baking terms
- Baking ingredients and function
- Baking tools, utensils, and equipment
- Preparation principles
Effective Term: Full Academic Year 2019/2020 |
|
-
CUL 260 - Pastry Arts II 3 Credits, 5 Contact Hours 1 lecture period 4 lab periods
Advanced theory and practice of operating a bakery or pastry shop in a hotel or restaurant kitchen. Includes planning, ordering, and scheduling for bakery production; safety and sanitation; and bakery and pastry vocabulary. Also includes advanced yeast breads; classic French pastries; ice cream and frozen desserts; pastry assembly; pastry garnishes; and complex plated desserts.
Prerequisite(s): CUL 160 Corequisite(s): CUL 251
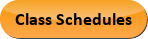
Course Learning Outcomes
- Describe and execute the planning, ordering, and scheduling required in a commercial bakeshop.
- Identify, describe, and implement proper food safety and sanitation procedures required of bakery personnel in the operation of a commercial bakeshop.
- Define and use baking and pastry terminology, including English and non-English vocabulary.
- Identify, describe, and produce a variety of advanced yeast breads.
- Identify, describe, and produce a variety of classic French pastries.
- Identify, describe, and produce a variety of ice creams and frozen desserts.
- Assemble a variety of pastries from prepared dough, creams, custards and cakes.
- Identify, describe and produce a variety of advanced pastry garnishes.
- Produce complex plated desserts for restaurant and banquet service.
Outline:
- Planning, Ordering, and Scheduling for Bakeshop Production
- Quantity
- Timeliness
- Storage
- Personnel
- Safety and Sanitation
- Tools and equipment
- Food storage
- Food workers’ personal hygiene
- Bakery and Pastry Vocabulary
- Value and use of terminology
- Professional jargon
- International lexicon
- Advanced Yeast Breads
- Danish pastry
- Croissants
- Brioche
- Sourdough
- Classic French Pastries
- Napoleons
- St. Honore
- Paris-Breast
- Soufflés
- Charlottes
- Petit Fours
- Ice Cream and Frozen Desserts
- Ice cream
- Sorbet
- Sherbet
- Semifreddo
- Assembling Pastries
- Use of prepared dough, creams, custards, and cakes
- Selecting pastry components
- Color
- Flavor
- Texture
- Availability
- Assembly skills
- Pastry Garnishes
- Advanced piping techniques
- Tempered chocolate decorations
- Poured and spun sugar
- Complex Plated Desserts
- Dessert sauces
- Preparation
- Use in decoration
- Chocolate garnishes
- Sugar garnishes
Effective Term: Full Academic Year 2019/20 |
|
-
CUL 266 - Ice Creams/Bavarians/Mousse/Sauces 3 Credits, 5 Contact Hours 1 lecture period 4 lab periods
Professional dessert presentations using both classical and modern techniques of mousse, Bavarians, ice creams, sorbets and sauces. Includes the theory and applications necessary to prepare light desserts: the science and effects of egg coagulation, ice crystallization, and gelatin on liquids and fats in a hands-on situation. Also includes current application of fruit cookery, dessert sauces and tableside desserts.
Prerequisite(s): CUL 160
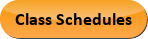
Course Learning Outcomes 1. Demonstrate the fundamental principles of producing frozen stirred custards and ice creams, gelatin, Italian/ common meringue and Paté a Bomb, raw and cooked fruit sauces, caramel and chocolate sauces, chocolate and fruit mousses Outline:
- Frozen aerated dessert production:
- Ice Cream
- Gelato
- Sorbet
- Granita
- Working with Gelatin
- Powdered and sheet gelatin
- Understanding the blooming process of gelatin
- Understanding on how to incorporate gelatin into dessert items
- Meringue
- Common Meringue
- Italian Meringue
- Pate a Bomb
- How it is incorporated into a Mousse and Bavarian
- Sauces
- Fruit - cooked and uncooked
- Caramel
- Chocolate
- Mousses
- Fruit
- Chocolate
- Caramel
Effective Term: Full Academic Year 2019/2020 |
|
-
CUL 276 - Pastry Production 3 Credits, 5 Contact Hours 1 lecture period 4 lab periods
Techniques and principles of skill development, production planning, and pace of production in the bakeshop. Includes preparation of a variety of cookies, sponge and specialty cakes, and breads.
Prerequisite(s): CUL 160
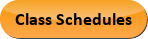
Course Learning Outcomes
- Demonstrate the fundamental principles of high volume baking, producing the following items (but not limited to): Quick Breads, Laminated Dough, Yeast Dough
- Describe the classification of Cookie Dough
- Demonstrate High Ratio Cakes using Whipped Egg/Separated Egg Foam
- Produce various Buttercreams - Italian, French and Swiss
Outline:
- Mass Production of Baked Goods
- Recipe Conversion Factor
- Understanding the science of high volume baking
- Quick Breads
- Muffins
- Pound Cakes
- Scones and Biscuits
- Laminated Dough
- Danish
- Croissant
- Puff Pastry
- Yeast Dough
- Lean- crusty breads
- Enriched- soft breads
- Slack- flat breads
- Cookie Dough
- Learning the classifications of cookie dough’s.
- Cakes
- High Ratio
- Whipped Egg
- Separated Egg Foam
- Buttercreams
- Italian
- Swiss
- French
Effective Term: Full Academic Year 2019/2020 |
|
-
CUL 289 - Culinary Arts Capstone II 2 Credits, 2 Contact Hours .5 lecture periods 1.5 lab period
The capstone experience for Culinary Arts and Baking & Pastry. Includes preparation of a final advanced-level culinary project that meets the learning outcomes required in specific cooking/lab and lecture courses. Also includes a review of culinary principles, the demonstration of sanitation skills and safety practices, and the display of an advanced level of professionalism and proficiency in kitchen operations and food preparation, as well as restaurant operations.
Information: Course activities will take place in a simulated work setting for an entire 16-week semester. You must complete all requirements in your pathway to be eligible to enroll in this course. See the program advisor for more information.
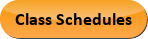
Course Learning Outcomes 1. Demonstrate safety and sanitation skills in the handling, preparing, cooking, and clean-up of food learned in a combination of all previous hospitality courses.
2. Exhibit professionalism in appearance, team work, and work proficiency learned in a combination of all previous hospitality courses.
3. Execute a formal dinner experience using a combination of skills learned throughout the program to include: different cooking techniques, knife skills, cooking vocabulary, characteristics and use of specific foods, use of tools and equipment, costing, scaling, and following a recipe, preparing food products from the hot foods, cold foods, baked products and sauces categories that meets advanced commercial standards in appearance and taste. Outline: Students work on a culinary assignment that is appropriate to their program of study and their readiness to enter the workforce. They will incorporate knowledge and skills acquired in the AAS Degree program into a culinary project that will take place in the culinary lab or a simulated working kitchen. Skills and knowledge to be demonstrated may include:
- Basic Knife Cuts
- Brunoise
- Dice
- Julienne
- Batonnet
- Roll Cut
- Chiffonade
- Bias
- Creating a Safe Food Service Environment:
- Occupational safety hazards
- Food-borne Illnesses
- Potential hazards
- Cross contamination
- Food service worker personal habits
- Managerial and supervisory responsibilities
- Hazard Analysis Critical Control Points (HACCP): A Food Protection System
- Introduction to the HACCP system
- Hazards
- Analysis
- Critical control points
- Time and temperature charting
- Sanitation in the Purchasing, Receiving and Storage of Food
- Food suppliers
- Receiving fresh foods
- Receiving processed foods
- Storing food and supplies
- Sanitation in the Preparation and Service of Food
- Time and temperature principles
- Thawing food
- Preparing food
- Cooking food
- Cooking temperatures for meat
- Holding temperatures
- Preventing cross-contamination
- Transporting food
- Using leftover food
- The Menu
- Elements
- Design
- Principles of Cooking
- Heat transfer
- Cooking media
- Cooking methods
- Dry heat
- Moist heat
- Combination
- Tools and Equipment
- Hand tools
- Identification and selection
- Use and maintenance
- Heavy Equipment
- Identification and selection
- Use and maintenance
- Knives and Knife Skills
- Selecting and storing
- Sharpening and Straightening Blade with “Steel”
- Classic cuts
- Cooking Techniques:
- Sauté/sweat
- Braise
- Grill
- Broil
- Roast
- Poach/simmer
- Deep Fat Fry
- Fabrication:
- Beef
- Poultry
- Shellfish
- Fish
- Pork
- Lamb
- Egg Cookery
- Boil
- Poach
- Fry
- Omelets
- Dressings: Emulsified and Non-Emulsified
- Vinegars
- Oils
- Binders
- Mustards
- Mayonnaise
- Basic Sandwiches
- Types
- Preparation
- Presentation techniques
- Herbs & Spices
- Herbs:
- Basil
- Cilantro
- Thyme
- Rosemary
- Mint
- Oregano
- Spices
- Nutmeg
- Pepper
- Cinnamon
- Allspice
- Cloves
- Ginger
- Salad Greens
- Washing and storage Types
- Romaine
- Bibb
- Endive
- Frisee
- Oakleaf
- Serving techniques
- Bakery and Pastry Vocabulary
- Professional jargon
- International lexicon
- Ingredients
- Flour
- Sugar
- Dairy and eggs
- Leavening agents
- Fats
- Flavoring agents
- Yeast Breads
- 10-stage process
- History
- Production for restaurant and catering service
- Quick Breads
- Muffin method
- Biscuit method
- Creaming method
- Production for restaurant and catering service
- Creams and Custards
- Crème Chantilly
- Crème anglaise
- Crème patisserie
- Ice cream and sorbet
- Dough’s
- Pate Sucree
- Pate Brisee
- Pate a Choux
- Dacquoise
- Cakes, Filling and Frostings
- Cake mixing methods
- High fat
- Low fat
- Fillings and frostings
- Buttercream
- Ganache
- Meringue
- Cookies and Brownies
- Bagged cookies
- Bar cookies
- Drop cookies
- Wafer cookies
- Refrigerated cookies
- Sauces
- Mother Sauces
- Espagnole
- Veloute
- Hollandaise
- Tomato
- Bechamel
- Liaison
- Thickening Agents:
- Roux
- White
- Brown
- Starches:
- Potato
- Rice
- Corn
- Arrowroot
- Flour
- Restaurant Operations
- Concept Development
- Location and Design
- Menus
- Budgeting
- Staffing
- Kitchen Equipment
- Marketing, Sales, Promotion
- Food Purchasing
- Garden to Table
- Plants in Desert Conditions
- Plant Basics
- Water
- Pest Control
- Natural Pollinators
- Nutrition
- Special Diets
- Gluten-free
- Vegetarian
- Vegan
- International Cuisine
Effective Term: Fall 2023 |
Dance |
|
-
DNC 105 - Ballet Folklorico I 2 Credits, 3 Contact Hours 1 lecture period 2 lab periods
Study of diverse regional dances and music of Mexico. Includes Mexican folkloric dance terminology, history and music from a variety of understanding of the similarities and differences between each region will be explored. Includes the influences of the regional environment and the artistic interpretation of its people. Also includes an introduction to dance vocabulary, choreography techniques, dance history, the anatomy of a dancer, and injury prevention.
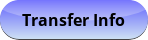.png) 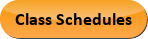
Course Learning Outcomes
- Learn basic vocabulary of Mexican Folklorico Dance
- Demonstrate correct placement and alignment for folklorico at the beginning level.
- Apply basic folklorico techniques, theories, and styles at the beginning level.
- Perform basic locomotor and axial movements in the center and across the floor at the beginning level.
- Practice the basic elements of dance: space, time, and energy at the beginning level.
- Perform folklorico movement in an expressive manner at the beginning level.
- Integrate Mexican Folklorico Dance with physical fitness
Outline:
- Class Protocol
- Concepts
- Warm-up
- Floor work
- Standing and center floor work
- Locomotor work
- Combination
- Cool down
- Class Decorum
- Warm-Up
- Placement and centering
- Anatomy and physiology of a proper warm-up
- Floor Work
- Flexibility techniques
- Various types of stretches
- Theories of stretching
- Floor positions
- Floor Isolations
- Upper body designs
- Strength concepts
- Standing and Center Floor Work
- Placement and centering
- Positions of the feet
- Positions of the arms
- Torso work
- Leg and footwork
- Axial work
- Isolations
- Turns
- Intermediate Locomotor Work
- Movements
- Combinations
- Traveling movements
- Aerial (jumping, leaping) movements
- Elements
- Energy
- Space
- Time
- Developing the Craft
- Dynamics
- Stage directions
- Basic performance skills
- Focus
- Style
Effective Term: Fall 2023 |
|
-
DNC 150 - Ballet I 2 Credits, 3 Contact Hours 1 lecture period 2 lab periods
Introduction to the theory and practice of ballet at the beginning level. Includes terminology, barre, floor work, standing and center floor work, locomotor work, elements, and developing the craft.
Information: May be taken two times for a maximum of four credit hours. If this course is repeated, see a financial aid or Veteran’s Affairs advisor to determine funding eligibility as appropriate.
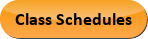
Course Learning Outcomes
- Discuss the terminology of ballet in French terms.
- Demonstrate correct placement and alignment for ballet at the beginning level.
- Use basic locomotor and axial movements at the barre, in center floor, and across the floor at the beginning level.
- Identify correct components of ballet technique, theories, and styles at the beginning level.
- Utilize the theory of the body’s relationship to gravity and the center of gravity used in ballet at the beginning level.
- Practice the basic elements of dance: space, time, and energy at the beginning level.
- Apply technical skills, theories, and style in performance of ballet at the beginning level.
Outline:
- Ballet (Dance) Terminology
- Barre
- Basic placement and centering
- Positions of the feet
- Positions of the arms
- Positions of the torso
- Foot and leg articulation
- Balance
- Stretching
- Floor Work
- Flexibility techniques
- Various types of stretches
- Theories of stretching
- Basic floor positions
- Strength concepts
- Standing and Center Floor Work
- Basic placement and centering
- Positions of the feet
- Positions of the arms
- Positions of the torso work
- Leg and foot articulation
- Axial work
- Adagio – slow work
- Turns
- Locomotor Work
- Allegro – fast work
- Beginning combinations
- Space awareness
- Time awareness
- Energy awareness
- Beginning traveling movements
- Beginning aerial (jumping, leaping) movements
- Elements
- Energy
- Space
- Time
- Developing the Craft
- Dynamics
- Stage directions
- Basic performance skills
- Focus
- Style
Effective Term: Spring 2017 |
|
-
DNC 151 - Ballet II 2 Credits, 3 Contact Hours 1 lecture period 2 lab periods
Continuation of DNC 150 . Includes ballet techniques at the intermediate level, terminology, barre, floor work, standing and center floor work, locomotor work, elements, and developing the craft.
Prerequisite(s): DNC 150 Information: May be taken two times for a maximum of four credit hours. If this course is repeated, see a financial aid or Veteran’s Affairs advisor to determine funding eligibility as appropriate.
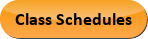
Course Learning Outcomes
- Continue learning the terminology of ballet in the French terms.
- Demonstrate correct placement and alignment for ballet at the intermediate level.
- Use basic locomotor and axial movements at the barre, in center floor, and across the floor at the intermediate level.
- Identify correct components of ballet technique, theories, and styles at the intermediate level.
- Utilize the theory of the body’s relationship to gravity and the center of gravity used in ballet at the intermediate level.
- Practice the basic elements of dance: space, time, and energy at the intermediate level.
- Apply technical skills, theories, and style in performance of ballet at the intermediate level.
Outline:
- Ballet (Dance) Terminology
- Barre
- Placement and centering
- Positions of the feet
- Positions of the arms
- Positions of the torso
- Foot and leg articulation
- Balance
- Stretching
- Floor Work
- Flexibility techniques
- Various types of stretches
- Theories of stretching
- Floor positions
- Strength concepts
- Standing and Center Floor Work
- Placement and centering
- Positions of the feet
- Positions of the arms
- Positions of the torso work
- Leg and foot articulation
- Axial work
- Adagio – slow work
- Turns
- Locomotor Work
- Allegro – fast work
- Intermediate combinations
- Space awareness
- Time awareness
- Energy awareness
- Intermediate traveling movements
- Intermediate aerial (jumping, leaping) movements
- Elements
- Energy
- Time
- Space
- Developing the Craft
- Dynamics
- Stage directions
- Basic performance skills
- Focus
- Style
Effective Term: Spring 2017 |
|
-
DNC 152 - Ballet III 2 Credits, 3 Contact Hours 1 lecture period 2 lab periods
Continuation of DNC 151 . Includes ballet technique at the advanced level, ballet terminology, barre, floor work, standing and center floor work, locomotor work, elements, and developing the craft.
Prerequisite(s): DNC 151 Information: May be taken two times for a maximum of four credit hours. If this course is repeated, see a financial aid or Veteran’s Affairs advisor to determine funding eligibility as appropriate.
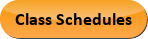
Course Learning Outcomes
- Continue learning the terminology of ballet in the French terms.
- Demonstrate correct placement and alignment for ballet at a more advanced level.
- Use basic locomotor and axial movements at the barre, in center floor, and across the floor.
- Identify correct components of ballet technique, theories, and styles.
- Utilize the theory of the body’s relationship to gravity and the center of gravity used in ballet.
- Practice the basic elements of dance: space, time, and energy.
- Apply technical skills, theories, and style in performance of ballet.
Outline:
- Ballet (Dance) Terminology
- Barre
- Placement and centering
- Positions of the feet
- Positions of the arms
- Positions of the torso
- Foot and leg articulation
- Balance
- Stretching
- Floor Work
- Flexibility techniques
- Various types of stretches
- Theories of stretching
- Floor positions
- Strength concepts
- Standing and Center Floor Work
- Placement and centering
- Positions of the feet
- Positions of the arms
- Positions of the torso work
- Leg and foot articulation
- Axial work
- Adagio – slow work
- Turns
- Locomotor Work
- Allegro – fast work
- Advanced combinations
- Space awareness
- Time awareness
- Energy awareness
- Advanced traveling movements
- Advanced aerial (jumping, leaping) movements
- Elements
- Energy
- Space
- Time
- Developing the Craft
- Dynamics
- Stage directions
- Basic performance skills
- Focus
- Style
Effective Term: Spring 2017 |
|
-
DNC 166 - Modern Dance I 2 Credits, 3 Contact Hours 1 lecture period 2 lab periods
Development of basic skills for dance. Includes modern dance technique at a beginning level, class protocol, warm-up, floor work, standing and center floor work, locomotor work, elements, and developing the craft.
Information: May be taken two times for a maximum of four credit hours. If this course is repeated, see a financial aid or Veteran’s Affairs advisor to determine funding eligibility as appropriate.
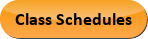
Course Learning Outcomes
- Demonstrate correct placement and alignment for modern dance at the beginning level.
- Apply basic modern dance techniques, theories, and styles at the beginning level.
- Utilize the theory of the body’s relationship to gravity and the center of gravity used in modern dance at the beginning level.
- Perform basic locomotor and axial movements in the center and across the floor at the beginning level.
- Practice the basic elements of dance: space, time, and energy at the beginning level.
- Perform modern movement in an expressive manner at the beginning level.
Outline:
- Class Protocol
- Concepts
- Warm-up
- Floor work
- Standing and center floor work
- Locomotor work
- Combination
- Cool down
- Class decorum
- Warm-Up
- Placement and centering
- Anatomy and physiology of a proper warm-up
- Floor Work
- Flexibility techniques
- Various types of stretches
- Theories of stretching
- Basic floor positions
- Floor isolations
- Upper body designs
- Strength concepts
- Standing and Center Floor Work
- Basic placement and centering
- Positions of the feet
- Positions of the arms
- Torso work
- Leg and footwork
- Axial work
- Isolations
- Turns
- Basic Locomotor Work
- Movements
- Combinations
- Traveling movements
- Aerial (jumping, leaping) movements
- Elements
- Energy
- Space
- Time
- Developing the Craft
- Dynamics
- Stage directions
- Basic performance skills
- Focus
- Style
Effective Term: Spring 2017 |
|
-
DNC 174 - Hip-Hop Dance I 2 Credits, 3 Contact Hours 1 lecture period 2 lab periods
Development of basic skills for dance. Includes hip-hop dance technique at a beginning level, class protocol, warm-up, floor work, standing and center floor work, locomotor work, elements, and developing the craft.
Information: May be taken two times for a maximum of four credit hours. If this course is repeated, see a financial aid or Veteran’s Affairs advisor to determine funding eligibility as appropriate.
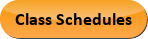
Course Learning Outcomes
- Demonstrate correct placement and alignment for hip-hop dance at the beginning level.
- Apply basic hip-hop dance techniques, theories, styles, and history at the beginning level.
- Utilize the theory of the body’s relationship to gravity and the center of gravity used in hip-hop dance at the beginning level.
- Perform basic locomotor and axial movements in the center and across the floor at the beginning level.
- Practice the basic elements of dance: space, time, and energy at the beginning level.
- Perform hip-hop movement in an expressive manner at the beginning level.
Outline:
- Class Protocol
- Concepts
- Warm-up
- Floor work
- Standing and center floor work
- Locomotor work
- Combination
- Cool down
- Class decorum
- Warm-Up
- Placement and centering
- Anatomy and physiology of a proper warm-up
- Floor Work
- Flexibility techniques
- Various types of stretches
- Theories of stretching
- Basic floor positions
- Floor isolations
- Upper body designs
- Strength concepts
- Standing and Center Floor Work
- Basic placement and centering
- Positions of the feet
- Positions of the arms
- Torso work
- Leg and footwork
- Axial work
- Isolations
- Turns
- Basic Locomotor Work
- Movements
- Combinations
- Traveling movements
- Aerial (jumping, leaping) movements
- Elements
- Energy
- Space
- Time
- Developing the Craft
- Dynamics
- Stage directions
- Basic performance skills
- Focus
- Style
Effective Term: Full Academic Year 2020/2021 |
|
-
DNC 219 - Jazz Dance I 2 Credits, 3 Contact Hours 1 lecture period 2 lab periods
Introduction and development of movement skills necessary to prepare the body as an instrument of expression in jazz dance styles. Includes class protocol, warm-up, floor work, standing and center floor work, locomotor work, elements, and developing the craft.
Information: May be taken two times for a maximum of four credit hours. If this course is repeated, see a financial aid or Veteran’s Affairs advisor to determine funding eligibility as appropriate.
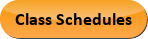
Course Learning Outcomes
- Demonstrate correct placement and alignment for jazz dance at the beginning level.
- Apply basic jazz dance technique, theories, and styles.
- Utilize the theory of the body’s relationship to gravity and the center of gravity used in jazz dance at the beginning level.
- Perform basic locomotor and axial movements in the center and across the floor at the beginning level.
- Practice the basic elements of the dance: space, time, and energy with particular attention to rhythm and syncopation.
- Practice isolation movements in syncopated rhythms with all parts of the body at the beginning level.
- Practice basic rhythmic analysis and the impact of polyrhythms and syncopation on jazz dance.
- Perform jazz dance utilizing technical skills, theories, and styles at the beginning level.
Outline:
- Class Protocol
- Concepts
- Warm-up
- Floor work
- Standing and center floor work
- Locomotor work
- Combination
- Cool down
- Class Decorum
- Warm-Up
- Placement and centering
- Anatomy and physiology of a proper warm-up
- Floor Work
- Flexibility techniques
- Various types of stretches
- Theories of stretching
- Floor positions
- Floor Isolations
- Upper body designs
- Strength concepts
- Standing and Center Floor Work
- Placement and centering
- Positions of the feet
- Positions of the arms
- Torso work
- Leg and footwork
- Axial work
- Isolations
- Turns
- Intermediate Locomotor Work
- Movements
- Combinations
- Traveling movements
- Aerial (jumping, leaping) movements
- Elements
- Energy
- Space
- Time
- Developing the Craft
- Dynamics
- Stage directions
- Basic performance skills
- Focus
- Style
Effective Term: Spring 2017 |
|
-
DNC 220 - Jazz Dance II 2 Credits, 3 Contact Hours 1 lecture period 2 lab periods
Continuation of DNC 219 . Progressive development of alignment for intermediate level jazz dance. Includes class protocol, warm-up, floor work, standing and center floor work, locomotor work, elements, and developing the craft.
Prerequisite(s): DNC 219 Information: May be taken two times for a maximum of four credit hours. If this course is repeated, see a financial aid or Veteran’s Affairs advisor to determine funding eligibility as appropriate.
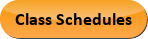
Course Learning Outcomes
- Demonstrate correct placement and alignment for jazz dance at the intermediate level.
- Apply basic jazz dance technique, theories, and styles at the intermediate level.
- Utilize the theory of the body’s relationship to gravity and the center of gravity used in jazz dance at the intermediate level.
- Perform basic locomotor and axial movements in the center and across the floor at the intermediate level.
- Practice the basic elements of dance: space, time, and energy with particular attention to rhythm and syncopation at the intermediate level.
- Practice isolation movements in syncopated rhythms with all parts of the body at the intermediate level.
- Practice basic rhythmic analysis and the impact of polyrhythms and syncopation on jazz dance at the intermediate level.
- Perform jazz dance utilizing technical skills, theories, and style at the intermediate level.
Outline:
- Class Protocol
- Concepts
- Warm-up
- Floor work
- Standing and center floor work
- Locomotor work
- Combination
- Cool down
- Class decorum
- Warm-Up
- Placement and centering
- Basic anatomy and physiology of a proper warm-up
- Floor Work
- Flexibility techniques
- Various types of stretches
- Theories of stretching
- Floor positions
- Floor isolations
- Upper body designs
- Strength concepts
- Standing and Center Floor Work
- Placement and centering
- Positions of the feet
- Positions of the arms
- Torso work
- Leg and footwork
- Axial work
- Isolations
- Intermediate turns and beginning turning combinations
- Intermediate Locomotor Work
- Across the floor movements
- Combinations
- Traveling movements
- Aerial (jumping, leaping) movements
- Elements
- Energy
- Space
- Time
- Developing the Craft
- Dynamics
- Stage directions
- Intermediate performance skills
- Focus
- Style
Effective Term: Spring 2017 |
|
-
DNC 221 - Jazz Dance III 2 Credits, 3 Contact Hours 1 lecture period 2 lab periods
Continuation of DNC 220 . Progressive development of alignment for advanced level jazz dance. Includes class protocol, warm-up, floor work, standing and center floor work, locomotor work, elements, and developing the craft.
Prerequisite(s): DNC 220 Information: May be taken two times for a maximum of four credit hours. If this course is repeated, see a financial aid or Veteran’s Affairs advisor to determine funding eligibility as appropriate.
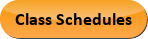
Course Learning Outcomes
- Demonstrate correct placement and alignment for jazz dance at a more advanced level.
- Apply basic jazz dance technique, theories, and styles at a more advanced level.
- Utilize the theory of the body’s relationship to gravity and the center of gravity used in jazz dance at a more advanced level.
- Perform basic locomotor and axial movements in the center and across the floor at a more advanced level.
- Practice the basic elements of dance: space, time, and energy with particular attention to rhythm and syncopation at a more advanced level.
- Practice isolation movements in syncopated rhythms with all parts of the body at a more advanced level.
- Practice basic rhythmic analysis and the impact of polyrhythms and syncopation on jazz dance at a more advanced level.
- Perform jazz dance utilizing technical skills, theories, and style at a more advanced level.
Outline:
- Class Protocol
- Concepts
- Warm-up
- Floor work
- Standing and center floor work
- Locomotor work
- Combination
- Cool down
- Class decorum
- Warm-Up
- Placement and centering
- Anatomy and physiology of a proper warm-up
- Floor Work
- Flexibility techniques
- Various types of stretches
- Theories of stretching
- Floor positions
- Floor isolations
- Upper body designs
- Strength concepts of the upper and lower extremities
- Standing and Center Floor Work
- Placement and centering
- Positions of the feet
- Positions of the arms
- Torso work
- Leg and footwork
- Axial work
- Isolations
- Advanced turns and turning combinations
- Advanced Locomotor Work
- Across the floor movements
- Combinations
- Traveling movements
- Aerial (jumping, leaping) movements
- Elements
- Energy
- Space
- Time
- Developing the Craft
- Dynamics
- Stage directions
- Advanced performance skills
- Focus
- Style
Effective Term: Spring 2017 |
|
-
DNC 269 - Dance Ensemble 1 Credits, 3 Contact Hours 0 lecture periods 3 lab periods
Practical experience in all aspects for taking a dance piece from basic choreography and creating a professional performance. Includes rehearsal/performance process, responsibilities of a performer and/or a choreographer, performance skills, production elements, and publicity.
Prerequisite(s): DNC 150 or DNC 166 or DNC 219 .
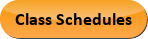
Course Learning Outcomes
- Perform or create choreography for a dance concert.
- Demonstrate the understanding of one element of dance production (audio, visual, costuming, use of makeup, sets/props, or marketing)
Outline:
- Rehearsal/Performance Process
- Audition process
- Casting
- Scheduling
- Preliminary rehearsals
- Technical rehearsals
- Dress rehearsals
- Performance
- Post-performance process
- Responsibilities of a Performer
- During rehearsal
- Attendance
- Attitude
- Practice
- Teamwork
- Performance
- Call
- Preparation
- Mental focus
- Projection
- Professional image
- Production Elements
- Costuming
- Make-up
- Stage
- Sets/Props
- Audio-visual elements
- Publicity
- Public Service Announcements (PSA’s)
- Press releases
- Photo shoots
- Social Media
- Posters and flyers
- Other
*This class is geared toward producing a dance concert. Students will learn and demonstrate one production skill in addition to performance or choregraphy each semester they are enrolled.
Effective Term: Spring 2017 |
Dental Assisting Education |
|
-
DAE 159 - Introduction to Health Care for Dental Assisting 2 Credits, 2 Contact Hours 2 lecture periods 0 lab periods
Basic skills essential to working successfully with patients and co-workers in dental offices and clinics, as a member of the dental health team. Includes study skills, psychology, vital signs, communication in the dental environment, job entry skills, research, and oral speech projects.
Corequisite(s): DAE 160 , DAE 161 , DAE 162 , DAE 162LB , DAE 163 , DAE 163LC , DAE 164 , DAE 164LB , DAE 165 , DAE 165LC Information: Consent of program coordinator is required before enrolling in this course.
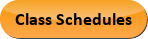
Course Learning Outcomes
- Demonstrate competency in the knowledge and skills required to systematically collect diagnostic data.
- Demonstrate competency in the knowledge and skills required to perform a variety of business office procedures.
- Demonstrate competency in the knowledge and skills required to manage infection hazard control protocol consistent with published professional guidelines.
- Define and explain state and federal dental laws and regulations.
Outline:
- Study Skills and Psychology
- College study skills
- Reading skills/note taking
- Learning styles
- Learning skills
- Library – computer lab orientations/skills
- Critical thinking
- Psychology of human behavior
- Goal setting/Maslow’s hierarchy of needs
- Stress and stress management
- Patient fear of dentistry
- Concepts of wellness
- Effects of health and disease
- Vital Signs
- Temperature
- Pulse
- Respiration
- Blood pressure
- Communication in the Dental Environment
- Teamwork and office communication
- Verbal and non-verbal communication
- Listening skills
- The handicapped patient
- The elderly patient
- The child and adolescent patient
- Job Entry Skills
- The job search
- Job application
- Resume and cover letter
- The Job interview
- Role playing
- Working interview
- Research and Oral Speech Projects
- Research paper
- Access networked resources
- Use library/periodicals
- Oral presentation to class
- Group dynamics
- Critiques
Effective Term: Full Academic Year 2017/18 |
|
-
DAE 160 - Orientation to Dental Care 1 Credits, 1 Contact Hours 1 lecture period 0 lab periods
Overview of the field of dental care. Includes the profession of dentistry, areas of service, ethics, and jurisprudence.
Corequisite(s): DAE 159 , DAE 161 , DAE 162 , DAE 162LB , DAE 163 , DAE 163LC , DAE 164 , DAE 164LB , DAE 165 , DAE 165LC Information: Consent of program coordinator is required before enrolling in this course.
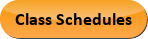
Course Learning Outcomes
- Demonstrate competency in the knowledge and skills required to perform a variety of business office procedures.
- Demonstrate competency in the knowledge and skills required to manage infection hazard control protocol consistent with published professional guidelines.
- Define and explain state and federal dental laws and regulations.
Outline:
- Profession of Dentistry
- History of the profession
- Dental assisting
- Dental laboratory technology
- Dental hygiene
- Areas of Service
- General dentistry
- Specialties
- Orthodontics, periodontics, endodontics, pediatric dentistry
- Oral Surgery, prosthodontics, oral pathology, public health
- Hospital dentistry
- Dental schools
- Ethics and Jurisprudence
- Arizona State Dental Practice Act
- Certification
- Registration
- Licensure
- Professionalism
- Ethical standards
- Malpractice
Effective Term: Full Academic Year 2017/18 |
|
-
DAE 161 - Biomedical Dental Science 3 Credits, 3 Contact Hours 3 lecture periods 0 lab periods
Biosciences as they relate to the oral cavity. Impacts of anatomy, physiology, microbiology, oral pathology, and nutrition on dental health.
Corequisite(s): DAE 159 , DAE 160 , DAE 162 , DAE 162LB , DAE 163 , DAE 163LC , DAE 164 , DAE 164LB , DAE 165 , DAE 165LC Information: Consent of program coordinator is required before enrolling in this course.
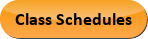
Course Learning Outcomes
- Demonstrate competency in the knowledge and skills required to manage infection hazard control protocol consistent with published professional guidelines.
- Demonstrate knowledge of biomedical dental science.
- Define and explain state and federal dental laws and regulations.
Outline:
- Anatomy and Physiology
- Systems of the body
- Related systems as applied to dental health
- Head and neck anatomy
- Oral anatomy
- Oral cavity
- Microbiology, Infection Control, and Oral Pathology
- Microbiology
- Types of microorganisms
- Transmission of diseases
- Methods of sterilization and effects on bacterial and viral growth
- Prevention of disease
- Sterilization
- Barrier protection
- Infection Control
- Infection control guidelines/OSHA
- Bloodborne pathogen exposure
- Protective barrier techniques
- Personal protective barrier
- Treatment room cleaning and disinfection
- Oral Pathology
- Review of histology
- Injury and repair
- Inflammation
- Repair
- Tissue changes
- Neoplasia
- Inflammatory disease of the oral cavity
- Miscellaneous disorders
- Nutrition
- Social and environmental nutrition
- Proteins, carbohydrates, and fats
- Social diet
- Physical body chemistry
- Physiological and psychological stress
Effective Term: Full Academic Year 2017/18 |
|
-
DAE 162 - Dental Assisting I 2 Credits, 2 Contact Hours 2 lecture periods 0 lab periods
Principles and techniques of dental assisting. Includes tooth morphology of human dentition, hand and rotary dental instruments, instruments used in various operative procedures, and chairside procedures.
Corequisite(s): DAE 159 , DAE 160 , DAE 161 , DAE 162LB , DAE 163 , DAE 163LC , DAE 164 , DAE 164LB , DAE 165 , DAE 165LC Information: Consent of program coordinator is required before enrolling in this course.
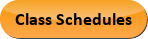
Course Learning Outcomes
- Demonstrate competency in the knowledge and skills required to systematically collect diagnostic data.
- Demonstrate competency in the knowledge and skills required to perform a variety of clinical supportive treatments.
- Demonstrate competency in the knowledge and skills required to perform a variety of business office procedures.
- Demonstrate competency in the knowledge and skills required to manage infection hazard control protocol consistent with published professional guidelines.
- Demonstrate knowledge of biomedical dental science.
- Define and explain state and federal dental laws and regulations.
Outline:
- Tooth Morphology of Human Dentition
- Dental terminology
- Morphology
- Charting
- Hand and Rotary Dental Instruments
- Hand cutting instruments
- Conventional handpieces
- High speed handpieces
- Rotary instruments
- Matrix
- Miscellaneous dental instruments
- Surgical instruments
- Orthodontic, periodontal, endodontic and prosthodontic instruments
- Sharpening hand instruments
- Instrument sterilization/asepsis
- Chairside Procedures
- Rubber dam
- Indications for use in dental procedures
- Identifying armamentarium
- Placement
- Removal
- Temporary crowns
- Indications for use in dental procedures
- Types
- Instrumentation
- Suture removal
- Indications for use
- Types
- Instrumentation
Effective Term: Full Academic Year 2017/18 |
|
-
DAE 162LB - Dental Assisting I Lab 1 Credits, 3 Contact Hours 0 lecture periods 3 lab periods
This is the lab portion of DAE 162. Principles and techniques of dental assisting. Includes tooth morphology of human dentition, hand and rotary dental instruments, instruments used in various operative procedures, and chairside procedures.
Corequisite(s): DAE 159 , DAE 160 , DAE 161 , DAE 162 , DAE 163 , DAE 163LC , DAE 164 , DAE 164LB , DAE 165 , DAE 165LC Information: Consent of program coordinator is required before enrolling in this course.
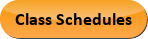
Course Learning Outcomes
- Demonstrate competency in the knowledge and skills required to systematically collect diagnostic data.
- Demonstrate competency in the knowledge and skills required to perform a variety of clinical supportive treatments.
- Demonstrate competency in the knowledge and skills required to perform a variety of business office procedures.
- Demonstrate competency in the knowledge and skills required to manage infection hazard control protocol consistent with published professional guidelines.
- Demonstrate knowledge of biomedical dental science.
- Define and explain state and federal dental laws and regulations.
Outline:
- Tooth Morphology of Human Dentition
- Dental terminology
- Morphology
- Charting
- Hand and Rotary Dental Instruments
- Hand cutting instruments
- Conventional handpieces
- High speed handpieces
- Rotary instruments
- Matrix
- Miscellaneous dental instruments
- Surgical instruments
- Orthodontic, periodontal, endodontic and prosthodontic instruments
- Sharpening hand instruments
- Instrument sterilization/asepsis
- Chairside Procedures
- Rubber dam
- Indications for use in dental procedures
- Identifying armamentarium
- Placement
- Removal
- Temporary crowns
- Indications for use in dental procedures
- Types
- Instrumentation
- Suture removal
- Indications for use
- Types
- Instrumentation
Effective Term: Full Academic Year 2017/18 |
|
-
DAE 163 - Oral Radiography 2 Credits, 2 Contact Hours 2 lecture periods 0 lab periods
Principles to dental radiography as a diagnostic aid. Includes radiation protection and biology. Also includes clinic experience in exposing, processing, mounting, and interpreting radiographs on mannequins and patients using a variety of radiographic techniques.
Corequisite(s): DAE 159 , DAE 160 , DAE 161 , DAE 162 , DAE 162LB , DAE 163LC , DAE 164 , DAE 164LB , DAE 165 , DAE 165LC Information: Consent of program coordinator is required before enrolling in this course. DHE 116 can be substituted for DAE 163 if completed within the last three years, see academic advisor or faculty for information regarding course substitution. This course meets the CODA Standard for the Dental Hygiene Education program for Advanced Standing (2-4) for the course, DHE116, in the PCC Dental Hygiene program.
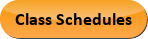
Course Learning Outcomes
- Demonstrate competency in the knowledge and skills required to systematically collect diagnostic data
- Define and explain state and federal dental laws and regulations
- Demonstrate competency in the knowledge and skills required to manage infections hazard control protocol consistent with published professional guidelines
- Demonstrate competency in the knowledge and skills in dental radiology
- Demonstrate competency in interpreting normal and abnormal anatomy and conditions
Performance Objectives:
- Identify the important people and events in the history of radiography.
- State the principles of radiation production.
- Identify and demonstrate ALARA methods of radiation production for the patient and operator.
- Operate and maintain x-ray units safely.
- Identify radiographic films as to size and use.
- Process radiographic films manually.
- Practice quality assurance procedures.
- Identify radiographic anatomical landmarks.
- Perform radiograph mounting.
- Interpret normal and abnormal pathological condition visible on dental radiographs.
- Describe and perform radiographic film exposure techniques.
- Describe and perform digital radiography exposure techniques.
- Identify and correct undiagnostic radiographs.
- Evaluate radiographic quality.
- Complete a variety of radiographs on mannequin and patients.
- Identify and perform infection control precautions as specified by the Occupational Safety and Health Administration (OSHA) and program policy and Health Administration (OSHA) and program policy.
Outline:
- Introduction to Radiography
- History of radiography
- Production and characteristics of x-radiation
- Dental x-ray equipment
- Components
- Parts
- Inverse Square Law
- Radiation Health and Safety
- Radiation safety legislation
- Radiation measurement terminology
- Measuring and monitoring devices
- Protection measures for the patient
- Radiation protection for operators
- Maximum permissible doses
- Dental Film
- Film emulsion and speeds
- Packaging, storage, and protection of film
- Types of intraoral and extraoral films
- Handling cassettes
- Film Processing
- Darkroom
- Equipment
- Illumination
- Maintenance
- Processing solutions and procedures
- Inadequacies caused by faulty processing techniques
- Film duplication
- Xeroradiography
- Digital Radiography
- Identification of Anatomical Landmarks for Mounting and Interpretation
- Radiolucent and radiopaque images
- Alveolar bone and supporting structures
- Landmarks identified
- Mounting, labeling, filing, and storage of radiographs
- Film viewing and preliminary radiographic interpretation
- Abnormal and normal pathological conditions
- Exposure Techniques
- Criteria for intraoral radiographs
- Horizontal and vertical angulation
- Principles of bisecting and paralleling techniques
- The periapical examination
- Fundamentals of interproximal radiography
- Radiography for children
- Patient management
- Infection control
- Evaluation of radiographic quality
- Digital radiography
- Principles of Supplementary Film
- Occlusal surveys
- Types of use of extraoral film
- Lateral jaw survey
- Temporomandibular articulation survey
- Cephalometric radiography
- Panoramic radiography
- Other imaging systems
Effective Term: Fall 2022 |
|
-
DAE 163LC - Oral Radiography Clinical Lab 1 Credits, 4 Contact Hours 0 lecture periods 4 lab periods
This is the clinical portion of DAE 163 . Principles to dental radiography as a diagnostic aid. Includes radiation production and biology. Also includes clinic experience in exposing, processing, mounting, and interpreting radiographs on mannequins and patients using a variety of radiographic techniques.
Corequisite(s): DAE 159 , DAE 160 , DAE 161 , DAE 162 , DAE 162LB , DAE 163 , DAE 164 , DAE 164LB , DAE 165 , DAE 165LC Information: Consent of program coordinator is required before enrolling in this course. DHE 116LC can be substituted for DAE 163LC if completed within the last three years, see academic advisor or faculty for information regarding course substitution. This course meets the CODA Standard for the Dental Hygiene Education program for Advanced Standing (2-4) for the course, DHE116LC, in the PCC Dental Hygiene program.
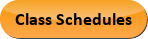
Course Learning Outcomes
- Demonstrate competency in the knowledge and skills required to systematically collect diagnostic data.
- Demonstrate competency in the knowledge and skills required to manage infection hazard control protocol consistent with published professional guidelines.
- Demonstrate knowledge of biomedical dental science.
- Define and explain state and federal dental laws and regulations.
- Demonstrate competency in the knowledge and skills in dental radiology.
- Complete a variety of radiographs on mannequins and patients.
Outline:
- Introduction to Radiography
- History of radiography
- Production and characteristics of x-radiation
- Dental x-ray equipment
- Components
- Parts
- Inverse Square Law
- Radiation Health and Safety
- Radiation safety legislation
- Radiation measurement terminology
- Measuring and monitoring devices
- Protection measures for the patient
- Radiation protection for operators
- Maximum permissible doses
- Dental Film
- Film emulsion and speeds
- Packaging, storage, and protection of film
- Types of intraoral and extraoral films
- Handling cassettes
- Film Processing
- Darkroom
- Equipment
- Illumination
- Maintenance
- Processing solutions and procedures
- Inadequacies caused by faulty processing techniques
- Film duplication
- Xeroradiography
- Digital Radiography
- Identification of Anatomical Landmarks for Mounting and Interpretation
- Radiolucent and radiopaque images
- Alveolar bone and supporting structures
- Landmarks identified
- Mounting, labeling, filing, and storage of radiographs
- Film viewing and preliminary radiographic interpretation
- Abnormal and normal pathological conditions
- Exposure Techniques
- Criteria for intraoral radiographs
- Horizontal and vertical angulation
- Principles of bisecting and paralleling techniques
- The periapical examination
- Fundamentals of interproximal radiography
- Radiography for children
- Patient management
- Infection control
- Evaluation of radiographic quality
- Digital radiography
- Principles of Supplementary Film
- Occlusal surveys
- Types of use of extraoral film
- Lateral jaw survey
- Temporomandibular articulation survey
- Cephalometric radiography
- Panoramic radiography
- Other imaging systems
New Outline:
- Introduction to Radiography
- History of radiography
- Production and characteristics of x-radiation
- Dental x-ray equipment
- Components
- Parts
- Inverse Square Law
- Radiation Health and Safety
- Radiation safety legislation
- Radiation measurement terminology
- Measuring and monitoring devices
- Protection measures for the patient
- Radiation protection for operators
- Maximum permissible doses
- Dental Film
- Film emulsion and speeds
- Packaging, storage, and protection of film
- Types of intraoral and extraoral films
- Handling cassettes
- Film Processing
- Darkroom
- Equipment
- Illumination
- Maintenance
- Processing solutions and procedures
- Inadequacies caused by faulty processing techniques
- Film duplication
- Xeroradiography
- Digital Radiography
- Identification of Anatomical Landmarks for Mounting and Interpretation
- Radiolucent and radiopaque images
- Alveolar bone and supporting structures
- Landmarks identified
- Mounting, labeling, filing, and storage of radiographs
- Film viewing and preliminary radiographic interpretation
- Abnormal and normal pathological conditions
- Exposure Techniques
- Criteria for intraoral radiographs
- Horizontal and vertical angulation
- Principles of bisecting and paralleling techniques
- The periapical examination
- Fundamentals of interproximal radiography
- Radiography for children
- Patient management
- Infection control
- Evaluation of radiographic quality
- Digital radiography
- Principles of Supplementary Film
- Occlusal surveys
- Types of use of extraoral film
- Lateral jaw survey
- Temporomandibular articulation survey
- Cephalometric radiography
- Panoramic radiography
- Other imaging systems
Effective Term: Fall 2022 |
|
-
DAE 164 - Dental Materials 2 Credits, 2 Contact Hours 2 lecture periods 0 lab periods
Chemical and physical properties of dental materials used in dental practice. Includes introduction to dental materials, preventive sealants, restorative materials, dental cements, impression materials, gypsum products, and miscellaneous dental materials. Also includes gold, non-precious alloys, and casting of metals.
Corequisite(s): DAE 159 , DAE 160 , DAE 161 , DAE 162 , DAE 162LB , DAE 163 , DAE 163LC , DAE 164LB , DAE 165 , DAE 165LC Information: Consent of program coordinator is required before enrolling in this course. DHE 132 can be substituted for DAE 164 if completed within the last three years, see academic advisor or faculty for information regarding course substitution. This course meets the CODA Standard for the Dental Hygiene Education program for Advanced Standing (2-4) for the course, DHE 132, in the PCC Dental Hygiene program.
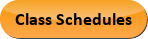
Course Learning Outcomes
- Demonstrate proper use of Personal Protective Equipment (PPE), Occupational Safety and Health Administration (OSHA) standards, the identification of Globally Harmonized System (GHS), and manufacturer’s’ Safety Data Sheets (SDS).
- Define and explain state and federal dental laws and regulations.
- Compare and contrast different dental restorative materials and their biocompatibility significance.
- Explain the importance of the study of dental materials.
- Identify, synthesize, and evaluate dental materials in radiographic imagery.
- Demonstrate effective communication skills using verbal, non-verbal, and written forms.
- Demonstrate knowledge of properties and manipulation of dental materials.
- Demonstrate at competency level the use of various types of dental materials
Performance Objectives:
- Identify the properties of matter and material
- Operatie and maintain laboratory equipment safetly
- Prepare dental waxes, cements, preventive sealants, and restorative materials.
- Explain the importance of the study of dental materials
- Identify, synthesize, and evaluate dental materials in radiographic imagery.
- Demonstrate effective communication skills using verbal, non-verbal, and written forms.
- Demonstrate knowledge of properties and manipulation of dental materials
- Demonstrate at compentency level the use of various types of dental materials
Outline:
- Introduction to Dental Materials
- American Dental Association (ADA) specifications
- Proper use of PPE with guidance by the Center of Disease Control (CDC)
- Properties of matter
- Physical and chemical properties required for dental materials
- Precautions
- Handling materials to the standards of Environmental Protection Agency (EPA) and Pima’s Environmental Health and Safety (EHS)
- Hazardous substances as labeled by Globally Harmonized System (GHS)
- Occupational Safety and Health Administration (OSHA) regulations
- Safe use of laboratory equipment
- Arizona State dental statutes and regulations for auxiliary practitioners
- Preventive Sealants and Restorative Materials
- Types of preventive sealants
- Indications for use
- Properties, advantages, and disadvantages
- Manipulation
- Direct filling materials and armamentarium
- Esthetic/ Resins
- Amalgam
- Finish and polish esthetic and amalgam restorations
- Matrices
- Rubber dam
- Dental Cements
- Temporary and permanent cements and cement bases
- Composition and uses of various types
- Manipulation of cements
- Cavity liners and varnishes
- Special applications of cements
- Impression Materials
- Types of impression materials
- Types of impression trays
- Custom, mouth guard, and whitening trays
- Agar hydrocolloid (reversible hydrocolloid)
- Rubber impression materials and elastomers
- Alginate
- Bite registration
- Disinfection of impressions
- Gypsum Products
- Classification of uses of gypsum products
- Composition and manufacture
- Variables
- Manipulation
- Construction of model, cast, or die
- Modeling trimming
- Articulation
- Miscellaneous Dental Materials
- Uses of resins and acrylic
- Waxes
- Dental ceramics and porcelain
- Polishing and cleaning agents
- Periodontal dressings
- Provisional restorations
- Gold, Non-Precious Alloys, and Casting of Metals
- Gold foil
- Gold casting alloys
- Wrought metals
- Dental implant materials
Effective Term: Fall 2022 |
|
-
DAE 164LB - Dental Materials Lab 1 Credits, 3 Contact Hours 0 lecture periods 3 lab periods
This is the lab portion of DAE 164 . Chemical and physical properties of dental materials used in dental practice. Includes introduction to dental materials, preventive sealants, restorative materials, dental cements, impression materials, gypsum products, and miscellaneous dental materials. Also includes gold, non-precious alloys, and casting of metals.
Corequisite(s): DAE 159 , DAE 160 , DAE 161 , DAE 162LB , DAE 162 , DAE 163LC , DAE 163 , DAE 164 , DAE 165LC , DAE 165 Information: Consent of program coordinator is required before enrolling in this course. DHE 132LB can be substituted for DAE 164LB if completed within the last three years, see academic advisor or faculty for information regarding course substitution. This course meets the CODA Standard for the Dental Hygiene Education program for Advanced Standing (2-4) for the course, DHE 116LC, in the PCC Dental Hygiene program
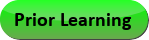.png)
Course Learning Outcomes
- Demonstrate proper use of Personal Protective Equipment (PPE), Occupational Safety and Health Administration (OSHA) standards, the identification of Globally Harmonized System (GHS), and manufacturer’s’ Safety Data Sheets (SDS).
- Define and explain state and federal dental laws and regulations.
- Compare and contrast different dental restorative materials and their biocompatibility significance.
- Explain the importance of the study of dental materials.
- Identify, synthesize, and evaluate dental materials in radiographic imagery.
- Demonstrate effective communication skills using verbal, non-verbal, and written forms.
- Demonstrate knowledge of properties and manipulation of dental materials.
- Demonstrate at competency level the use of various types of dental materials.
Performance Objectives:
- Identify the properties of matter and materials.
- Operate and maintain laboratory equipment safely.
- Prepare dental waxes, cements, preventive sealants, and restorative materials.
- Prepare materials to make occlusal registration for articulating study casts.
- Prepare and pour elastic, plastic, and rigid impression materials.
- Prepare and pour study casts using gypsum products.
- Fabricate trays, e.g. custom trays, mouth guard, and whitening trays.
- Fabricate provisional restorations.
- Describe casting techniques and identify metals used in the fabrication of dental prostheses.
- Prepare, place, and finish composite and amalgam restorations.
Outline:
- Introduction to Dental Materials
- American Dental Association (ADA) specifications
- Proper use of PPE with guidance by the Center of Disease Control (CDC)
- Properties of matter
- Physical and chemical properties required for dental materials
- Precautions
- Handling materials to the standards of Environmental Protection Agency (EPA) and Pima’s Environmental Health and Safety (EHS)
- Hazardous substances as labeled by Globally Harmonized System (GHS)
- Occupational Safety and Health Administration (OSHA) regulations
- Safe use of laboratory equipment
- Arizona State dental statutes and regulations for auxiliary practitioners
- Preventive Sealants and Restorative Materials
- Types of preventive sealants
- Indications for use
- Properties, advantages, and disadvantages
- Manipulation
- Direct filling materials and armamentarium
- Esthetic/ Resins
- Amalgam
- Finish and polish esthetic and amalgam restorations
- Matrices
- Rubber dam
- Dental Cements
- Temporary and permanent cements and cement bases
- Composition and uses of various types
- Manipulation of cements
- Cavity liners and varnishes
- Special applications of cements
- Impression Materials
- Types of impression materials
- Types of impression trays
- Custom, mouth guard, and whitening trays
- Agar hydrocolloid (reversible hydrocolloid)
- Rubber impression materials and elastomers
- Alginate
- Bite registration
- Disinfection of impressions
- Gypsum Products
- Classification of uses of gypsum products
- Composition and manufacture
- Variables
- Manipulation
- Construction of model, cast, or die
- Modeling trimming
- Articulation
- Miscellaneous Dental Materials
- Uses of resins and acrylic
- Waxes
- Dental ceramics and porcelain
- Polishing and cleaning agents
- Periodontal dressings
- Provisional restorations
- Gold, Non-Precious Alloys, and Casting of Metals
- Gold foil
- Gold casting alloys
- Wrought metals
- Dental implant materials
Effective Term: Fall 2022 |
|
-
DAE 165 - Dental Assisting Procedures I 1 Credits, 1 Contact Hours 1 lecture period 0 lab periods
Techniques and procedures of chairside dental assisting. Includes dental equipment and room design; chairside assisting and team approach; procedures applied in clinical treatment; and computer systems and technology in the dental environment. Also includes the application of student supervised experience in performing dental assisting functions in the clinical setting on patients.
Corequisite(s): DAE 159 , DAE 160 , DAE 161 , DAE 162 , DAE 162LB , DAE 163 , DAE 163LC , DAE 164 , DAE 164LB , DAE 165LC Information: Consent of program coordinator is required before enrolling in this course.
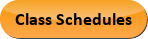
Course Learning Outcomes
- Demonstrate competency in the knowledge and skills required to systematically collect diagnostic data.
- Demonstrate competency in the knowledge and skills required to perform a variety of clinical supportive treatments.
- Demonstrate competency in the knowledge and skills required to perform a variety of business office procedures.
- Demonstrate competency in the knowledge and skills required to manage infection hazard control protocol consistent with published professional guidelines.
- Define and explain state and federal dental laws and regulations.
Outline:
- Dental Equipment and Room Design
- Equipment
- Dental chair
- Operator’s/assistant’s chair
- Dental unit
- Mobile unit
- Dental light
- Central vacuum
- Care of equipment
- Zones of operation
- Placement of operating equipment
- Positions of participants
- Classification of motions
- Preparation of patient
- Seating and draping
- Receiving and dismissing
- Charts and radiographs available
- Chairside Assisting and Team Approach: Four-Six Handed Dentistry
- Oral evacuation
- Suction tip and mirror placement
- Instrument transfer
- Handpiece transfer
- Stages of instrument transfer
- Preparing set-ups
- Basic examinations
- Oral examinations
- Anesthetic tray set-up
- Chairside Procedures Applied in Clinical Treatment
- Restorative tray set-up
- Periodontic tray set-up
- Surgical tray set-up
- Endodontic tray set-up
- Orthodontic tray set-up
- Fixed prosthodontic tray set-up
- Prosthdontic tray set-up
- Pediatric dentistry
- Application of sterilization, sanitation, and disinfection where applicable
- Computer Systems and Technology in the Dental Environment
- Charting and recording dental conditions
- Documenting treatment and treatment plan
- Application of Proper Dental Assisting Procedures Utilizing Live Patients
Effective Term: Full Academic Year 2017/18 |
|
-
DAE 165LC - Dental Assisting Clinical Procedures I 1 Credits, 4 Contact Hours 0 lecture periods 4 lab periods
Dental Assisting Procedures Clinical I This is the clinical portion of DAE 165 . Techniques and procedures of chairside dental assisting. Includes dental equipment and room design; chairside assisting and team approach; procedures applied in clinical treatment; and computer systems and technology in the dental environment. Also includes the application of student supervised experience in performing dental assisting functions in the clinical setting on patients.
Corequisite(s): DAE 159 , DAE 160 , DAE 161 , DAE 162 , DAE 162LB , DAE 163 , DAE 163LC , DAE 164 , DAE 164LB , DAE 165 Information: Consent of program coordinator is required before enrolling in this course.
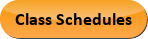
Course Learning Outcomes
- Demonstrate competency in the knowledge and skills required to systematically collect diagnostic data.
- Demonstrate competency in the knowledge and skills required to perform a variety of clinical supportive treatments.
- Demonstrate competency in the knowledge and skills required to perform a variety of business office procedures.
- Demonstrate competency in the knowledge and skills required to manage infection hazard control protocol consistent with published professional guidelines.
- Define and explain state and federal dental laws and regulations.
Outline:
- Dental Equipment and Room Design
- Equipment
- Dental chair
- Operator’s/assistant’s chair
- Dental unit
- Mobile unit
- Dental light
- Central vacuum
- Care of equipment
- Zones of operation
- Placement of operating equipment
- Positions of participants
- Classification of motions
- Preparation of patient
- Seating and draping
- Receiving and dismissing
- Charts and radiographs available
- Chairside Assisting and Team Approach: Four-Six Handed Dentistry
- Oral evacuation
- Suction tip and mirror placement
- Instrument transfer
- Handpiece transfer
- Stages of instrument transfer
- Preparing set-ups
- Basic examinations
- Oral examinations
- Anesthetic tray set-up
- Chairside Procedures Applied in Clinical Treatment
- Restorative tray set-up
- Periodontic tray set-up
- Surgical tray set-up
- Endodontic tray set-up
- Orthodontic tray set-up
- Fixed prosthodontic tray set-up
- Prosthdontic tray set-up
- Pediatric dentistry
- Application of sterilization, sanitation, and disinfection where applicable
- Computer Systems and Technology in the Dental Environment
- Charting and recording dental conditions
- Documenting treatment and treatment plan
- Application of Proper Dental Assisting Procedures Utilizing Live Patients
Effective Term: Full Academic Year 2017/18 |
|
-
DAE 166 - Dental Assisting II 3 Credits, 3 Contact Hours 3 lecture periods 0 lab periods
Principles and techniques of dental assisting. Includes pharmacology and therapeutics; and dental office inventory control. Also includes techniques and procedures for emergency medical/dental care as applied to dental assisting.
Prerequisite(s): DAE 159 , DAE 160 , DAE 161 , DAE 162 , DAE 162LB , DAE 163 , DAE 163LC , DAE 164 , DAE 164LB , and DAE 165 . Corequisite(s): DAE 167 , DAE 169 , DAE 169LC Information: Consent of program coordinator is required before enrolling in this course.
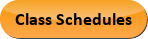
Course Learning Outcomes
- Demonstrate competency in the knowledge and skills required to systematically collect diagnostic data.
- Demonstrate competency in the knowledge and skills required to perform a variety of clinical supportive treatments.
- Demonstrate competency in the knowledge and skills required to perform a variety of business office procedures.
- Demonstrate competency in the knowledge and skills required to manage infection hazard control protocol consistent with published professional guidelines.
- Demonstrate knowledge of biomedical dental science.
- Define and explain state and federal dental laws and regulations.
Outline:
- Pharmacology and Therapeutics
- General principles of drug action
- Characterization of drug actions
- Route of drug administration
- Definitions and general introductory considerations
- Terms
- Pharmacology
- Pharmacognosy
- Posology
- Toxicology
- Pharmacodynamics
- Pharmacology in dental practice
- Publications in pharmacology
- Drug nomenclature
- Federal regulatory agencies
- The Bureau of Narcotic and Dangerous Drugs (BNDD)
- Prescription writing, sedative and hypnotic drugs (barbiturates)
- Parental sedation and nitrous oxide analgesia
- General considerations
- Psychological aspects of patient management
- Medical history
- Limitations of oral sedation
- Agents and adverse effects
- General anesthesia
- Chemical properties
- Physical properties, uptake, and elimination
- Pharmacologic effects of the central nervous system
- Agents and adverse effects
- Local Anesthetics
- Chemical properties
- Pharmacologic effects on the peripheral nervous system
- Agents and adverse effects
- Emergency Medical/Dental Care
- Dental office emergencies
- Health histories
- Emergency kits
- Oxygen therapy
- Epileptic convulsions
- Diabetes
- Hypertension
- Hemorrhage
- Allergic reaction
- Dental emergencies
- Summary and evaluation
- Dental Office Inventory Control
- Types of inventory systems
- Ordering
- Expendable/non-expendable supplies
- Receiving orders
Effective Term: Full Academic Year 2017/18 |
|
-
DAE 167 - Dental Assisting III 3 Credits, 3 Contact Hours 3 lecture periods 0 lab periods
Principles and techniques of dental practices management and oral health education as applied to dental assisting. Includes preventive dentistry in dental health education, dental office procedures, and summary and evaluation.
Prerequisite(s): DAE 159 , DAE 160 , DAE 161 , DAE 162 , DAE 162LB , DAE 163 , DAE 163LC , DAE 164 , DAE 164LB , DAE 165 , and DAE 165LB. Corequisite(s): DAE 166 , DAE 169 , DAE 169LC Information: Consent of program coordinator is required before enrolling in this course.
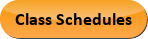
Course Learning Outcomes
- Demonstrate competency in the knowledge and skills required to systematically collect diagnostic data.
- Demonstrate competency in the knowledge and skills required to perform a variety of clinical supportive treatments.
- Demonstrate competency in the knowledge and skills required to perform a variety of business office procedures.
- Demonstrate competency in the knowledge and skills required to manage infection hazard control protocol consistent with published professional guidelines.
- Define and explain state and federal dental laws and regulations.
Outline:
- Preventive Dentistry in Dental Health Education
- Periodontal disease
- Home care
- Patient education
- Fluoride
- Coronal polish
- Miscellaneous preventive measures
- Care of prosthetic devices
- Public dental health
- Dental Office Procedures
- Duties of a dental secretary
- Office manual
- Telephone techniques
- Appointment control
- Record keeping
- Accounts receivable
- Dental insurance
- Recall systems
- Preventing disease transmission in records management
- Accounts payable
- Computers in practice management
- Inventory control
- Summary and Evaluation
Effective Term: Full Academic Year 2017/18 |
|
-
DAE 169 - Dental Assisting Procedures II 0.5 Credits, 0.5 Contact Hours .5 lecture periods 0 lab periods
Application and evaluation of skills acquired in a clinical environment. Includes specialty seminars and guest lecturers; rotations to specialty practices; first and second clinical externship rotation assignments; discussion and analysis of clinical externship; and evaluation process.
Prerequisite(s): DAE 159 , DAE 160 , DAE 161 , DAE 162 , DAE 162LB , DAE 163 , DAE 163LC , DAE 164 , DAE 164LB , DAE 165 , and DAE 165LC . Corequisite(s): DAE 166 , DAE 167 , DAE 169LC Information: Consent of program coordinator is required before enrolling in this course.
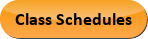
Course Learning Outcomes
- Demonstrate clinical experience and competency performing dental assisting functions.
Outline:
- Specialty Seminars and Guest Lecturers
- Orthodontist
- Oral surgeon
- Endodontist
- Pediatric dentist
- Prosthodontist
- TMD specialist
- Public health
- Periodontist
- Dental anesthesiologist
- Rotations to Specialty Practices
- First Clinical Externship Rotation Assignment
- Second Clinical Externship Rotation Assignment
- Discussion and Analysis of Clinical Externship
- Evaluation Process
- Mid-rotation evaluation
- Final rotation evaluation
Effective Term: Full Academic Year 2018/19 |
|
-
DAE 169LC - Dental Assisting Procedures Clinical II 6 Credits, 24 Contact Hours 0 lecture periods 24 lab periods
This is the clinical portion of DAE 169 . Application and evaluation of skills acquired in a clinical environment. Includes specialty seminars and guest lecturers; rotations to specialty practices; first and second clinical externship rotation assignments; discussion and analysis of clinical externship; and evaluation process.
Prerequisite(s): DAE 159 , DAE 160 , DAE 161 , DAE 162 , DAE 162 , DAE 163 , DAE 163LC , DAE 164 , DAE 164LB , DAE 165 , and DAE 165LC . Corequisite(s): DAE 166 , DAE 167 , DAE 169 Information: Consent of program coordinator is required before enrolling in this course.
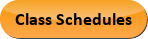
Course Learning Outcomes
- Demonstrate clinical experience and competency performing dental assisting functions.
Outline:
- Specialty Seminars and Guest Lecturers
- Orthodontist
- Oral surgeon
- Endodontist
- Pediatric dentist
- Prosthodontist
- TMD specialist
- Public health
- Periodontist
- Dental anesthesiologist
- Rotations to Specialty Practices
- First Clinical Externship Rotation Assignment
- Second Clinical Externship Rotation Assignment
- Discussion and Analysis of Clinical Externship
- Evaluation Process
- Mid-rotation evaluation
- Final rotation evaluation
Effective Term: Full Academic Year 2018/19 |
Dental Hygiene |
|
-
DHE 101 - Dental Hygiene I 2 Credits, 2 Contact Hours 2 lecture periods 0 lab periods
Introduction to the procedures used in the pre-clinical practice of dental hygiene at the beginning level. Includes professionalism and ethics, infection control, body mechanics/ergonomics, evaluation of patient medical and dental history, and assessment data. Also includes instrumentation, laboratory practice of dental hygiene procedures on student partners (e.g. removal of soft deposits, fluorides, various clinical procedures), and awareness of diverse patient populations.
Prerequisite(s): BIO 205IN and CIS 104 /CSA 104 . Corequisite(s): DHE 101LC , DHE 104 , DHE 104LB , DHE 107 , DHE 112 , DHE 116 , DHE 116LC Information: Students must be admitted to the PCC Dental Hygiene program and obtain consent of the Dental Hygiene department before enrolling in this course.
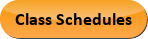
Course Learning Outcomes
- Demonstrate honest and ethical behavior in a professional setting.
- Perform infection control procedures consistent with the DHE program and Occupational Safety and Health Administration (OSHA) guidelines.
- Perform didactic and clinical skill evaluations, including proper instrumentation, successfully for assigned activities and entry level clinical skills assessment.
Performance Objectives:
- Explain “informed consent” and “standard of care” in a clinical setting.
- Identify methods to reduce risks within the practice of dental hygiene.
- Explain the process for maintaining confidentiality of patient records.
- Describe infection control procedures consistent with the DHE program and Occupational Safety and Health Administration (OSHA) guidelines.
- Discuss proper hand washing and gloving for clinic.
- Discuss patient positioning for optimal patient and operator comfort.
- Evaluate a medical/dental history and determine contraindications to dental treatment.
- Outline the rationale for pre-medication for the American Heart Association (AHA) regimen.
- Define the accepted ranges for temperature, blood pressure, pulse and respiration.
- Describe the procedures for hard and soft tissue examinations.
- Identify and document oral landmarks and abnormalities accurately.
- Discuss pen grasp, modified pen grasp, and palm-thumb grasp.
- Explain the use a fulcrum and finger-rest.
- Define the terms: long axis of the tooth, adaptation, insertion, vertical, horizontal, and oblique working strokes.
- Explain the “cutting edge” and its relationship to the angle formed between the tooth surface and the calculus removal instrument.
- Explain retraction, illumination, indirect vision, and using a dental mouth mirror.
- Explain a “walking stroke” when using a periodontal probe.
- Explain the use of a dental explorer utilizing short overlapping exploratory strokes.
- Describe the design, function and use technique of a universal curette, anterior and posterior sickle scaler and Gracey area specific curettes.
- Describe the function of Gracey curettes in root planning.
- Define the reasons for polishing coronal surfaces
- Define the term “selective polish.”
- Describe the application of a disclosing agent and evaluate for soft deposits.
- Explain the value of a fluoride treatment.
- Discuss the appropriate steps for a topical fluoride application.
- Complete a comprehensive dental hygiene treatment plan.
- Explain patient’s rights and responsibilities.
- Describe the process for recording services rendered in patient’s dental chart accurately.
- Integrate clinical experiences with didactic course work.
- Utilize a mock patient case study to develop didactic and clinical skill applications.
- Discuss the relevance of evidence-based decision making and patient care.
Outline:
- Professionalism/Ethics and Dental Hygiene Process of Care
- Apply professional code of ethics
- Assume responsibility for dental hygiene care based on accepted standard of care
- Perform self-assessment for professional growth
- Adhere to state and federal laws and regulations in the provision of dental hygiene care
- Infection Control
- Barrier protection
- Methods of sterilization
- Methods of disinfection
- Occupational Safety and Health Administration (OSHA) Standards and Centers for Disease Control (CDC) prevention guidelines
- Body Mechanics/Ergonomics
- Patient positioning
- Operator positioning
- Introduction to Patient Medical and Dental History Evaluation
- Obtain and interpret diagnostic information
- Predisposing etiologic risk factors
- Health conditions and medications that impact patient care
- Vital signs
- Assessment Data
- Extra and intraoral examination
- Periodontal charting
- Restorative charting
- Hard deposits
- Instrumentation
- Modified pen grasp, pen grasp, palm-thumb grasp
- Fulcrum and finger-rest
- Adaptation, insertion, angulation, working stroke
- Cutting edge relationship to tooth surface
- Mouth mirror
- Retraction, illumination, indirect vision
- Transillumination
- Periodontal probe-walking stroke
- Explorer-exploratory stroke
- Universal curette-working stroke
- Sickles
- Anterior
- Posterior
- Gracey curettes – root planing
- Removal of Soft Deposits – Coronal Polishing
- Selective polish
- Disclosing agents/plaque indices
- Fluorides
- Topical applications including fluoride varnish
- Assess value of fluoride treatment for dental hygiene care
- Clinical Procedures
- Comprehensive dental hygiene care plan
- Dental record documentation
- Consultations as indicated
- Patient needs and significant findings for delivery of care
- Informed consent
- Patient’s rights and responsibilities
- Respect Values and Preferences of Diverse Patient Population Groups While Promoting Oral Health
Effective Term: Full Academic Year 2021/2022 |
|
-
DHE 101LC - Dental Hygiene I Clinical 3 Credits, 12 Contact Hours 0 lecture periods 12 lab periods
This is the clinical lab portion of DHE 101 . Introduction to the procedures used in the pre-clinical practice of dental hygiene at the beginning level. Includes professionalism and ethics, infection control, body mechanics/ergonomics, evaluation of patient medical and dental history, and assessment data. Also includes instrumentation, laboratory practice of dental hygiene procedures on student partners (e.g. removal of soft deposits, fluorides, various clinical procedures), and awareness of diverse patient populations.
Corequisite(s): DHE 101 , DHE 104 , DHE 104LB , DHE 107 , DHE 112 , DHE 116 , DHE 116LC Information: Students must be admitted to the PCC Dental Hygiene program and obtain consent of the Dental Hygiene department before enrolling in this course.
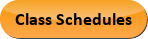
Course Learning Outcomes
- Demonstrate honest and ethical behavior in a professional setting.
- Perform infection control procedures consistent with the DHE program and Occupational Safety and Health Administration (OSHA) guidelines.
- Perform didactic and clinical skill evaluations, including proper instrumentation, successfully for assigned activities and entry level clinical skills assessment.
Performance Objectives:
- Explain “informed consent” and “standard of care” in a clinical setting.
- Identify methods to reduce risks within the practice of dental hygiene.
- Perform and maintain confidentiality of patient records.
- Perform infection control procedures consistent with the DHE program and Occupational Safety and Health Administration (OSHA) guidelines.
- Demonstrate proper hand washing and gloving for clinic.
- Prepare dental instruments for sterilization according to recognized Centers for Disease Control (CDC) and clinic protocols.
- Maintain aseptic environment when providing oral health care services to all patients to prevent transmission of blood borne pathogens.
- Demonstrate patient positioning for optimal patient and operator comfort.
- Evaluate a medical/dental history and determine contraindications to dental treatment.
- Outline the rationale for pre-medication for the American Heart Association (AHA) regimen.
- Evaluate and chart a medical/dental history on a student partner.
- Complete and record vital signs accurately on a student partner.
- Define the accepted ranges for temperature, blood pressure, pulse and respiration.
- Describe the procedures for hard and soft tissue examinations.
- Perform periodontal and restorative charting procedures on a student partner.
- Identify restorations on a tooth utilizing a shepherd’s hook explorer, radiographs and direct/indirect vision.
- Demonstrate pen grasp, modified pen grasp, and palm-thumb grasp.
- Explain and correctly use a fulcrum and finger-rest.
- Explain and identify the “cutting edge” and its relationship to the angle formed between the tooth surface and the calculus removal instrument.
- Explain and demonstrate retraction, illumination, indirect vision, and using a dental mouth mirror.
- Examine and position dental mouth mirror to demonstrate transillumination on a student partner.
- Explain and demonstrate a “walking stroke” when using a periodontal probe on a student partner.
- Demonstrate the use of a dental explorer utilizing short overlapping exploratory strokes.
- Describe the design of a universal curette.
- Demonstrate the correct technique for using universal curettes in all areas of the mouth on a student partner.
- Describe the design of an anterior sickle scaler and a posterior sickle scaler.
- Describe the correct technique for using sickle scalers on a student partner.
- Describe the function of Gracey curettes and root planing.
- Demonstrate the use of Gracey curettes utilizing a working stroke on a student partner.
- Define the reasons for polishing coronal surfaces and demonstrate the procedure on a student partner.
- Define the term “selective polish.”
- Demonstrate the application of a disclosing agent and evaluate for soft deposits.
- Explain the value of a fluoride treatment.
- Demonstrate the appropriate steps for a topical fluoride application on a student partner.
- Complete a comprehensive dental hygiene treatment plan.
- Explain patient’s rights and responsibilities.
- Record services rendered in patient’s dental chart accurately.
- Integrate laboratory experiences with didactic course work.
- Utilize a mock patient case study to develop didactic and clinical skill applications.
- Complete successfully skill evaluations for assigned laboratory activities.
- Perform entry level clinical procedures on a student partner at an acceptable passing level.
- Utilize a patient to develop didactic and entry level clinical assessment skills.
- Discuss the relevance of evidence-based decision making and patient care.
Outline:
- Professionalism/Ethics and Dental Hygiene Process of Care
- Apply professional code of ethics
- Assume responsibility for dental hygiene care based on accepted standard of care
- Perform self-assessment for professional growth
- Adhere to state and federal laws and regulations in the provision of dental hygiene care
- Infection Control
- Barrier protection
- Methods of sterilization
- Methods of disinfection
- Occupational Safety and Health Administration (OSHA) Standards and Centers for Disease Control (CDC) prevention guidelines
- Body Mechanics/Ergonomics
- Patient positioning
- Operator positioning
- Introduction to Patient Medical and Dental History Evaluation
- Obtain and interpret diagnostic information
- Predisposing etiologic risk factors
- Health conditions and medications that impact patient care
- Vital signs
- Assessment Data
- Extra and intraoral examination
- Periodontal charting
- Restorative charting
- Hard deposits
- Instrumentation
- Modified pen grasp, pen grasp, palm-thumb grasp
- Fulcrum and finger-rest
- Adaptation, insertion, angulation, working stroke
- Cutting edge relationship to tooth surface
- Mouth mirror
- Retraction, illumination, indirect vision
- Transillumination
- Periodontal probe-walking stroke
- Explorer-exploratory stroke
- Universal curette-working stroke
- Sickles
- Anterior
- Posterior
- Gracey curettes – root planing
- Removal of Soft Deposits – Coronal Polishing
- Selective polish
- Disclosing agents/plaque indices
- Fluorides
- Topical applications including fluoride varnish
- Assess value of fluoride treatment for dental hygiene care
- Clinical Procedures
- Comprehensive dental hygiene care plan
- Dental record documentation
- Consultations as indicated
- Patient needs and significant findings for delivery of care
- Informed consent
- Patient’s rights and responsibilities
- Respect Values and Preferences of Diverse Patient Population Groups While Promoting Oral Health
Effective Term: Full Academic Year 2021/2022 |
|
-
DHE 104 - Dental and Oral Morphology 1 Credits, 1 Contact Hours 1 lecture period 0 lab periods
Form and function of primary and permanent dentition. Includes oral cavity proper; form, function and physiology; and tooth identification. Also includes terminology, deciduous dentition morphology, occlusion, tooth anomalies, and root morphology.
Corequisite(s): DHE 101 , DHE 101LC , DHE 104LB , DHE 107 , DHE 112 , DHE 116 , DHE 116LC Information: Students must be admitted to the PCC Dental Hygiene program and obtain consent of the Dental Hygiene department before enrolling in this course.
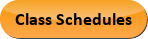
Course Learning Outcomes
- Describe and define the oral cavity proper, primary and adult dentitions, tooth surfaces, landmarks and anatomical features each tooth.
- Describe the process of tooth development, exfoliation, resorption and occlusion of primary and adult dentitions.
- Explain the relationships within the gingival unit, supporting structures of the teeth (periodontium) and tooth anomalies related to morphology.
Performance Objectives:
- Identify, name and code the teeth of the permanent dentition.
- Identify extracted or insitu tooth as to dentition, arch, and number.
- Describe and define the oral cavity proper.
- Identify the surfaces and landmarks of each tooth and explain the functions and location in the dental arches.
- Explain the difference between primary dentition, secondary dentition, and mixed dentition.
- Assess and code teeth using the Universal system of tooth identification.
- List the anatomical features of a tooth.
- Explain how the form of teeth relates to function.
- Name and code the teeth of the deciduous (primary) dentition.
- Describe the process of tooth development, exfoliation, and resorption.
- List the approximate ages of eruption for each primary and permanent tooth.
- List when crown and root are calcified for each tooth.
- Compare primary and permanent teeth.
- Define embrasures and identify their locations.
- Describe and define the term proximal contact and height of contour.
- Identify the centric relationship of the dental arches.
- Discuss the occlusion of deciduous and permanent teeth.
- Differentiate the various classifications of malocclusion.
- Explain the meaning of overjet, overbite, crossbite, and openbite.
- Identify anatomical landmarks related to the temporomandibular joint (TMJ).
- Describe the form and function of the temporomandibular joint.
- Discuss pulpal anatomy of primary and permanent teeth.
- Define intrinsic and extrinsic factors relative to dental anomalies.
- Describe the various classifications of tooth anomalies related to morphology.
- Define variations in root anomalies including concrescence, dilacerations, and hypercementosis.
- Distinguish abnormal crown and root formations.
- Explain the relationships within the gingival unit and supporting structures of the teeth (periodontium).
- State how clinical situations are related to tooth form and supportive dental structures.
- Compare and contrast the roots of maxillary and mandibular incisors in the permanent dentition.
- Describe the shapes of the roots of maxillary and mandibular permanent cuspids.
- Describe variances in occlusal anatomy among premolars.
- Describe the shapes of the roots of maxillary and mandibular permanent premolars.
- Describe each of the three permanent maxillary molars from all five aspects including the roots.
- Describe the three permanent mandibular molars from the five anatomical aspects including the roots.
- Explain the root location differences between maxillary and mandibular molars and why this is important.
- Identify anatomical root variations, cej curvature, furcations, developmental concavities for effective instrumentation adaptation.
- Relate root morphology to instrumentation applications.
- Demonstrate hand eye coordination by making an accurate drawing of assigned teeth.
- Practice carving to scale teeth out of wax using a lab knife and carving instruments.
- Use a Boley Gauge to measure a tooth carved out of wax.
- Apply didactic knowledge to computer related interactive case study.
Outline:
- Oral Cavity Proper
- Nomenclature/landmarks/anatomical features
- Permanent/secondary dentition
- Form, Function, and Physiology
- Maxillary/mandibular incisors
- Maxillary/mandibular canines
- Maxillary/mandibular premolars
- Maxillary/mandibular molars
- Dentition-Tooth Identification
- Permanent dentition – universal system
- Primary dentition – universal system
- Mixed dentition – universal system
- Landmarks – Terminology
- Surfaces of teeth
- Developmental fissures, cusps, grooves, pits
- Embrasures
- Height of contours
- Interproximal space
- Deciduous Dentition Morphology
- Eruption/exfoliation/resorption
- Eruption dates
- Development process
- Occlusion
- Angle’s classification system
- Malocclusion
- Centric relationship
- Temporomandibular joint (TMJ)
- Tooth Anomalies
- Extrinsic factors
- Intrinsic factors
- Root Morphology
- Distinguishing features
- Relationship to clinical instrumentation
- Root anomalies
- Concrescence
- Dilacerations
- Hypercementosis
- Root furcations
Effective Term: Fall 2016 |
|
-
DHE 104LB - Dental and Oral Morphology Lab 1 Credits, 3 Contact Hours 0 lecture periods 3 lab periods
This is the lab portion of DHE 104 . Form and function of primary and permanent dentition. Includes oral cavity proper; form, function and physiology; and tooth identification. Also includes terminology, deciduous dentition morphology, occlusion, tooth anomalies, and root morphology.
Corequisite(s): DHE 101 , DHE 101LC , DHE 104 , DHE 107 , DHE 112 , DHE 116 , DHE 116LC Information: Students must be admitted to the PCC Dental Hygiene program and obtain consent of the Dental Hygiene department before enrolling in this course.
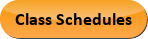
Course Learning Outcomes
- Describe and define the oral cavity proper, primary and adult dentitions, tooth surfaces, landmarks and anatomical features each tooth.
- Describe the process of tooth development, exfoliation, resorption and occlusion of primary and adult dentitions.
- Explain the relationships within the gingival unit, supporting structures of the teeth (periodontium) and tooth anomalies related to morphology.
Performance Objectives:
- Identify, name and code the teeth of the permanent dentition.
- Identify extracted or insitu tooth as to dentition, arch, and number.
- Describe and define the oral cavity proper.
- Identify the surfaces and landmarks of each tooth and explain the functions and location in the dental arches.
- Explain the difference between primary dentition, secondary dentition, and mixed dentition.
- Assess and code teeth using the Universal system of tooth identification.
- List the anatomical features of a tooth.
- Explain how the form of teeth relates to function.
- Name and code the teeth of the deciduous (primary) dentition.
- Describe the process of tooth development, exfoliation, and resorption.
- List the approximate ages of eruption for each primary and permanent tooth.
- List when crown and root are calcified for each tooth.
- Compare primary and permanent teeth.
- Define embrasures and identify their locations.
- Describe and define the term proximal contact and height of contour.
- Identify the centric relationship of the dental arches.
- Discuss the occlusion of deciduous and permanent teeth.
- Differentiate the various classifications of malocclusion.
- Explain the meaning of overjet, overbite, crossbite, and openbite.
- Identify anatomical landmarks related to the temporomandibular joint (TMJ).
- Describe the form and function of the temporomandibular joint.
- Discuss pulpal anatomy of primary and permanent teeth.
- Define intrinsic and extrinsic factors relative to dental anomalies.
- Describe the various classifications of tooth anomalies related to morphology.
- Define variations in root anomalies including concrescence, dilacerations, and hypercementosis.
- Distinguish abnormal crown and root formations.
- Explain the relationships within the gingival unit and supporting structures of the teeth (periodontium).
- State how clinical situations are related to tooth form and supportive dental structures.
- Compare and contrast the roots of maxillary and mandibular incisors in the permanent dentition.
- Describe the shapes of the roots of maxillary and mandibular permanent cuspids.
- Describe variances in occlusal anatomy among premolars.
- Describe the shapes of the roots of maxillary and mandibular permanent premolars.
- Describe each of the three permanent maxillary molars from all five aspects including the roots.
- Describe the three permanent mandibular molars from the five anatomical aspects including the roots.
- Explain the root location differences between maxillary and mandibular molars and why this is important.
- Identify anatomical root variations, cej curvature, furcations, developmental concavities for effective instrumentation adaptation.
- Relate root morphology to instrumentation applications.
- Demonstrate hand eye coordination by making an accurate drawing of assigned teeth.
- Practice carving to scale teeth out of wax using a lab knife and carving instruments.
- Use a Boley Gauge to measure a tooth carved out of wax.
- Apply didactic knowledge to computer related interactive case study.
Outline:
- Oral Cavity Proper
- Nomenclature/landmarks/anatomical features
- Permanent/secondary dentition
- Form, Function, and Physiology
- Maxillary/mandibular incisors
- Maxillary/mandibular canines
- Maxillary/mandibular premolars
- Maxillary/mandibular molars
- Dentition-Tooth Identification
- Permanent dentition – universal system
- Primary dentition – universal system
- Mixed dentition – universal system
- Landmarks – Terminology
- Surfaces of teeth
- Developmental fissures, cusps, grooves, pits
- Embrasures
- Height of contours
- Interproximal space
- Deciduous Dentition Morphology
- Eruption/exfoliation/resorption
- Eruption dates
- Development process
- Occlusion
- Angle’s classification system
- Malocclusion
- Centric relationship
- Temporomandibular joint (TMJ)
- Tooth Anomalies
- Extrinsic factors
- Intrinsic factors
- Root Morphology
- Distinguishing features
- Relationship to clinical instrumentation
- Root anomalies
- Concrescence
- Dilacerations
- Hypercementosis
- Root furcations
Effective Term: Fall 2016 |
|
-
DHE 107 - Oral Embryology and Histology 2 Credits, 2 Contact Hours 2 lecture periods 0 lab periods
The development and histology of teeth related to the intra and extra oral tissues of the head as they relate to the practice of dental hygiene. Includes terminology and formation of primary embryonic layers, histology, tooth development, enamel, dentin, and pulp. Also includes cementum, periodontal ligament, bone and alveolar process, mucous membranes, and salivary glands.
Corequisite(s): DHE 101 , DHE 101LC , DHE 104 , DHE 104LB , DHE 112 , DHE 116 , DHE 116LC Information: Students must be admitted to the PCC Dental Hygiene program and obtain consent of the Dental Hygiene department before enrolling in this course.
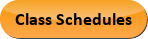
Course Learning Outcomes
- Identify normal growth and development of the face and oral structures at the cellular level by distinguishing enamel, dentine, and pulp at the microscopic and macroscopic level.
- Describe the processes of tooth development in utero which correlates to growth and development of oral structures.
- Identify bones, nerves, lymphatic or blood vessels by location and muscles and their relationship to occlusion and mastication.
Performance Objectives:
- Describe the processes which occur at the cellular level in the growth and development or oral structures.
- Differentiate among enamel, dentin, and pulp at the microscopic and macroscopic level.
- Describe the function and characteristics of anatomical structures the head and neck.
- Explain the muscles and their relationship to occlusion and mastication.
- Identify bones, nerves, lymphatic or blood vessels by location.
- Determine injection sites by identifying nerve innervations of individual teeth.
- Identify normal growth and development of the face and oral structures.
Outline:
- Terminology and Formation of Primary Embryonic Layers
- Development of the face and oral cavity
- Formation of maxilla and mandible
- Introduction / Definitions of Histology
- Tissue content
- Tissue classification
- Tooth Development, Eruption and Exfoliation
- Early tooth development
- Tooth germ
- Eruption
- Root, cementum, periodontal ligament formation
- Exfoliation
- Enamel
- Amelogenesis
- Composition
- Structure and clinical appearance
- Dentin/Dentinogenesis
- Odontoblasts
- Coronal dentin/root dentin
- Clinical dentin
- Pulp
- Composition
- Function
- Clinical
- Cementum
- Cementogenesis
- Structure and composition
- Periodontal Ligament
- Location and composition
- Principle fibers
- Function
- Bone and Alveolar Process
- Structure and composition of bone
- Bone growth
- Formation
- Resorption
- Remodeling
- Aveolar process
- Mucous Membrane and Salivary Glands
- Mucous membranes of the oral cavity
- Tongue
- Salivary glands
Effective Term: Fall 2016 |
|
-
DHE 112 - Preventive Dentistry 3 Credits, 3 Contact Hours 3 lecture periods 0 lab periods
Introduction to dental disease and the promotion of dental health. Includes the role of dental hygienists as prevention specialists, clinical treatment theories, patient care readiness, dental disease, risk assessment, and oral hygiene instruction. Also includes dentin sensitivity, enamel demineralization and remineralization, chemotherapeutics, and tobacco cessation.
Corequisite(s): DHE 101 , DHE 101LC , DHE 104 , DHE 104LB , DHE 107 , DHE 116 , DHE 116LC Information: Students must be admitted to the PCC Dental Hygiene program and obtain consent of the Dental Hygiene department before enrolling in this course.
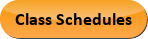
Course Learning Outcomes
- Outline preventive measures to ensure safe, effective, and legally sound dental hygiene care.
- Summarize the dental hygiene models to include evidence-based decision making and the dental hygiene process of care model.
- Explain the theory of minimally invasive dentistry, components of caries risk assessment, and how the two compliment conservative restorative care.
- Identify local and systemic oral hygiene factors, patient motivation techniques, and oral physiotherapy techniques.
Performance Objectives:
- Summarize the dental hygiene models to include evidence-based decision making and the dental hygiene process of care model.
- Identify critical components of patient health histories as they apply to treatment planning.
- Describe the etiologies of dental diseases and the associated treatment regimens.
- Explain the theory of minimally invasive dentistry, components of caries risk assessment, and how the two compliment conservative restorative care.
- Identify local and systemic oral hygiene factors, patient motivation techniques, and oral physiotherapy techniques.
- Summarize the etiology of dentinal sensitivity and current products and techniques used to treat the condition.
- Explain the process of enamel demineralization and the theories and products associated with treatment.
- Describe the strengths and limitations of chemicotherapy and the types of treatment applications commonly utilized.
- Outline the psychotherapies associated with tobacco cessation and the local and national resources for patient and clinician support.
Outline:
- Role of Dental Hygienists as Prevention Specialists
- Medical risk management
- Legal risk management
- Clinical Treatment Theories
- Evidence-based decision making
- Minimally invasive dentistry
- Dental hygiene process care model
- Patient Care Readiness
- Critical health histories
- Oral cancer screening technology
- Dental Disease – Etiology and Treatment
- Caries Risk Assessment
- Methamphetamine addiction
- Prevalence
- Oral manifestations
- Oral piercing care
- Oral Hygiene Instruction, Reinforcement, and Motivation
- Oral hygiene aids
- Patient communication and motivation
- Removable appliance care and maintenance
- Dentin Sensitivity
- Xerostomia – patient management
- O’Leary’s plaque index
- Demineralization/Remineralization
- Fluoride therapies
- Sealants
- Chemotherapeutics
- Oral irrigation
- Dentifrices
- Mouthwashes
- Oral hygiene instruction
- Tobacco Management/Tobacco Cessation
Effective Term: Fall 2016 |
|
-
DHE 116 - Oral Radiography 2 Credits, 2 Contact Hours 2 lecture periods 0 lab periods
Principles of dental radiography as a diagnostic aid. Includes radiation production and biology. Also includes clinic experience in exposing, processing, mounting, and interpreting radiographs on mannequins and patients using a variety of radiographic techniques.
Corequisite(s): DHE 101 , DHE 101LC , DHE 104 , DHE 104LB , DHE 107 , DHE 112 , DHE 116LC Information: Students must be admitted to the PCC Dental Hygiene program and obtain consent of the Dental Hygiene department before enrolling in this course. DAE 163 can be substituted for DHE 116 if the course meets the CODA-approved Advanced Standing standard and was completed within the last three years. Please see an academic advisor or faculty for information regarding course substitution. To substitute DAE 163 for DHE 116, student must have current and active CDA certification.
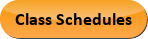
Course Learning Outcomes
- Demonstrate competency in the knowledge and skills required to systematically collect diagnostic data
- Define and explain state and federal dental laws and regulations
- Demonstrate competency in the knowledge and skills required to manage infections hazard control protocol consistent with published professional guidelines
- Demonstrate competency in the knowledge and skills in dental radiology
- Demonstrate competency in interpreting normal and abnormal anatomy and conditions
Performance Objectives:
- Identify the important people and events in the history of radiography.
- State the principles of radiation production.
- Identify and demonstrate ALARA methods of radiation production for the patient and operator.
- Operate and maintain x-ray units safely.
- Identify radiographic films as to size and use.
- Process radiographic films manually.
- Practice quality assurance procedures.
- Identify radiographic anatomical landmarks.
- Perform radiograph mounting.
- Interpret normal and abnormal pathological condition visible on dental radiographs.
- Describe and perform radiographic film exposure techniques.
- Describe and perform digital radiography exposure techniques.
- Identify and correct undiagnostic radiographs.
- Evaluate radiographic quality.
- Complete a variety of radiographs on mannequin and patients.
- Identify and perform infection control precautions as specified by the Occupational Safety and Health Administration (OSHA) and program policy.
Outline:
- Introduction to Radiography
- History of radiography
- Production and characteristics of x-radiation
- Dental x-ray equipment
- Components
- Parts
- Inverse Square Law
- Radiation Health and Safety
- Radiation safety legislation
- Radiation measurement terminology
- Measuring and monitoring devices
- Protection measures for the patient
- Radiation protection for operators
- Maximum permissible doses
- Dental Film
- Film emulsion and speeds
- Packaging, storage, and protection of film
- Types of intraoral and extraoral films
- Handling cassettes
- Film Processing
- Darkroom
- Equipment
- Illumination
- Maintenance
- Processing solutions and procedures
- Inadequacies caused by faulty processing techniques
- Film duplication
- Xeroradiography
- Digital Radiography
- Identification of Anatomical Landmarks for Mounting and Interpretation
- Radiolucent and radiopaque images
- Alveolar bone and supporting structures
- Landmarks identified
- Mounting, labeling, filing, and storage of radiographs
- Film viewing and preliminary radiographic interpretation
- Abnormal and normal pathological conditions
- Exposure Techniques
- Criteria for intraoral radiographs
- Horizontal and vertical angulation
- Principles of bisecting and paralleling techniques
- The periapical examination
- Fundamentals of interproximal radiography
- Radiography for children
- Patient management
- Infection control
- Evaluation of radiographic quality
- Digital radiography
- Principles of Supplementary Film
- Occlusal surveys
- Types of use of extraoral film
- Lateral jaw survey
- Temporomandibular articulation survey
- Cephalometric radiography
- Panoramic radiography
- Other imaging systems
Effective Term: Fall 2022 |
|
-
DHE 116LC - Oral Radiography Clinical 1 Credits, 4 Contact Hours 0 lecture periods 4 lab periods
This is the clinical lab portion of DHE 116 . Principles of dental radiography as a diagnostic aid. Includes radiation production and biology. Also includes clinic experience in exposing, processing, mounting, and interpreting radiographs on mannequins and patients using a variety of radiographic techniques.
Corequisite(s): DHE 101 , DHE 101LC , DHE 104 , DHE 104LB , DHE 107 , DHE 112 , DHE 116 Information: Students must be admitted to the PCC Dental Hygiene program and obtain the consent of the Dental Hygiene department before enrolling in this course. DAE 163LC can be substituted for DHE 116LC if the course meets the CODA-approved Advanced Standing standard and was completed within the last three years. Please see an academic advisor or faculty for information regarding course substitution. To substitute DAE 163LC for DHE 116LC, the student must have current and active CDA certification.
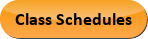
Course Learning Outcomes
- Demonstrate competency in the knowledge and skills required to systematically collect diagnostic data
- Define and explain state and federal dental laws and regulations
- Demonstrate competency in the knowledge and skills required to manage infections hazard control protocol consistent with published professional guidelines
- Demonstrate competency in the knowledge and skills in dental radiology
- Demonstrate competency in interpreting normal and abnormal anatomy and conditions
Performance Objectives:
- Identify the important people and events in the history of radiography.
- State the principles of radiation production.
- Identify and demonstrate ALARA methods of radiation production for the patient and operator.
- Operate and maintain x-ray units safely.
- Identify radiographic films as to size and use.
- Process radiographic films manually.
- Practice quality assurance procedures.
- Identify radiographic anatomical landmarks.
- Perform radiograph mounting.
- Interpret normal and abnormal pathological condition visible on dental radiographs.
- Describe and perform radiographic film exposure techniques.
- Describe and perform digital radiography exposure techniques.
- Identify and correct undiagnostic radiographs.
- Evaluate radiographic quality.
- Complete a variety of radiographs on mannequin and patients.
- Identify and perform infection control precautions as specified by the Occupational Safety and Health Administration (OSHA) and program policy.
Outline:
- Introduction to Radiography
- History of radiography
- Production and characteristics of x-radiation
- Dental x-ray equipment
- Components
- Parts
- Inverse Square Law
- Radiation Health and Safety
- Radiation safety legislation
- Radiation measurement terminology
- Measuring and monitoring devices
- Protection measures for the patient
- Radiation protection for operators
- Maximum permissible doses
- Dental Film
- Film emulsion and speeds
- Packaging, storage, and protection of film
- Types of intraoral and extraoral films
- Handling cassettes
- Film Processing
- Darkroom
- Equipment
- Illumination
- Maintenance
- Processing solutions and procedures
- Inadequacies caused by faulty processing techniques
- Film duplication
- Xeroradiography
- Digital Radiography
- Identification of Anatomical Landmarks for Mounting and Interpretation
- Radiolucent and radiopaque images
- Alveolar bone and supporting structures
- Landmarks identified
- Mounting, labeling, filing, and storage of radiographs
- Film viewing and preliminary radiographic interpretation
- Abnormal and normal pathological conditions
- Exposure Techniques
- Criteria for intraoral radiographs
- Horizontal and vertical angulation
- Principles of bisecting and paralleling techniques
- The periapical examination
- Fundamentals of interproximal radiography
- Radiography for children
- Patient management
- Infection control
- Evaluation of radiographic quality
- Digital radiography
- Principles of Supplementary Film
- Occlusal surveys
- Types of use of extraoral film
- Lateral jaw survey
- Temporomandibular articulation survey
- Cephalometric radiography
- Panoramic radiography
- Other imaging systems
Effective Term: Fall 2022 |
|
-
DHE 119 - Periodontology 2 Credits, 2 Contact Hours 2 lecture periods 0 lab periods
Survey of periodontology comprised of the etiology, diagnosis, and prognosis of periodontal disease. Includes tissues and microscopic anatomy of the periodontium, historical background, causes, microbiology and classification of periodontal disease, local and systemic contributing factors, clinical assessment, radiographic analysis, and evidence-based periodontal care. Also includes decision making during treatment planning, nonsurgical and patient’s role in periodontal therapy, maintenance therapy, research articles and applications, and new dental technology.
Prerequisite(s): DHE 101 , DHE 101LC , DHE 104 , DHE 104LB , DHE 107 , DHE 112 , DHE 116 , and DHE 116LC Corequisite(s): DHE 120 , DHE 122 , DHE 132 , DHE 132LB , DHE 150 , DHE 150LB , DHE 150LC Information: Students must be admitted to the PCC Dental Hygiene program and obtain consent of the Dental Hygiene department before enrolling in this course.
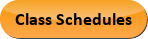
Course Learning Outcomes
- Identify structures of the periodontium.
- List and define the microscopic anatomy of the periodontium.
- Describe progression of periodontal disease.
- Identify periodontal conditions on radiographic imagery.
- Describe steps in nonsurgical periodontal therapy.
- Explain basic components and use of lasers.
- Describe the relationship of oral biofilms and periodontal disease.
- Describe the host immune response to periodontal pathogens.
- Classify periodontal diseases.
- List and describe systemic factors associated with periodontal disease.
- Describe the clinical periodontal assessment.
- Identify local contributing factors for periodontal disease.
Outline:
- Tissues of the Periodontium
- Nerve supply
- Blood supply
- Lymphatic system
- Microscopic Anatomy of the Periodontium
- Histology of body tissues
- Histology of the gingiva
- Histology of root cementum and alveolar bone
- The History of Periodontal Disease
- The periodontium in health and disease
- Classifications systems
- AAP classification for periodontal diseases
- Search for the Causes of Periodontal Disease
- Epidemiology: researching periodontal disease
- Control and progression of periodontal disease
- Risk factors for periodontal disease
- Microbiology of Periodontal Disease
- Bacteria in the oral environment
- Bacteria associated with periodontal health and disease
- Structure and colonization of plaque biofilms
- Mechanisms of periodontal destruction
- Control of plaque biofilms
- Host Immune Response
- Role of host response in periodontal disease
- Pathogenesis of inflammatory periodontal disease
- Local Contributing Factors
- Local factors that increase plaque biofilm retention
- Local factors that increase plaque biofilm pathogenicity
- Local factors that cause direct damage
- Systemic Contributing Factors
- Systemic risk factors for periodontitis
- Genetic risk factors for periodontitis
- Systemic medications with periodontal side effects
- Classification of Periodontal Diseases and Conditions
- Epidemiology: researching periodontal disease
- Control and progression of periodontal disease
- Risk factors for periodontal disease
- Gingival Disease
- Classification of gingival diseases
- Dental-plaque induced gingival diseases
- Non-plaque-induced gingival
- Lesions
- Periodontitis
- Chronic periodontitis
- Aggressive periodontitis
- Periodontitis as a manifestation of systemic disease
- Necrotizing periodontal diseases
- Developmental or acquired deformities and conditions
- Clinical Periodontal Assessment
- Periodontal screening examination
- Comprehensive periodontal assessment
- Clinical features that require calculations
- Radiographic Analysis of the Periodontium
- Radiographic appearance of the periodontium
- Use of radiographs for periodontal evaluation
- Evidence-Based Periodontal Care
- Best practice
- Role of evidence-based care in best practice
- Finding clinically relevant information
- Lifelong learning skills for best practice
- Decision Making During Treatment Planning
- Decisions related to assigning a periodontal diagnosis
- Decisions related to treatment sequencing
- Informed consent for periodontal treatment
- Nonsurgical Periodontal Therapy
- Nonsurgical instrumentation
- Decisions following nonsurgical therapy
- Patient’s Role in Nonsurgical Periodontal Therapy
- Patient self-care
- Tooth brushing and tongue cleaning
- Interdental care
- Periodontal Maintenance Therapy
- Procedures and planning for periodontal maintenance
- Disease recurrence and patient compliance
- Root caries as a complication during periodontal maintenance
- Case Studies and Research Articles and Applications
- Comprehensive patient cases
- Guidelines for reading dental literature
- Procedures for searching the Internet
- Periodontal resources on the Internet
- New Dental Technology
Introduction
Effective Term: Fall 2016 |
|
-
DHE 120 - Oral Pathology 2 Credits, 2 Contact Hours 2 lecture periods 0 lab periods
Overview of oral pathology which is the study of human disease as found within all of the tissues represented in the area of the oral cavity. Includes introduction to pathology, diagnostic methods, normal exam and variants of normal, inflammation and repair, physical/chemical injuries of the oral tissues, and immunity and autoimmune diseases. Also includes infectious diseases, developmental disorders, neoplasia, genetic disorders, and oral manifestations of systemic disease.
Prerequisite(s): DHE 101 /DHE 101LC , DHE 104 /DHE 104LB , DHE 107 , DHE 112 , and DHE 116 /DHE 116LC Corequisite(s): DHE 119 , DHE 122 , DHE 132 , DHE 132LB , DHE 150LB , DHE 150 , DHE 150LB Information: Students must be admitted to the PCC Dental Hygiene program and obtain consent of the Dental Hygiene department before enrolling in this course.
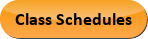
Course Learning Outcomes
- Compare and contrast normal from abnormal.
- Summarize the process of inflammation and repair.
- Describe clinical and radiographic abnormalities found during an oral examination.
- Explain the etiology, pathogenesis, signs, symptoms, clinical characteristics, radiographic finds, pathologic features, appropriate treatment, and prognosis of infectious diseases covered in the course.
- Identify high risk areas, early manifestations, signs, symptoms, growth characteristics, histopathologic features, radiographic findings, prognosis, modalities of treatment, sequelae of therapy, and predisposing factors of oral squamous cell carcinoma.
- Outline basic diagnostic procedures to provide additional information or a definitive diagnosis for oral lesions.
- Explain epidemiology and available preventative measures for those diseases representing significant public health problems.
- Apply the nomenclature to the diseases discussed in order to communicate effectively with dental and medical colleagues.
- Describe the limitations of her/his diagnostic abilities and be capable of intelligent referral of patients to specialists.
Outline:
- Introduction to Pathology
- Diagnostic Methods, Clinical Exam
- Normal Exam/Variants of Normal
- Inflammation and Repair
- Physical/Chemical Injuries of the Oral Tissues
- Immunity and Autoimmune Diseases
- Infectious Diseases
- Developmental Disorders
- Neoplasia
- Squamous cell cancer
- Soft tissue
- Hard tissue
- Genetic Disorders
- Oral Manifestations of Systemic Diseases
Effective Term: Fall 2016 |
|
-
DHE 122 - Pharmacology 2 Credits, 2 Contact Hours 2 lecture periods 0 lab periods
Introduction to the theory of pharmacology as it relates to dentistry. Includes drug action and handling, prescription writing, autonomic drugs, non-opioid analgesics, anti-infective agents, anti-fungal and anti-viral agents, anti-anxiety agents, cardiovascular agents, and anti-convulsant agents. Also includes psychotherapeutic agents, antacids and antihistamines, adreno cortico steroid agents, anti-neoplastic agents, and respiratory and gastrointestinal medications, emergency medications, and drug interactions and drug abuse.
Prerequisite(s): DHE 101 , DHE 101LC , DHE 104 , DHE 104LB , DHE 107 , DHE 112 , DHE 116 , and DHE 116LC Corequisite(s): DHE 119 , DHE 120 ,DHE 132 , DHE 132LB , DHE 150 , DHE 150LB , DHE 150LC Information: Students must be admitted to the PCC Dental Hygiene program and obtain consent of the Dental Hygiene department before enrolling in this course.
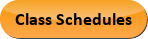
Course Learning Outcomes
- Describe the basic principles of pharmacology, the sources of drug production, and the agencies who regulate drug dispensaries.
- Analyze commonly prescribe dental drugs including therapeutic use, mechanism of action, pharmacokinetics, pharmacologic effects, adverse reactions, contrainindications, and patient education.
- Demonstrate the components of prescription writing.
- Describe the pharmacokinetics, conditions, and applications of analgesics in dental hygiene care.
- Compare and contrast the affinity, potency, and efficacy of the following drug classes: autonomic drugs; anti-infective agents; cardiovascular agents; psychotherapeutic agents; hormones, anti-neoplastic agents; respiratory and gastrointestinal medications; anti-anxiety agents; and emergency medications.
Outline:
- Introduction, Sources, and Regulatory Agencies
- Terminology
- Printed sources
- Computer sources
- Online sources
- Regulation and classification of drugs
- Labeling requirements
- Black box warning
- Drug Action and Handling and Adverse Reactions
- Routes of drug administration
- Pharmacodynamics
- Drug effects
- Drug interactions
- Bioequivalence and bioavailability
- Prescription Writing
- Goals of prescription writing
- Units of measurement
- Latin abbreviations
- Safety of prescription pads
- Patient adherence
- Reduction of medication errors
- Prescribing for children
- Safety in pregnancy
- Autonomic Drugs
- Nervous system
- Neurotransmitters
- Sympathomimetic drugs
- Adrenergic agonists
- Adrenergic receptor antagonists
- transmission
- Non-Opioid Analgesics
- Neurophysiology of pain
- Drug therapy for dental pain
- Non-narcotic analgesics
- Non-steroidal anti-inflammation drugs
- Opioid analgesics
- Substance abuse and dependency
- Anti-Infective Agents
- Antimicrobial agents
- Bactericidal antibiotics
- Bacteriostatic antibiotics
- Miscellaneous antibiotics
- Prevention of infective endocarditis
- Antibacterial agents: topical
- Controlled-release drug delivery
- Anti-Fungal and Anti-Viral Agents
- Herpes simplex
- Antiretroviral agents
- Antifungal agents
- Anti-Anxiety Agents
- Benzodiazepines
- Barbiturates
- Nonbarbiturates
- Nitrous oxide
- Narcotics
- General anesthesia
- Cardiovascular Agents
- Hypertension
- Angina pectoris
- Heart failure
- Arrythmias
- Anti-Convulsant Agents
- Psychotherapeutic Agents
- Antipsychotic drugs
- Mood disorders
- Anxiolytics
- Sedative/hypnotic drugs
- Antacids and Antihistamines
- Adreno Cortico Steroid Agents
- Other Hormones
- Anti-Neoplastic and Immunosuppressant Agents
- Actions
- Treatment
- Adverse side effects
- Limitations to dental treatment
- Chemotherapy
-
- Respiratory and Gastrointestinal Medications
- Lung anatomy
- Asthma
- Cold
- Cough
- Peptic ulcer
- Irritable bowel syndrome
- Nausea and vomiting
- Constipation
- Diarrhea
- Inflammatory bowel disease
- Emergency Medications
- Preparation of dental staff
- Basic life support
- Emergency medical kit and equipment
- Drug Interactions and Drug Abuse
Effective Term: Fall 2016 |
|
-
DHE 132 - Dental Materials 2 Credits, 2 Contact Hours 2 lecture periods 0 lab periods
Chemical and physical properties of dental materials used in dental practice. Includes introduction to dental materials, preventive sealants and restorative materials, dental cements. Also includes impression materials, gypsum products, miscellaneous dental materials; and gold, non-precious alloys, and casting of metals.
Prerequisite(s): DHE 101 , DHE 101LC , DHE 104 , DHE 104LB , DHE 107 , DHE 112 , DHE 116 , and DHE 116LC . Corequisite(s): DHE 119 , DHE 120 , DHE 122 , DHE 132LB , DHE 150 , DHE 150LB , DHE 150LC Information: Students must be admitted to the PCC Dental Hygiene program and obtain the consent of the Dental Hygiene department before enrolling in this course. DAE 164 can be substituted for DHE 132 if the course meets the CODA-approved Advanced Standing standard and was completed within the last three years. Please see an academic advisor or faculty for information regarding course substitution. To substitute DAE 164 for DHE 132, the student must have current and active CDA certification.
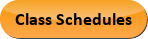
Course Learning Outcomes
- Demonstrate proper use of Personal Protective Equipment (PPE), Occupational Safety and Health Administration (OSHA) standards, the identification of Globally Harmonized System (GHS), and manufacturer’s’ Safety Data Sheets (SDS).
- Define and explain state and federal dental laws and regulations.
- Compare and contrast different dental restorative materials and their biocompatibility significance.
- Explain the importance of the study of dental materials.
- Identify, synthesize, and evaluate dental materials in radiographic imagery.
- Demonstrate effective communication skills using verbal, non-verbal, and written forms.
- Demonstrate knowledge of properties and manipulation of dental materials.
- Demonstrate at competency level the use of various types of dental materials.
Performance Objectives:
- Identify the properties of matter and materials.
- Operate and maintain laboratory equipment safely.
- Prepare dental waxes, cements, preventive sealants, and restorative materials.
- Prepare materials to make occlusal registration for articulating study casts.
- Prepare and pour elastic, plastic, and rigid impression materials.
- Prepare and pour study casts using gypsum products.
- Fabricate trays, e.g. custom trays, mouth guard, and whitening trays.
- Fabricate provisional restorations.
- Describe casting techniques and identify metals used in the fabrication of dental prostheses.
- Prepare, place, and finish composite and amalgam restorations.
Outline:
- Introduction to Dental Materials
- American Dental Association (ADA) specifications
- Proper use of PPE with guidance by the Center of Disease Control (CDC)
- Properties of matter
- Physical and chemical properties required for dental materials
- Precautions
- Handling materials to the standards of Environmental Protection Agency (EPA) and Pima’s Environmental Health and Safety (EHS)
- Hazardous substances as labeled by Globally Harmonized System (GHS)
- Occupational Safety and Health Administration (OSHA) regulations
- Safe use of laboratory equipment
- Arizona State dental statutes and regulations for auxiliary practitioners
- Preventive Sealants and Restorative Materials
- Types of preventive sealants
- Indications for use
- Properties, advantages, and disadvantages
- Manipulation
- Direct filling materials and armamentarium
- Esthetic/ Resins
- Amalgam
- Finish and polish esthetic and amalgam restorations
- Matrices
- Rubber dam
- Dental Cements
- Temporary and permanent cements and cement bases
- Composition and uses of various types
- Manipulation of cements
- Cavity liners and varnishes
- Special applications of cements
- Impression Materials
- Types of impression materials
- Types of impression trays
- Custom, mouth guard, and whitening trays
- Agar hydrocolloid (reversible hydrocolloid)
- Rubber impression materials and elastomers
- Alginate
- Bite registration
- Disinfection of impressions
- Gypsum Products
- Classification of uses of gypsum products
- Composition and manufacture
- Variables
- Manipulation
- Construction of model, cast, or die
- Modeling trimming
- Articulation
- Miscellaneous Dental Materials
- Uses of resins and acrylic
- Waxes
- Dental ceramics and porcelain
- Polishing and cleaning agents
- Periodontal dressings
- Provisional restorations
- Gold, Non-Precious Alloys, and Casting of Metals
- Gold foil
- Gold casting alloys
- Wrought metals
- Dental implant materials
Effective Term: Fall 2022 |
|
-
DHE 132LB - Dental Materials Lab 1 Credits, 3 Contact Hours 0 lecture periods 3 lab periods
This is the lab portion of DHE 132 . Chemical and physical properties of dental materials used in dental practice. Includes introduction to dental materials, preventive sealants and restorative materials, dental cements. Also includes impression materials, gypsum products, miscellaneous dental materials; and gold, non-previous alloys, and casting of metals.
Prerequisite(s): DHE 101 , DHE 101LC , DHE 104 , DHE 104LB , DHE 107 , DHE 112 , DHE 116 , DHE 116LC Corequisite(s): DHE 119 , DHE 120 , DHE 122 , DHE 132 , DHE 150 , DHE 150LB , DHE 150LC Information: Students must be admitted to the PCC Dental Hygiene program and obtain the consent of the Dental Hygiene department before enrolling in this course. DAE 164LB can be substituted for DHE 132LB if the course meets the CODA-approved Advanced Standing standard and was completed within the last three years. Please see an academic advisor or faculty for information regarding course substitution. To substitute DAE 164LB for DHE 132LB, the student must also hold a current and active CDA certificate.
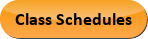
Course Learning Outcomes
- Demonstrate proper use of Personal Protective Equipment (PPE), Occupational Safety and Health Administration (OSHA) standards, the identification of Globally Harmonized System (GHS), and manufacturer’s Safety Data Sheets (SDS).
- Define and explain state and federal dental laws and regulations.
- Compare and contrast different dental restorative materials and their biocompatibility significance.
- Explain the importance of the study of dental materials.
- Identify, synthesize, and evaluate dental materials in radiographic imagery.
- Demonstrate effective communication skills using verbal, non-verbal, and written forms.
- Demonstrate knowledge of properties and manipulation of dental materials.
- Demonstrate at competency level the use of various types of dental materials.
Performance Objectives:
- Identify the properties of matter and materials.
- Operate and maintain laboratory equipment safely.
- Prepare dental waxes, cements, preventive sealants, and restorative materials.
- Prepare materials to make occlusal registration for articulating study casts.
- Prepare and pour elastic, plastic, and rigid impression materials.
- Prepare and pour study casts using gypsum products.
- Fabricate trays, e.g. custom trays, mouth guard, and whitening trays.
- Fabricate provisional restorations.
- Describe casting techniques and identify metals used in the fabrication of dental prostheses.
- Prepare, place, and finish composite and amalgam restorations.
Outline:
- Introduction to Dental Materials
- American Dental Association (ADA) specifications
- Proper use of PPE with guidance by the Center of Disease Control (CDC)
- Properties of matter
- Physical and chemical properties required for dental materials
- Precautions
- Handling materials to the standards of Environmental Protection Agency (EPA) and Pima’s
-
Environmental Health and Safety (EHS)
-
-
- Hazardous substances as labeled by Globally Harmonized System (GHS)
- Occupational Safety and Health Administration (OSHA) regulations
- Safe use of laboratory equipment
- Arizona State dental statutes and regulations for auxiliary practitioners
- Preventive Sealants and Restorative Materials
- Types of preventive sealants
- Indications for use
- Properties, advantages, and disadvantages
- Manipulation
- Direct filling materials and armamentarium
- Esthetic/ Resins
- Amalgam
- Finish and polish esthetic and amalgam restorations
- Matrices
- Rubber dam
- Dental Cements
- Temporary and permanent cements and cement bases
- Composition and uses of various types
- Manipulation of cements
- Cavity liners and varnishes
- Special applications of cements
- Impression Materials
- Types of impression materials
- Types of impression trays
- Custom, mouth guard, and whitening trays
- Agar hydrocolloid (reversible hydrocolloid)
- Rubber impression materials and elastomers
- Alginate
- Bite registration
- Disinfection of impressions
- Gypsum Products
- Classification of uses of gypsum products
- Composition and manufacture
- Variables
- Manipulation
- Construction of model, cast, or die
- Modeling trimming
- Articulation
- Miscellaneous Dental Materials
- Uses of resins and acrylic
- Waxes
- Dental ceramics and porcelain
- Polishing and cleaning agents
- Periodontal dressings
- Provisional restorations
- Gold, Non-Precious Alloys, and Casting of Metals
- Gold foil
- Gold casting alloys
- Wrought metals
-
Dental implant materials
Effective Term: Fall 2022 |
|
-
DHE 150 - Dental Hygiene II 2 Credits, 2 Contact Hours 2 lecture periods 0 lab periods
Continuation of DHE 101 /DHE 101LC Application of dental hygiene skills with a variety of clinical patients with simple dental hygiene care plans. Includes instrument review, evidence-based decision making and treatment planning, medical emergency management review, special needs patients, powered instruments, air powder polishing and stain removal, care of dental prostheses, advanced instrumentation and alternate fulcrums, tobacco cessation, subgingival irrigation, and antimicrobials. Also includes dental implant instruments, case studies, table clinics, and laboratory procedures.
Prerequisite(s): DHE 101 , DHE 101LC , DHE 104 , DHE 104LB , DHE 107 , DHE 112 , and DHE 116LC . Corequisite(s): DHE 119 , DHE 120 , DHE 122 , DHE 132 , DHE 132LB , DHE 150LB , DHE 150LC Information: Students must be admitted to the PCC Dental Hygiene program and obtain consent of the Dental Hygiene department before enrolling in this course.
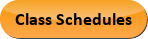
Course Learning Outcomes
- Demonstrate professional and ethical behavior in patient care.
- Apply asepsis protocol of recommended clinical guidelines for infection control and hazard management prior, during and after the provision of dental hygiene services.
- Demonstrate proper body mechanics techniques for optimal operator and patient comfort.
- Identify appropriate instruments for patients with varying dental needs.
- Assess patient’s needs for preventive, educational and therapeutic dental hygiene clinical services and interpret dental radiographs for dental disease.
- Describe evidence-based decision making principles and clinical reasoning to patient care plans.
- Integrate, synthesize, and evaluate oral health services of diverse patient population groups through community service learning activities.
- Apply knowledge of powered instruments, application of dental sealants, practice of medical emergency procedures, and computer applications and record documentation to effectively manage patient care.
Outline:
- Instrument Review
- Evidence Based Decision Making and Treatment Planning
- Medical Emergency Management Review
- Introduction to Special Needs Patients
- Powered Instruments
- Ultrasonic
- Piezo
- Sonic
- Air Powder Polishing and Stain Removal
- Care of Dental Prosthesis
- Instrumentation and Alternate Fulcrums
- Tobacco Cessation
- Subgingival Irrigation
- Antimicrobials
- Dental Implant Instruments
- Case Studies
- Table Clinics
- Laboratory Procedures
- Air-jet polisher
- Assembly monitoring of sterilizers
- Biologic monitoring of sterilizers
- Desensitizing agents
- Cleaning of removal partials/dentures
- Implant instrument identification
- Intra-oral camera armentarium
- Subgingival irrigation techniques – antimicrobials
- Instrument sharpening
- Ultrasonics
- Ultrasonic and piezo powered instruments
- Diagnodent
- VELscope
- Midwest Caries ID pen
- Caries risk assessment
Effective Term: Fall 2016 |
|
-
DHE 150LB - Dental Hygiene II Lab 0.5 Credits, 1.5 Contact Hours 0 lecture periods 15 lab periods
This is the lab portion of DHE 150. Application of dental hygiene skills with a variety of clinical patients with simple dental hygiene care plans. Includes instrument review, evidence-based decision making and treatment planning, medical emergency management review, special needs patients, powered instruments, air powder polishing and stain removal, care of dental prostheses, advanced instrumentation and alternate fulcrums, tobacco cessation, subgingival irrigation, and antimicrobials. Also includes dental implant instruments, case studies, table clinics, and laboratory procedures.
Prerequisite(s): DHE101/101LC, 104/104LB, 107, 112 and 116/116LC. Corequisite(s): DHE 119, DHE 120, DHE 122, DHE 132, DHE 132LB, DHE 150, DHE 150LC Information: Students must be admitted to the PCC Dental Hygiene program and obtain consent of the Dental Hygiene department before enrolling in this course.
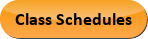
Course Learning Outcomes
- Demonstrate professional and ethical behavior in patient care.
- Apply asepsis protocol of recommended clinical guidelines for infection control and hazard management prior, during and after the provision of dental hygiene services.
- Demonstrate proper body mechanics techniques for optimal operator and patient comfort.
- Identify appropriate instruments for patients with varying dental needs.
- Assess patient’s needs for preventive, educational and therapeutic dental hygiene clinical services and interpret dental radiographs for dental disease.
- Describe evidence-based decision making principles and clinical reasoning to patient care plans.
- Integrate, synthesize, and evaluate oral health services of diverse patient population groups through community service learning activities.
- Apply knowledge of powered instruments, application of dental sealants, practice of medical emergency procedures, and computer applications and record documentation to effectively manage patient care.
Outline:
- Instrument Review
- Evidence Based Decision Making and Treatment Planning
- Medical Emergency Management Review
- Introduction to Special Needs Patients
- Powered Instruments
- Ultrasonic
- Piezo
- Sonic
- Air Powder Polishing and Stain Removal
- Care of Dental Prosthesis
- Instrumentation and Alternate Fulcrums
- Tobacco Cessation
- Subgingival Irrigation
- Antimicrobials
- Dental Implant Instruments
- Case Studies
- Table Clinics
- Laboratory Procedures
- Air-jet polisher
- Assembly monitoring of sterilizers
- Biologic monitoring of sterilizers
- Desensitizing agents
- Cleaning of removal partials/dentures
- Implant instrument identification
- Intra-oral camera armentarium
- Subgingival irrigation techniques – antimicrobials
- Instrument sharpening
- Ultrasonics
- Ultrasonic and piezo powered instruments
- Diagnodent
- VELscope
- Midwest Caries ID pen
- Caries risk assessment
Effective Term: Fall 2016 |
|
-
DHE 150LC - Dental Hygiene II Clinical 3 Credits, 12 Contact Hours 0 lecture periods 12 lab periods
This is the clinical lab portion of DHE 150 . Application of dental hygiene skills with a variety of clinical patients with simple dental hygiene care plans. Includes instrument review, evidence-based decision making and treatment planning, medical emergency management review, special needs patients, powered instruments, air powder polishing and stain removal, care of dental prostheses, advanced instrumentation and alternate fulcrums, tobacco cessation, subgingival irrigation, and antimicrobials. Also includes dental implant instruments, case studies, table clinics, and laboratory procedures.
Prerequisite(s): DHE 101 , DHE 101LC , DHE 104 , DHE 104LB , DHE 107 , DHE 112 , and DHE 116LC Corequisite(s): DHE 119 , DHE 120 , DHE 122 , DHE 132 , DHE 132LB , DHE 150 , DHE 150LB Information: Students must be admitted to the PCC Dental Hygiene program and obtain consent of the Dental Hygiene department before enrolling in this course.
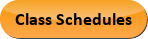
Course Learning Outcomes
- Demonstrate professional and ethical behavior in patient care.
- Apply asepsis protocol of recommended clinical guidelines for infection control and hazard management prior, during and after the provision of dental hygiene services.
- Demonstrate proper body mechanics techniques for optimal operator and patient comfort.
- Identify appropriate instruments for patients with varying dental needs.
- Assess patient’s needs for preventive, educational and therapeutic dental hygiene clinical services and interpret dental radiographs for dental disease.
- Describe evidence-based decision making principles and clinical reasoning to patient care plans.
- Integrate, synthesize, and evaluate oral health services of diverse patient population groups through community service learning activities.
- Apply knowledge of powered instruments, application of dental sealants, practice of medical emergency procedures, and computer applications and record documentation to effectively manage patient care.
Outline:
- Instrument Review
- Evidence Based Decision Making and Treatment Planning
- Medical Emergency Management Review
- Introduction to Special Needs Patients
- Powered Instruments
- Ultrasonic
- Piezo
- Sonic
- Air Powder Polishing and Stain Removal
- Care of Dental Prosthesis
- Instrumentation and Alternate Fulcrums
- Tobacco Cessation
- Subgingival Irrigation
- Antimicrobials
- Dental Implant Instruments
- Case Studies
- Table Clinics
- Laboratory Procedures
- Air-jet polisher
- Assembly monitoring of sterilizers
- Biologic monitoring of sterilizers
- Desensitizing agents
- Cleaning of removal partials/dentures
- Implant instrument identification
- Intra-oral camera armentarium
- Subgingival irrigation techniques – antimicrobials
- Instrument sharpening
- Ultrasonics
- Ultrasonic and piezo powered instruments
- Diagnodent
- VELscope
- Midwest Caries ID pen
- Caries risk assessment
Effective Term: Fall 2016 |
|
-
DHE 160LC - Clinical Skills Enhancement I .25-2 Credits, 1-8 Contact Hours 0 lecture periods 1-8 lab periods
A clinical remediation course designed to support identified first year dental hygiene students who are performing at or below clinic course expectations. Includes education plan, development of individualized clinical remediation plan, and assessment.
Information: Students must be admitted to the PCC Dental Hygiene program and obtain consent of the Dental Hygiene department before enrolling in this course. May be taken two times for a maximum of four credit hours. If this course is repeated, see a financial aid or Veteran’s Affairs advisor to determine funding eligibility as appropriate.
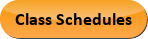
Course Learning Outcomes
- Utilize effective remediation clinical skills which may include but are not limited to:
- Speed of care
- Sequencing of care delivery
- Prioritization of care delivery/time management
- Skill refinement
- Documentation
- Data collection
- Patient safety
- Organization
- Communication
- Perform and participate in the assessment portion of the course with supervising faculty.
- Develop an educational plan with dental hygiene faculty based upon student’s identified clinical deficiencies.
- Develop an individualized clinical remediation plan.
- Measure his or her success in the areas of deficiencies
Outline:
- Introduction, Sources, and Regulatory Agencies
- Terminology
- Printed sources
- Computer sources
- Online sources
- Regulation and classification of drugs
- Labeling requirements
- Black box warning
- Drug Action and Handling and Adverse Reactions
- Routes of drug administration
- Pharmacodynamics
- Drug effects
- Drug interactions
- Bioequivalence and bioavailability
- Prescription Writing
- Goals of prescription writing
- Units of measurement
- Latin abbreviations
- Safety of prescription pads
- Patient adherence
- Reduction of medication errors
- Prescribing for children
- Safety in pregnancy
- Autonomic Drugs
- Nervous system
- Neurotransmitters
- Sympathomimetic drugs
- Adrenergic agonists
- Adrenergic receptor antagonists
- transmission
- Non-Opioid Analgesics
- Neurophysiology of pain
- Drug therapy for dental pain
- Non-narcotic analgesics
- Non-steroidal anti-inflammation drugs
- Opioid analgesics
- Substance abuse and dependency
- Anti-Infective Agents
- Antimicrobial agents
- Bactericidal antibiotics
- Bacteriostatic antibiotics
- Miscellaneous antibiotics
- Prevention of infective endocarditis
- Antibacterial agents: topical
- Controlled-release drug delivery
- Anti-Fungal and Anti-Viral Agents
- Herpes simplex
- Antiretroviral agents
- Antifungal agents
- Anti-Anxiety Agents
- Benzodiazepines
- Barbiturates
- Nonbarbiturates
- Nitrous oxide
- Narcotics
- General anesthesia
- Cardiovascular Agents
- Hypertension
- Angina pectoris
- Heart failure
- Arrythmias
- Anti-Convulsant Agents
- Psychotherapeutic Agents
- Antipsychotic drugs
- Mood disorders
- Anxiolytics
- Sedative/hypnotic drugs
- Antacids and Antihistamines
- Adreno Cortico Steroid Agents
- Other Hormones
- Anti-Neoplastic and Immunosuppressant Agents
- Actions
- Treatment
- Adverse side effects
- Limitations to dental treatment
- Chemotherapy
- Respiratory and Gastrointestinal Medications
- Lung anatomy
- Asthma
- Cold
- Cough
- Peptic ulcer
- Irritable bowel syndrome
- Nausea and vomiting
- Constipation
- Diarrhea
- Inflammatory bowel disease
- Emergency Medications
- Preparation of dental staff
- Basic life support
- Emergency medical kit and equipment
- Drug Interactions and Drug Abuse
Effective Term: Fall 2016 |
|
-
DHE 208 - Pain and Anxiety Control for Dental Hygiene 1 Credits, 1 Contact Hours 1 lecture period 0 lab periods
Delivery of local anesthetics. Includes introduction to pain and anxiety control; pharmacology, neurophysiology, and local anesthetic agents; nitrous oxide and oxygen analgesia. Also includes health history and complications, treatment, laboratory practices on student partners, emergency procedures, and head and neck anatomy.
Prerequisite(s): DHE 119 , DHE 122 , DHE 132 , DHE 132LB , DHE 150 , DHE 150LB , DHE 150LC Corequisite(s): DHE 208LC , DHE 209 , DHE 212 , DHE 250 , DHE 250LC Information: Students must be admitted to the PCC Dental Hygiene program and obtain consent of the Dental Hygiene department before enrolling in this course.
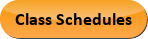
Course Learning Outcomes
- Describe and apply principles and techniques to manage pain control, pain/impulse conduction related to nerve anatomy and physiology along with possible interactions with other medications or health conditions.
- Describe the pharmacological properties, actions, considerations and contraindications to local anesthetic agents, vasoconstrictors, and nitrous oxide.
- Determine the appropriate pain control armamentarium, agents and techniques needed to ensure patient safety and comfort during the administration of local anesthesia and nitrous oxide – oxygen analgesia according to legal and ethical standards.
- Demonstrate competence in administering selected local anesthetic injections and nitrous oxide – oxygen analgesia.
Performance Objectives:
- Describe the need for pain control and explain pain/impulse conduction related to nerve anatomy and physiology.
- Describe the pharmacological properties, actions, considerations and contraindications to local anesthetic agents, vasoconstrictors, and nitrous oxide.
- Assess the client’s medico-dental history as it relates to choice of technique and agents used in the administration of local anesthetics and nitrous oxide – oxygen analgesia.
- Determine the appropriate pain control armamentarium, agents and techniques needed to ensure patient safety and comfort during the administration of local anesthesia and nitrous oxide – oxygen analgesia.
- Apply principles, techniques, and determine ways to prevent and manage potential emergency situations and any possible interactions with other medications or health conditions associated with dental anesthetics and nitrous oxide.
- Demonstrate competence in administering selected local anesthetic injections and nitrous oxide – oxygen analgesia.
- Practice pain control techniques according to legal and ethical standards.
- Apply highest standards of infection control and safety to protect the client and operator.
- Plan, present, and receive client consent for an individualized dental hygiene treatment plan, based upon the assessment data and medical history, for the anesthesia patient, including referral to appropriate health care professionals.
- Describe the limitations and indications for local inhalation anesthesia.
- Describe the related aspects of nitrous oxide oxygen to respiratory physiology.
- List the components, equipment and functions for a nitrous oxide delivery system and a scavenger system.
- Demonstrate the use and maintenance of nitrous oxide delivery system equipment.
- Explain the need for oxygen and describe how to administer it.
- Review clinic protocols for medical emergency procedures.
Outline:
- Introduction to Pain and Anxiety Control
- Pharmacology, Neurophysiology, and Local Anesthetic Agents
- Pharmacology of local anesthetics and vasoconstrictors
- Neurophysiology/how local anesthetics work
- Topical and local anesthetics used in dentistry
- Selecting a local anesthetic agent
- Calculating amounts of local anesthetics/vasoconstrictors
- Nitrous Oxide and Oxygen Analgesia
- National Institute for Occupational Safety and Health (NIOSH) update and steps for prevention
- Signs and symptoms of nitrous oxide and oxygen analgesia
- Management of nitrous oxide and oxygen analgesia complications
- Health History and Complications
- Health history evaluation
- Drug interactions
- Local and systemic complications
- Treatment
- Treatment planning for local anesthesia
- Ethical and legal considerations
- Laboratory Practice on Student Partners
- Posterior superior alveolar
- Middle superior alveolar
- Anterior superior alveolar
- Greater palatine
- Nasopalatine
- Inferior alveolar
- Lingual
- Mental
- Long buccal
- Infraorbital
- Gow-Gates
- Second division
- Akinosi
- Intramuscular
- Nitrous oxide and oxygen analgesia
- Emergency Procedures
- Head and Neck Anatomy
Effective Term: Fall 2016 |
|
-
DHE 208LC - Pain and Anxiety Control for Dental Hygiene Clinical 1 Credits, 4 Contact Hours 0 lecture periods 4 lab periods
This is the clinical lab portion of DHE 208 . Delivery of local anesthetics. Includes introduction to pain and anxiety control; pharmacology, neurophysiology, and local anesthetic agents; nitrous oxide and oxygen analgesia. Also includes health history and complications, treatment, laboratory practices on student partners, emergency procedures, and head and neck anatomy.
Prerequisite(s): , DHE 120 , DHE 122 , DHE 132 , DHE 132LB , DHE 150 , DHE 150LB , DHE 150LC Corequisite(s): DHE 208 , DHE 209 , DHE 212 , DHE 250 , DHE 250LC Information: Students must be admitted to the PCC Dental Hygiene program and obtain consent of the Dental Hygiene department before enrolling in this course.
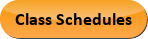
Course Learning Outcomes
- Describe and apply principles and techniques to manage pain control, pain/impulse conduction related to nerve anatomy and physiology along with possible interactions with other medications or health conditions.
- Describe the pharmacological properties, actions, considerations and contraindications to local anesthetic agents, vasoconstrictors, and nitrous oxide.
- Determine the appropriate pain control armamentarium, agents and techniques needed to ensure patient safety and comfort during the administration of local anesthesia and nitrous oxide – oxygen analgesia according to legal and ethical standards.
- Demonstrate competence in administering selected local anesthetic injections and nitrous oxide – oxygen analgesia.
Performance Objectives:
- Describe the need for pain control and explain pain/impulse conduction related to nerve anatomy and physiology.
- Describe the pharmacological properties, actions, considerations and contraindications to local anesthetic agents, vasoconstrictors, and nitrous oxide.
- Assess the client’s medico-dental history as it relates to choice of technique and agents used in the administration of local anesthetics and nitrous oxide – oxygen analgesia.
- Determine the appropriate pain control armamentarium, agents and techniques needed to ensure patient safety and comfort during the administration of local anesthesia and nitrous oxide – oxygen analgesia.
- Apply principles, techniques, and determine ways to prevent and manage potential emergency situations and any possible interactions with other medications or health conditions associated with dental anesthetics and nitrous oxide.
- Demonstrate competence in administering selected local anesthetic injections and nitrous oxide – oxygen analgesia.
- Practice pain control techniques according to legal and ethical standards.
- Apply highest standards of infection control and safety to protect the client and operator.
- Plan, present, and receive client consent for an individualized dental hygiene treatment plan, based upon the assessment data and medical history, for the anesthesia patient, including referral to appropriate health care professionals.
- Describe the limitations and indications for local inhalation anesthesia.
- Describe the related aspects of nitrous oxide oxygen to respiratory physiology.
- List the components, equipment and functions for a nitrous oxide delivery system and a scavenger system.
- Demonstrate the use and maintenance of nitrous oxide delivery system equipment.
- Explain the need for oxygen and describe how to administer it.
- Review clinic protocols for medical emergency procedures.
Outline:
- Introduction to Pain and Anxiety Control
- Pharmacology, Neurophysiology, and Local Anesthetic Agents
- Pharmacology of local anesthetics and vasoconstrictors
- Neurophysiology/how local anesthetics work
- Topical and local anesthetics used in dentistry
- Selecting a local anesthetic agent
- Calculating amounts of local anesthetics/vasoconstrictors
- Nitrous Oxide and Oxygen Analgesia
- National Institute for Occupational Safety and Health (NIOSH) update and steps for prevention
- Signs and symptoms of nitrous oxide and oxygen analgesia
- Management of nitrous oxide and oxygen analgesia complications
- Health History and Complications
- Health history evaluation
- Drug interactions
- Local and systemic complications
- Treatment
- Treatment planning for local anesthesia
- Ethical and legal considerations
- Laboratory Practice on Student Partners
- Posterior superior alveolar
- Middle superior alveolar
- Anterior superior alveolar
- Greater palatine
- Nasopalatine
- Inferior alveolar
- Lingual
- Mental
- Long buccal
- Infraorbital
- Gow-Gates
- Second division
- Akinosi
- Intramuscular
- Nitrous oxide and oxygen analgesia
- Emergency Procedures
- Head and Neck Anatomy
Effective Term: Fall 2016 |
|
-
DHE 209 - Ethics and Practice Management 1 Credits, 1 Contact Hours 1 lecture period 0 lab periods
Application of ethical theories and ethical principles in the practice of dental hygiene. Includes the business of dentistry, dental hygiene career opportunities, ethics, and jurisprudence.
Prerequisite(s): DHE 119 , DHE 120 , DHE 122 , DHE 132 , DHE 132LB , DHE 150 , DHE 150LB , and DHE 150LC Corequisite(s): DHE 208LC , DHE 208 , DHE 212 , DHE 250 , DHE 250LC Information: Students must be admitted to the PCC Dental Hygiene program and obtain consent of the Dental Hygiene department before enrolling in this course.
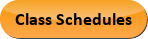
Course Learning Outcomes
- Describe the core values found in the Code of Ethics of the American Dental Hygienists’ Association and explain the terms used in ethical principles.
- Identify and discuss ethical principles and ethical theories and their application to the practice of dental hygiene and within the workplace.
- Discuss and apply basic concepts of business management, social issues identification that can affect the dental hygienist in an employment environment.
Performance Objectives:
- Identify and discuss ethical theories and principles and their application to dental hygiene practice.
- Differentiate between social philosophy theories and their role in dental hygiene patient care.
- Describe principle components of criminal, civil and tort law.
- Discuss social issues within workplace legislation and how they can affect your employment environment.
- Apply basic concepts of business management to the dental or dental hygiene component of a practice.
- Demonstrate ability to effectively schedule dental hygiene procedures in a dental office.
- Critique current time management and periodontal maintenance systems that may be used in dentistry.
- Identify criteria for informed consent.
- Identify different employment opportunities for dental hygienists.
- Prepare a resume and cover letter for future employment.
- Discuss economic considerations for a profitable practice, including production, collection, and office overhead.
- Explain the contributions of the dental hygienist to the dental office team.
- Develop a mission statement and goals for the dental hygiene component of a dental practice.
- Determine the productivity of the dental hygiene component of a dental practice.
- List and define the elements necessary for a complete case presentation.
- Define the term “marketing” as it relates to the dental practice.
- Discuss marketing strategies for practice promotion and elements of a practice that enhance client satisfaction.
- Express the importance of a periodontal maintenance system.
- Evaluate the different types of periodontal maintenance systems.
- Design an inventory control system for dental hygiene supplies.
- Differentiate insurance codes and nomenclature used for dental hygiene services.
- Design an employment contract, including elements of setting job descriptions, compensation, terms of employment evaluation, and termination procedures.
- Evaluate job performance, including expectations and techniques necessary for changing performance.
- Write an employment resume and cover letter.
- Access the Internet via the World Wide Web for researching dental and dental hygiene related topics.
Outline:
- The Business of Dentistry
- Patient and time management – appointment scheduling
- Dental insurance and bookkeeping systems
- Marketing plan
- Patient recall systems
- Employment contract
- Ethics and jurisprudence
- Dental Hygiene Career Opportunities
- Searching for employment
- Marketing your skills
- Resume/cover letter
- Job interviews
- Ethics
- Ethical principles and core values
- Ethical theories
- Social philosophy
- Ethical decision making in dental hygiene practice
- Jurisprudence
- Criminal, civil, and tort law
- Contract law
- Licensure
- State Dental Practice Act
Effective Term: Fall 2016 |
|
-
DHE 212 - Nutrition for Oral Health 1 Credits, 1 Contact Hours 1 lecture period 0 lab periods
Introduction of the principles of nutrition including food sources, digestion, absorption, and metabolism of nutrients essential to the oral health of individuals. Includes nutrition as the foundation for general and oral health, nutritional and oral implications of common chronic health conditions, carbohydrates, proteins, lipids, fats, minerals and mineralization, medications and oral health, and nutritional concerns for the dentally compromised patient.
Prerequisite(s): DHE 119 , DHE 120 , DHE 122 , DHE 132 , DHE 132LB , DHE 150 , DHE 150LB , and DHE 150LC Corequisite(s): DHE 208LC , DHE 208 , DHE 209 , DHE 250 , DHE 250LC Information: Students must be admitted to the PCC Dental Hygiene program and obtain consent of the Dental Hygiene department before enrolling in this course.
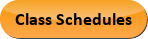
Course Learning Outcomes
- Identify the components of the oral cavity, essential nutrients, and nutrition classes that support optimal oral health and the function of each part of the human digestive tract.
- Describe the oral implications of chronic health conditions which include, but are not limited to hypertension, osteoporosis and immune deficiency disorders and dental caries.
- Describe the nutritional concerns associated with the dentally compromised patient as they relate to oral surgery, orthodontics, prosthetics (dentures), dysphagia, temporomandibular disorders, and cognitive and physical impairments.
Performance Objectives:
- Explain the oral and general physical conditions associated with malnutrition.
- Describe the parts and function of each part of the human digestive tract.
- Summarize how diet can affect the health of the human dentition.
- Explain the relationship between diet and dental caries.
- Describe the oral implications of chronic health conditions which include, but are not limited to hypertension, osteoporosis and immune deficiency disorders.
- Identify the function, chemistry, classification, sources, digestion, absorption, transport, and storage of carbohydrates.
- Identify the chemistry, synthesis, digestion, absorption, metabolism, sources, deficiency, digestion, absorption, transport, and storage of proteins.
- Identify the function, types, compound lipids, derived lipids, digestion, absorption, transport, and storage of fats.
- Summarize the mineralization of bones and teeth and the role of electrolytes and trace elements in this process.
- Outline the impact medications can have on nutrition and oral health with focus upon drug-nutrient interactions, nutritional implications of common medications, and the oral effects of medications.
- Describe the nutritional concerns associated with the dentally compromised patient as they relate to oral surgery, orthodontics, prosthetics (dentures), dysphagia, temporomandibular disorders, and cognitive and physical impairments.
Outline:
- Nutrition as the Foundation for General and Oral Health
- Oral cavity
- Essential nutrients
- Nutrient classes
- Malnutrition
- Digestive tract
- Diet, Nutrition, and Teeth
- Local effects of diet on teeth
- Diet and dental caries
- Nutritional and Oral Implications of Common Chronic Health Conditions
- Hypertension
- Osteoporosis
- Immune system disorders
- Carbohydrates, Diabetes, and Associated Health Conditions
- Carbohydrates
- Function
- Chemistry
- Classification
- Sources
- Digestion, absorption, transport, and storage
- Carbohydrate-related issues and concerns
- Proteins for System and Oral Health
- Chemistry
- Synthesis
- Digestion and absorption
- Metabolism
- Sources
- Deficiency
- Digestion, absorption, transport, and storage
- Lipids and Fats in Health and Disease
- Function
- Types
- Compound lipids
- Derived lipids
- Digestion, absorption, transport, and storage
- Minerals and Mineralization
- Mineralization of bones and teeth
- Mineralizing minerals
- Electrolytes
- Trace elements (microminerals)
- How Medications Can Affect Nutrition and Oral Health
- Drug-nutrient interactions
- Nutritional implications of common medications
- Oral effects and medications
- Nutritional Concerns for the Dentally Compromised Patient
- Oral surgery
- Orthodontics
- Dentures
- Dysphagia
- Temporomandibular disorders
- Cognitive and physical impairments
Effective Term: Fall 2016 |
|
-
DHE 213 - Advanced Periodontal Services 2 Credits, 2 Contact Hours 2 lecture periods 0 lab periods
Application of Dental Hygiene skills on advanced periodontal patients. Includes periodontal exam and initial phase, treatment plan, periodontal classifications, plaque control, scaling and root planning indications and limitations, sonic and ultrasonic therapy in periodontal services, hand and powered instrumentation, and implant maintenance. Also includes occlusal evaluation and adjustment, assessment, reevaluation of treatment and maintenance, periodontal healing, antimicrobials and antibiotics, surgical procedures, and nonsurgical periodontal therapy.
Prerequisite(s): DHE 208LC , DHE 208 , DHE 212 , DHE 250 , DHE 250LC Corequisite(s): DHE 213CA , DHE 213CB , DHE 216 , DHE 255 , DHE 255LC Information: Students must be admitted to the PCC Dental Hygiene program and obtain consent of the Dental Hygiene department before enrolling in this course.
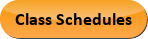
Course Learning Outcomes
- Describe the use of lasers in dentistry, safety precautions, steps in applying lasers for bacterial reduction.
- Demonstrate the use of emerging dental technology at the level of competency.
- Evaluate the components of the periodontal examination and explain how they serve as diagnostic aids for determination of the initial phase of therapy.
- Integrate, synthesize, and evaluate an individualized dental hygiene treatment plan for the periodontal patient.
- Summarize the American Academy of Periodontology (AAP) periodontal classifications.
- Explain oral conditions indicative of the need for scaling and root planning and outline the purpose of the initial phase of therapy.
- Demonstrate appropriate instrumentation techniques on patients with periodontal disease.
- Create an individualized dental hygiene care plan and maintenance program for the periodontal patient
- Evaluate possible outcomes after periodontal treatment.
- Describe the physiological process of the reattachment of periodontal tissues as well as the formation of new fiber attachment.
- Explain the purpose and oral conditions associated with gingival curettage, flap surgeries, gingivectomies, mucogingival surgeries, regenerative procedures and implant surgeries.
Outline:
- Periodontal Exam and Initial Phase
- Treatment Plan
- Periodontal Classifications
- Plaque Control for the Periodontal Patient
- Scaling and Root Planning Indications and Limitations
- Sonic and Ultrasonic Therapy in Periodontal Services
- Hand and Powered Instrumentation
- Implant Maintenance
- Occlusal Evaluation and Adjustment
- Reevaluation of Treatment and Maintenance
- Periodontal Healing – Reattachment/New Attachment
- Antimicrobials, Antibiotics, and Soft Tissue Dental Lasers in Periodontal Therapy
- Use of lasers
- Safety procedures
- Steps for bacterial reduction
- Surgical Procedures
- Gingival curettage
- Gingivectomies
- Flap surgeries
- Mucogingival surgeries
- Regenative surgical procedures
- Implant surgery
- Non-Surgical Periodontal Therapy
Effective Term: Full Academic Year 2018/19 |
|
-
DHE 213CA - Advanced Periodontal Services Clinical - A 1 Credits, 3 Contact Hours 0 lecture periods 3 lab periods
This is the clinical lab part A portion of DHE 213 . Application of Dental Hygiene skills on advanced periodontal patients. Includes periodontal exam and initial phase, treatment plan, periodontal classifications, plaque control, scaling and root planning indications and limitations, sonic and ultrasonic therapy in periodontal services, hand and powered instrumentation, and implant maintenance. Also includes occlusal evaluation and adjustment, assessment, reevaluation of treatment and maintenance, periodontal healing, antimicrobials and antibiotics, surgical procedures, and nonsurgical periodontal therapy.
Prerequisite(s): DHE 208 , DHE 208LC , DHE 209 , DHE 212 , DHE 250 ,and DHE 250LC . Corequisite(s): DHE 213 , DHE 213CB , DHE 216 , DHE 255 , DHE 255LC Information: Students must be admitted to the PCC Dental Hygiene program and obtain consent of the Dental Hygiene department before enrolling in this course.
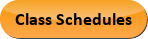
Course Learning Outcomes
- Describe the use of lasers in dentistry, safety precautions, steps in applying lasers for bacterial reduction.
- Demonstrate the use of emerging dental technology at the level of competency.
- Evaluate the components of the periodontal examination and explain how they serve as diagnostic aids for determination of the initial phase of therapy.
- Integrate, synthesize, and evaluate an individualized dental hygiene treatment plan for the periodontal patient.
- Summarize the American Academy of Periodontology (AAP) periodontal classifications.
- Explain oral conditions indicative of the need for scaling and root planning and outline the purpose of the initial phase of therapy.
- Demonstrate appropriate instrumentation techniques on patients with periodontal disease.
- Create an individualized dental hygiene care plan and maintenance program for the periodontal patient
- Evaluate possible outcomes after periodontal treatment.
- Describe the physiological process of the reattachment of periodontal tissues as well as the formation of new fiber attachment.
- Explain the purpose and oral conditions associated with gingival curettage, flap surgeries, gingivectomies, mucogingival surgeries, regenerative procedures and implant surgeries.
Outline:
- Periodontal Exam and Initial Phase
- Treatment Plan
- Periodontal Classifications
- Plaque Control for the Periodontal Patient
- Scaling and Root Planning Indications and Limitations
- Sonic and Ultrasonic Therapy in Periodontal Services
- Hand and Powered Instrumentation
- Implant Maintenance
- Occlusal Evaluation and Adjustment
- Reevaluation of Treatment and Maintenance
- Periodontal Healing – Reattachment/New Attachment
- Antimicrobials, Antibiotics, and Soft Tissue Dental Lasers in Periodontal Therapy
- Use of lasers
- Safety procedures
- Steps for bacterial reduction
- Surgical Procedures
- Gingival curettage
- Gingivectomies
- Flap surgeries
- Mucogingival surgeries
- Regenative surgical procedures
- Implant surgery
- Non-Surgical Periodontal Therapy
Effective Term: Full Academic Year 2018/19 |
|
-
DHE 213CB - Advanced Periodontal Services Clinical - B 1 Credits, 4 Contact Hours 0 lecture periods 4 lab periods
This is the clinical lab portion part B of DHE 213 . Application of Dental Hygiene skills on advanced periodontal patients. Includes periodontal exam and initial phase, treatment plan, periodontal classifications, plaque control, scaling and root planning indications and limitations, sonic and ultrasonic therapy in periodontal services, hand and powered instrumentation, and implant maintenance. Also includes occlusal evaluation and adjustment, assessment, reevaluation of treatment and maintenance, periodontal healing, antimicrobials and antibiotics, surgical procedures, and nonsurgical periodontal therapy.
Prerequisite(s): DHE 208 , DHE 208LC , DHE 209 , DHE 212 , DHE 250LC ,and DHE 250 Corequisite(s): DHE 213 , DHE 213CA , DHE 216 , DHE 255 , DHE 255LC Information: Students must be admitted to the PCC Dental Hygiene program and obtain consent of the Dental Hygiene department before enrolling in this course.
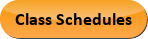
Course Learning Outcomes
- Describe the use of lasers in dentistry, safety precautions, steps in applying lasers for bacterial reduction.
- Demonstrate the use of emerging dental technology at the level of competency.
- Evaluate the components of the periodontal examination and explain how they serve as diagnostic aids for determination of the initial phase of therapy.
- Integrate, synthesize, and evaluate an individualized dental hygiene treatment plan for the periodontal patient.
- Summarize the American Academy of Periodontology (AAP) periodontal classifications.
- Explain oral conditions indicative of the need for scaling and root planning and outline the purpose of the initial phase of therapy.
- Demonstrate appropriate instrumentation techniques on patients with periodontal disease.
- Create an individualized dental hygiene care plan and maintenance program for the periodontal patient
- Evaluate possible outcomes after periodontal treatment.
- Describe the physiological process of the reattachment of periodontal tissues as well as the formation of new fiber attachment.
- Explain the purpose and oral conditions associated with gingival curettage, flap surgeries, gingivectomies, mucogingival surgeries, regenerative procedures and implant surgeries.
Outline:
- Periodontal Exam and Initial Phase
- Treatment Plan
- Periodontal Classifications
- Plaque Control for the Periodontal Patient
- Scaling and Root Planning Indications and Limitations
- Sonic and Ultrasonic Therapy in Periodontal Services
- Hand and Powered Instrumentation
- Implant Maintenance
- Occlusal Evaluation and Adjustment
- Reevaluation of Treatment and Maintenance
- Periodontal Healing – Reattachment/New Attachment
- Antimicrobials, Antibiotics, and Soft Tissue Dental Lasers in Periodontal Therapy
- Use of lasers
- Safety procedures
- Steps for bacterial reduction
- Surgical Procedures
- Gingival curettage
- Gingivectomies
- Flap surgeries
- Mucogingival surgeries
- Regenative surgical procedures
- Implant surgery
- Non-Surgical Periodontal Therapy
Effective Term: Full Academic Year 2018/19 |
|
-
DHE 216 - Community and Dental Health Education 3 Credits, 3 Contact Hours 3 lecture periods 0 lab periods
Overview of public dental health education. Includes critiquing dental literature, community dental health planning, basic biostatistics, and epidemiology and research in the dental community. Also includes dental needs and demands, dental care delivery and prevention in the United States.
Prerequisite(s): DHE 208 , DHE 208LC , DHE 209 , DHE 212 , DHE 250 , and DHE 250LC . Corequisite(s): DHE 213 , DHE 213CA , DHE 213CB , DHE 255 , DHE 255LC Information: Students must be admitted to the PCC Dental Hygiene program and obtain consent of the Dental Hygiene department before enrolling in this course.
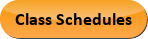
Course Learning Outcomes
- Develop a dental health presentation based on the needs of a population.
- Integrate, synthesize, and evaluate a dental health program in a variety of settings.
- Demonstrate educational principles of learning during the oral health presentation.
- Perform, analyze, and interpret data for: central tendency, reliability, validity, and probability.
- Critique a professional journal article for content and research.
- Explain the dental health care system in the United States.
- Discuss the dental needs, demand, and utilization in the United States.
- Discuss the history, benefits, and issues of water fluoridation in the United States.
Outline:
- Critiquing Dental Literature
- Criteria for review
- The scientific method
- Steps in Community Dental Health Program Planning
- Assessing the population
- Planning the program
- Implementing the program
- Education strategies
- Principles and methods
- Learning and teaching
- Evaluating the program
- Basic Biostatistics
- Sampling
- Data statistics
- Statistical decision making
- Epidemiology
- Caries indices
- Gingival and periodontal indices
- Other indices
- Research in the Dental Community
- Types of research
- Ethical and legal considerations in research
- Dental Needs and Demand in the United States
- Dental Care Delivery in the United States
- Prevention of Oral Disease in Public Health
Effective Term: Fall 2016 |
|
-
DHE 250 - Dental Hygiene III 3 Credits, 3 Contact Hours 3 lecture periods 0 lab periods
Continuation of DHE 150 , DHE 150LB , and DHE 150LC . Application of dental hygiene skills with a variety of clinical patients with dental hygiene care plans at the intermediate level. Includes dental hygiene theory and care, instrumentation, and care of patients with various physical disabilities.
Prerequisite(s): DHE 119 , DHE 120 , DHE 122 , DHE 132 , DHE 132LB , DHE 150 , DHE 150LB , and DHE 150LC Corequisite(s): DHE 208 , DHE 208LC , DHE 209 , DHE 212 , DHE 250LC Information: Students must be admitted to the PCC Dental Hygiene program and obtain consent of the Dental Hygiene department before enrolling in this course.
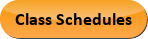
Course Learning Outcomes
- Develop a dental hygiene care plan; formulate a dental hygiene diagnosis, using the dental hygiene human needs concept.
- Discuss modifications to dental hygiene care and oral health education for the mentally challenged and for the patients with physical disabilities.
- Define and collected available treatment alternatives that relate to periodontal disease and the specific treatment that satisfies the needs for patient oral health.
- Demonstrate intermediate to advanced instrumentation skills with a vast variety of dental instruments.
Performance Objectives:
- Construct a dental hygiene care plan using the dental hygiene human needs concept.
- Formulate a dental hygiene diagnosis using the patient’s assessment finds.
- Organize and apply the patient’s dental hygiene diagnosis into a comprehensive treatment plan.
- Construct an appointment plan using the comprehensive treatment plan.
- Describe the importance of the re-evaluation appointment.
- Identify characteristic for patients with physical and sensory disabilities.
- Describe the differences between universal and area-specific curets.
- Compare and contrast between power-driven and hand scaling instruments.
- List concerns in a dental office for treating a patient with physical and sensory disabilities.
- List concerns in a dental office for treating a patient with a cleft lip or palate.
- Decide when to refer for additional supportive care for patients with physical and sensory disabilities.
- List the possible oral changes for the patients with physical disabilities.
- Modify the dental hygiene treatment to the needs of patients.
- Discuss modifications to dental hygiene care and oral health education for individuals with intellectual disorders.
- Explain how to escort a patient with a visual impairment to the dental chair and give patient education.
- Design strategies to communicate with a patient with a sensory impairment.
- List special considerations in dental treatment for the patient with arthritis.
- Develop a dental hygiene care plan for an individual undergoing chemotherapy or radiation therapy.
- Advise patients with oral cancer about how their chemotherapy or radiation therapy will affect their oral structures.
- Discuss the dental needs of HIV positive patients in the different stages and how to treat those patients.
- Identify a patient history that might be positive for hepatitis and list a dental hygiene care plan for the patient.
- Define infective endocarditis and identify potential risks for subacute bacterial endocarditis.
- Develop a dental hygiene management plan for patient with a history of congestive heart failure, heart attack, intrinsic heart disease, and cardiac arrhythmias.
- Describe the disease process of diabetes and discuss the dental hygiene treatment for managing the patient with diabetes.
- List the procedures for the mock board exam and participates in the exam.
- List causes of chronic obstructive pulmonary disease (COPD) and determine the treatment modification for patients with this disease.
- Discuss the oral sign associated with bleeding disorders.
- List the precautions and dental treatment modifications for patients with bleeding disorders.
- Identify oral manifestations of patients on dialysis.
- Relate the pharmacological precautions taken with a patient in renal failure.
Outline:
- Dental Hygiene Theory and Care
- Human needs theory and dental hygiene care
- Dental hygiene diagnosis
- Cross-cultural practice
- Dental hygiene care plan and evaluation
- Instrumentation
- Use of curet and sickle scalers and files
- Power-driven and hand scaling instruments
- Wheel Chair Transfer
- Care of Patients With Physical Disabilities
- Physical disability or impairment
- Sensory disability
- Cleft lip and/or palate
- Care of Patients With Mental Disabilities
- Intellectual Disorders
- Mental Disorder
- Alcohol-Related Disorder
- Eating Disorders
- Care of Patients with Health Conditions
- Seizure Disabilities
- Cardiovascular Disease
- Blood Disease
- Diabetes
- Oral Cancer
- Transplant Organs
- HIV/AIDS
- Hepatitis or Liver Disease
- Renal Failure and Dialysis
- Pulmonary Disease
Effective Term: Full Academic Year 2021/2022 |
|
-
DHE 250LC - Dental HygieneIII Clinical 4 Credits, 16 Contact Hours 0 lecture periods 16 lab periods
Continuation of DHE 150 , DHE 150LB , and DHE 150LC . This is the clinical lab portion of DHE 250. Application of dental hygiene skills with a variety of clinical patients with dental hygiene care plans at the intermediate level. Includes dental hygiene theory and care, instrumentation, and care of patients with various physical disabilities.
Prerequisite(s): DHE 119 , DHE 120 , DHE 122 , DHE 132 , DHE 132LB , DHE 150 , DHE 150LB , and DHE 150LC Corequisite(s): DHE 208 , DHE 208LC , DHE 209 , DHE 212 , DHE 250 Information: Students must be admitted to the PCC Dental Hygiene program and obtain consent of the Dental Hygiene department before enrolling in this course.
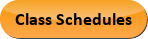
Course Learning Outcomes
- Develop a dental hygiene care plan; formulate a dental hygiene diagnosis, using the dental hygiene human needs concept.
- Discuss modifications to dental hygiene care and oral health education for the mentally challenged and for the patients with physical disabilities.
- Define and collected available treatment alternatives that relate to periodontal disease and the specific treatment that satisfies the needs for patient oral health.
- Demonstrate intermediate to advanced instrumentation skills with a vast variety of dental instruments.
Performance Objectives:
- Provide home care instructions based upon patient needs an interest level.
- Provide nutritional counseling service to clinical patients as treatment planned.
- Write up a dental hygiene care plan for patient services.
- Formulate a dental hygiene diagnosis.
- Respond to any medical emergencies.
- Explain etiology and prognosis of dental disease so that the patient understands his role in home care.
- Use radiographic surveys to detect abnormal tissues, detect calculus, critique technique, and identify normal landmarks.
- Expose radiographic surveys after determining the patient’s needs and diagnostic yield.
- Record findings from initial extra-oral and extra-oral exams.
- Demonstrate treatment procedures for medically compromised patients with the implementation of appropriate precautionary measures.
- Consult with the patient’s physicians in determining the appropriate modification in dental hygiene service for each patient recognized as a medically compromised.
- Complete a periodontal record for baseline information and provide updates for a clinical patient under treatment.
- Prepare dental hygiene treatment plans for patients who exhibit intermediate levels of periodontal disease.
- Integrate preventative measures, along with periodontal disease control services, for each patient indicated for these services.
- Explain and establish referrals to the dental specialties for patients who require additional dental treatment.
- Integrate dental care with a total approach to provide optimal health care.
- Practice consistent universal precautions, asepsis and safety standards in the dental environment.
- Evaluate the success of recommendations for the use of home care instructions and the patient’s plaque control techniques, based upon the tissue responses.
- Apply root-planning techniques when indicted for smoothing the cementum.
- Evaluate an individual patient success with prescribe plaque control techniques.
- Incorporate the use of diagnostic radiographs for the detection and treatment of periodontal disease.
- Successful placement of composite and amalgam restorations on a typodont.
- Introduction of the use of lasers.
Outline:
- Dental Hygiene Theory and Care
- Human needs theory and dental hygiene care
- Dental hygiene diagnosis
- Cross-cultural practice
- Dental hygiene care plan and evaluation
- Instrumentation
- Use of curet and sickle scalers and files
- Power-driven and hand scaling instruments
- Composite and amalgam restorations
- Introduction to lasers
- Wheel Chair Transfer
- Care of Patients With Physical Disabilities
- Physical disability or impairment
- Sensory disability
- Cleft lip and/or palate
- Care of Patients With Mental Disabilities
- Mental Retardation
- Mental Disorder
- Alcohol-Related Disorder
- Care of Patients with Health Conditions
- Seizure Disabilities
- Cardiovascular Disease
- Blood Disease
- Diabetes
- Oral Cancer
- Transplant Organs
- HIV/AIDS
- Hepatitis or Liver Disease
- Renal Failure and Dialysis
- Pulmonary Disease
Effective Term: Full Academic Year 2018/19 |
|
-
DHE 255 - Dental Hygiene IV 1 Credits, 1 Contact Hours 1 lecture period 0 lab periods
Continuation of DHE 250 /DHE 250LC . Includes application of dental hygiene skills with a variety of clinical patients with dental hygiene care plans at the advanced level. Also includes national, regional, and state exam preparation, advanced instrumentation, advanced ultrasonic inserts and techniques, and preparing for entry level employment.
Prerequisite(s): DHE 208 , DHE 208LC , DHE 209 , DHE 212 , DHE 250 , and DHE 250LC . Corequisite(s): DHE 213 , DHE 213CA , DHE 213CB , DHE 216 , DHE 250LC Information: Students must be admitted to the PCC Dental Hygiene program and obtain consent of the Dental Hygiene department before enrolling in this course.
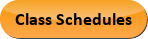
Course Learning Outcomes
- Outline patient and candidate requirements and procedures for the WREB clinical examination.
- Demonstrate advanced fulcrum techniques, advanced instrumentation, techniques for heavy calculus removal, and advanced techniques for root surface debridement at the level of competency.
- Demonstrate power instrumentation for proper angulation and adaptation.
- Apply the ADHA Code of Ethics as a guide for ethical consciousness, decision making, and practice.
- Document accurate information in the assessment, identification, planning, implementation, and evaluation phases of dental hygiene services.
- Demonstrate effective communicate skills verbally, non-verbally, both written and electronically.
- Apply knowledge of course materials to successfully pass National and Regional Board Exams.
Outline:
- American Dental Association (ADA) National Board Review and Preparation
- Western Regional Examining Board (WREB) Dental Hygiene Review and Preparation
- Identifying individual needs
- Mock exam
- Arizona State Board of Dental Examiners (AZSBDE) Jurisprudence Review and Preparation
- Ethics in dental hygiene
- Law and malpractice
- Mock exam
- Advanced Instrumentation
- Reinforcement scaling techniques
- Furcation instruments
- Mini-bladed gracey curets
- Advanced Ultrasonic Inserts and Techniques
- Insert selection
- Instrumentation technique
- Preparing for Entry Level Employment
Effective Term: Full Academic Year 2017/18 |
|
-
DHE 255LC - Dental Hygiene IV Clinical 3 Credits, 12 Contact Hours 0 lecture periods 12 lab periods
This is the clinical lab portion of DHE 255. Includes application of dental hygiene skills with a variety of clinical patients with dental hygiene care plans at the advanced level. Also includes national, regional, and state exam preparation, advanced instrumentation, advanced ultrasonic inserts and techniques, and preparing for entry level employment.
Prerequisite(s): DHE 208, 208LC, 209, 212, 250, and 250LC. Corequisite(s): DHE 213, DHE 213CA, DHE 213CB, DHE 216, DHE 255 Information: Students must be admitted to the PCC Dental Hygiene program and obtain consent of the Dental Hygiene department before enrolling in this course.
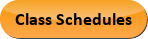
Course Learning Outcomes
- Outline patient and candidate requirements and procedures for the WREB clinical examination.
- Demonstrate advanced fulcrum techniques, advanced instrumentation, techniques for heavy calculus removal, and advanced techniques for root surface debridement at the level of competency.
- Demonstrate power instrumentation for proper angulation and adaptation, and proper use of lasers.
- Apply the ADHA Code of Ethics as a guide for ethical consciousness, decision making, and practice.
- Document accurate information in the assessment, identification, planning, implementation, and evaluation phases of dental hygiene services.
- Demonstrate effective communicate skills verbally, non-verbally, both written and electronically.
Outline:
- American Dental Association (ADA) National Board Review and Preparation
- Western Regional Examining Board (WREB) Dental Hygiene Review and Preparation
- Identifying individual needs
- Mock exam
- Advanced Instrumentation
- Reinforcement scaling techniques
- Furcation instruments
- Mini-bladed gracey curets
- Advanced Ultrasonic Inserts and Techniques
- Insert selection
- Instrumentation technique
- Preparing for Entry Level Employment
- Successful placement of composite and amalgam restorations into Kilgore tooth
- Use of laser therapy
Effective Term: Full Academic Year 2018/19 |
|
-
DHE 260LC - Clinical Skills Enhancement II .25-2 Credits, 1-4 Contact Hours 0 lecture periods 1-4 lab periods
A clinical remediation course designed to support identified second year dental hygiene students who are performing at or below clinic course expectations. Includes identification of need through clinical performance scores, development of individualized clinical remediation plan, and assessment.
Information: Students must be admitted to the PCC Dental Hygiene program and obtain consent of the Dental Hygiene department before enrolling in this course. May be taken two times for a maximum of four credit hours. If this course is repeated, see a financial aid or Veteran’s Affairs advisor to determine funding eligibility as appropriate.
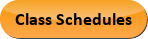
Course Learning Outcomes
- Develop an education plan with dental hygiene faculty based upon student’s identified clinical deficiencies.
- Development of an individualized clinical remediation plan.
- Measure his/her success in the areas of deficiencies.
Outline:
- Identification of Need for Remediation Through Clinical Performance Scores
- Faculty observation of student’s development
- Review clinic performance with student
- Confirmation of student interest in additional clinical instruction
- Development of Individualized Clinical Remediation Plan
- Skill remediation
- One-on-one clinical instruction
- Assessment
- Daily feedback for student
- Overall skill development progress
Effective Term: Fall 2016 |
Digital Arts |
|
-
DAR 101 - Color Rendering and Theory 4 Credits, 5 Contact Hours 3 lecture periods 2 lab periods
Basic color theory and rendering principles as applied to digital and graphic design. Includes color types, definition and use of color schemes, rendering concepts and techniques, media, technique, composition, designing characters for animation, three-dimensional techniques and construction, and professional environment.
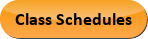
Course Learning Outcomes
- List color types and schemes.
- Describe color and it’s meaning in rendering and design.
- Create and render objects using basic concepts and techniques.
- Work within a professional environment meeting specifications and deadlines.
Outline:
- Color Types
- Primary
- Secondary
- Tertiary
- Definition and Use of Color Schemes
- Complementary
- Analogous
- Triadic
- Monochromatic
- Neutral
- Achromatic
- Rendering Concepts and Techniques
- Perspective
- Vanishing points
- Eye level line
- Proportion
- Light Sources
- Top lit
- Side lit
- Front lit
- Form
- Rectangular
- Spherical
- Cylindrical
- Media
- Ink
- Colored pencil
- Collage/paper construction
- Paint
- Three-dimensional modeling
- Technique
- Traditional
- Digital
- Combination
- Composition
- Dynamic
- Static
- Designing Characters for Animation
- Use various media to create an illustration
- Apply various techniques to an illustration
- Compose the elements in an illustration
- Three-Dimensional Techniques and Construction
- Armature
- Modeling applications
- Exterior color and texture
- Professional Environment
- Deadlines
- Critiques
Effective Term: Full Academic Year 2019/2020 |
|
-
DAR 102 - Fundamentals of Digital Design 4 Credits, 5 Contact Hours 3 lecture periods 2 lab periods
Overview of the fundamentals, theory, survey, and practice of digital arts design. Includes survey of industry careers, skills and processes necessary in digital design careers, digital arts software, and portfolio requirements in digital arts.
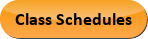
Course Learning Outcomes
- Identify the various commercial digital design careers, their inter-relationships, and the skills necessary to work in those careers.
- Discuss target audience and marketing strategies.
- Define portfolio standards for printed and digital portfolios locally, nationally, and globally.
- Work within a professional environment meeting specifications and deadlines.
Outline:
- Survey of Industry Careers
- Identify the various commercial digital design careers
- Inter-relationship of the careers
- Skills and Processes Necessary in Digital Design Careers
- Fundamentals of digital art design
- Principles of digital art design
- Defining target audience
- Marketing strategies using design techniques
- Digital Arts Software
- Adobe Illustrator
- Adobe Photoshop
- Adobe InDesign
- Portfolio Requirements in Digital Arts
- Printed books
- Digital portfolios
- Standards
Effective Term: Full Academic Year 2019/2020 |
|
-
DAR 103 - Introduction to Digital Video and Film Arts 3 Credits, 3 Contact Hours 3 lecture periods 0 lab periods
Overview of the theory and practice of the digital video and film arts industry. Includes various electronic media delivery systems, digital image, and target market and advertising.
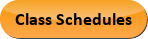
Course Learning Outcomes
- Describe the various electronic media delivery systems.
- Describe the use of the digital image for artistic, social, and professional purposes.
- Discuss the role of market and advertising in creating and providing content.
Outline:
- Various Electronic Media Delivery Systems
- Radio
- Television
- Cable
- Motion pictures
- Internet
- Emerging systems
- Game design
- Digital Image
- Artistic considerations
- Social trends and impact
- Professional purposes
- Intellectual and artistic property rights
- Target Market and Advertising
- Content as advertising
- Content as entertainment
- Analyzing the audience
Effective Term: Full Academic Year 2017/18 |
|
-
DAR 111 - Typography 4 Credits, 5 Contact Hours 3 lecture periods 2 lab periods
Letter forms and use in visual communications. Includes type rendering, letter spacing, type and headline groupings, type relationships, type images, and type applications.
Prerequisite(s): DAR 102 or DAR 103 .
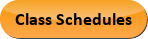
Course Learning Outcomes
- Design logo type and headlines into eye-catching groupings utilizing methods of alignment, word spacing, and line spacing.
- Create effective typographical headline, subheadline, and body text design relationships to ads, brochures, posters, logos, and other media.
- Produce typographical designs that project specific images appealing to formal and informal target audiences.
- Work within a professional environment meeting specifications and deadlines.
Outline:
- Type Rendering
- Proportions
- Griding methods
- Marker techniques
- Letter Spacing
- Kerning
- Tracking
- Leading
- Type and/or Headline Groupings
- Alignment methods
- Work spacing
- Line spacing
- Type Relationships
- Type styles
- Type sizes
- Type weights
- Type Images (Selection and Design)
- Formal image
- Informal image
- Others
- Type Applications
- Ads
- Brochures
- Posters
- Logos
Effective Term: Full Academic Year 2019/20 |
|
-
DAR 112 - Graphic Design 4 Credits, 5 Contact Hours 3 lecture periods 2 lab periods
Basic principles of color and design as applied to the graphics industry. Includes creating focal points, unity, texture, space relationships, color control, color harmonies, and psychology of color.
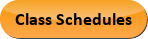
Course Learning Outcomes
- Create designs utilizing theory and principles of design.
- Create design controlling color and type to convey image.
- Produce designs that appeal to specific target audiences.
- Work within a professional environment meeting specifications and deadlines.
Outline:
- Focal Points
- Size
- Contrast
- Placement
- Unity
- Grouping
- Repetition
- Continuation
- Texture
- Specific
- Generic
- Space Relationships
- Dramatic break-up
- Negative space
- Asymmetrical balance
- Color Control
- Contrast
- Valve
- Saturation
- Framing colors
- Color Harmonies
- Monochromatic
- Analogous
- Complimentary
- Traditional
- Split-complimentary
- Sharing portions of a similar color
- Psychology of Color
Effective Term: Full Academic Year 2019/2020 |
|
-
DAR 115 - Digital Video Editing 4 Credits, 5 Contact Hours 3 lecture periods 2 lab periods
Introduction to non-linear editing on the computer. Includes historical development of editing, digital video and audio formats, techniques and theory of storytelling in editing, storytelling in various types, and organization for the edit.
Prerequisite(s): DAR 103 or concurrent enrollment.
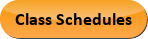
Course Learning Outcomes
- Identify historical development of editing.
- List various techniques of storytelling in editing.
- Demonstrate non-linear editing skills including importing, editing, exporting, and format considerations…
- Work within a professional environment meeting specifications and deadlines.
Outline:
- Historical Development of Editing
- Development of editing and how it affects storytelling
- The evolving post production environment
- Impact of digital media
- Digital Video and Audio Formats
- Identifying digital video formats
- Identifying digital audio formats
- Techniques and Theory of Storytelling in Editing
- Cut on action
- Cut on clean entry and clean exit
- Cut on motion
- Cut away from action
- Matching edits and avoiding jump cuts
- Montage editing
- Emerging trends in storytelling
- Storytelling in Various Types
- Narrative
- Advertising/commercials
- Music videos
- Documentary
- Social media
- Fundraising
- Instructional/educational/training
- Web
- News
- Organization for the Edit
- The concept of workflow
- Desktop environment (bins, timeline, tools)
- Ingest-sources
- Export – distribution, portfolio
Effective Term: Full Academic Year 2019/2020 |
|
-
DAR 120 - Applied Computer Graphics 4 Credits, 5 Contact Hours 3 lecture periods 2 lab periods
Introduction to computer graphics software. Includes current software, postscript illustration documents, paint and photo editing documents, desktop publishing documents, and introduction to graphic design concepts.
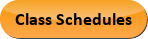
Course Learning Outcomes 1. Create and layout postscript illustration documents.
2. Create and layout paint and photo editing documents.
3. Create and layout desktop publishing documents.
4. Demonstrate basic layout techniques using graphics software. Outline:
- Current Software
- Terminology
- Tools
- Menus
- Postscript Illustration Documents
- Bezier curves
- Using strokes, fills, and gradients to define shape and form
- Paint and Photo Editing Documents
- Paint tools
- Masks
- Desktop Publishing Documents
- Typography
- Graphics
- Importing
- Introduction to Graphic Design Concepts
- Conceptualization
- Visualization
- Controlling areas of focus in design
Effective Term: Full Academic Year 2018/2019 |
|
-
DAR 122 - DeskTop Graphics: Adobe Illustrator 4 Credits, 5 Contact Hours 3 lecture periods 2 lab periods
Computer generated graphics and illustrations. Includes current Adobe Illustrator software, computer graphics hardware, documents, and professional environment.
Prerequisite(s): DAR 120
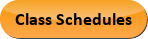
Course Learning Outcomes
- Demonstrate the use of current Adobe Illustrator software.
- Create desktop graphics documents using bezier curves, templates, text, color, gradients, patterns, graphs, placed images, and layers.
- Demonstrate the use of computer graphics hardware, including input and output devices.
- Utilize working in a professional environment with specifications and deadlines.
Outline:
- Current Adobe Illustrator Software
- Terminology
- Tools
- Menus
- Setup
- Preferences
- Documents
- Bezier curves
- Templates
- Text
- Color
- Gradients
- Patterns
- Graphs
- Placed images
- Layers
- Computer Graphics Hardware
- Input devices
- Mouse
- Keyboard
- Scanner
- Output devices
- Printer
- Central Processing Unit (CPU)
- Monitor
- Printer
- Professional Environment
- Specifications
- Deadlines
Effective Term: Fall 2016 |
|
-
DAR 124 - Writing for Film and Television 3 Credits, 3 Contact Hours 3 lecture periods 0 lab periods
Examining dramatic writing in visual mediums and creating the story for the screen. Includes story origins and formats, story structure, elements of story, preproduction, writing for alternative media, working in the film and television industry, writing processes, and criticism.
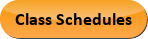
Course Learning Outcomes
- Describe storytelling in visual mediums both historically and today.
- Identify story structure when viewing various visual mediums.
- Describe the techniques of scriptwriting and the vocabulary for discussing story.
- Work within a professional environment meeting specifications and deadlines.
Outline:
- Story Origins and Formats
- Visual storytelling
- Screenplay history
- Page layout
- Screenwriting software
- Story Structure
- Three act structure
- Dramatic action
- Structural analysis
- Element of Story
- Plot
- Character
- Setting
- Theme
- Dramatic Traditions
- Tragedy
- Comedy
- Ancient genres of comedy
- Modern genres of comedy
- Experimental drama
- Preproduction
- Pitching
- Synopses
- Treatments
- Script readers
- Writing for Alternative Media
- Writing for games
- Writing drama for the web
- Writing for comics
- Writing for radio/podcasts
- Working in the Film and Television Industry
- The Writer’s Guild of America
- Earnings in film vs. television
- Collaborators, casts, and crew
- Adaptation
- Adaptation as interpretation
- Authenticity
- Writing Processes
- Physical techniques
- Time management
- Finding and maintaining inspiration
- Criticism
- Giving constructive feedback
- Separating oneself from one’s work
- Being specific
Effective Term: Full Academic Year 2019/2020 |
|
-
DAR 125 - Digital Cinematography I 4 Credits, 6 Contact Hours 2 lecture periods 4 lab periods
Principles and techniques of digital cinematography production. Includes digital video camera, camera and shooting competencies, lighting and composition, and working as a team.
Prerequisite(s): DAR 103 and DAR 115 or concurrent enrollment. Information: This course will require additional expenses for supplies in addition to course and lab fees.
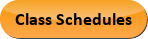
Course Learning Outcomes
- Identify the various digital video cameras, formats, and specifications.
- Demonstrate competencies with various video cameras, and their functions including aperture, shutter speeds, and electronic light metering.
- Describe the use of elements of lighting and composition in storytelling.
- Demonstrate the ability to function as a member of a production team in a professional environment.
Outline:
- Digital Video Camera
- Camera manual and specifications
- Formats
- Memory and data considerations
- Camera and Shooting Competencies
- Lenses
- Shutter speeds
- Depth of field
- Lighting and exposure
- How Lighting and Composition Affects Storytelling
- Visual language of aesthetics
- Expressing intentions visually
- Images in context
- Working as a Team
- Crew duties
- Safety
Effective Term: Full Academic Year 2019/2020 |
|
Page: 1
| 2
| 3
| 4
| 5
| 6
| 7
| 8
| 9
| 10
| 11
-> 15 |
|
|