|
May 09, 2025
|
|
|
|
2023-2024 College Catalog [ARCHIVED CATALOG]
|
CIS 131 - Programming and Problem Solving II 4 Credits, 4 Contact Hours 4 lecture periods 0 lab periods
Continuation of CIS 129 . Includes data structures and data representation, complex problem solving, procedural abstraction, and complex arrays with structured elements. Also includes object oriented programming, exception handling, file input and output, debugging, and testing.
Prerequisite(s): CIS 129 Gen-Ed: Meets AGEC-S Options requirement; Meets CTE - Options requirement
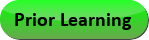.png)
Course Learning Outcomes
- Build data structures such as stacks, queues and trees .
- Build programs that use recursion to solve simple problems.
- Solve complex problems through programming.
- Design programs using sorting and searching algorithms.
- Create Object Oriented Programs with inheritance, constructors and destructors.
Outline:
- Revisit Data Structures and Data Representation
- Elementary data types
- Character
- String
- Integer
- Real
- Boolean
- Structured data types and data abstraction
- File
- Record
- Array
- Single dimension
- Multidimensional
- Procedural Abstraction
- Procedure code module
- Statement extension
- Actions upon objects
- Recursion
- Data Files
- Reading input from data files
- Building new output data files
- Adding to existing output data files
- Adding and random updating of data records in binary typed data files
- Object Oriented Programming
- Creating complex classes using abstract classes, inheritance and composition
- Creating overloaded methods, constructors and destructors
- Data encapsulation
- Exception Handling
- Creating programs using simple exception handling, propagation
- Using generic and specific try-catch blocks
- Using “finally block”
- Debugging and Testing
- Creating test data using black box, white box, robustness testing methods
- Creating a test plan
- Boundary conditions
- Program debugging
- Creating a program which can be debugged
- Using concepts such as step into, step over, breakpoints, run and continue
- Troubleshooting a problem to pinpoint errors
- Document program
- Complex Arrays with Structured Elements
- Sorting
- Searching
- Divide and conquer algorithms
- Solving complex problems/create programs
- Introducing complexity: best case, worst case, and average case
- Solving problems in pseudocode and/or flowcharts
- Input file data to print, input file data to array and sort array, array to print
- Translating the pseudocode/flowchart into code
Effective Term: Fall 2023
|
|