|
Dec 21, 2024
|
|
|
|
2022-2023 College Catalog [ARCHIVED CATALOG]
|
ENG 276IN - Computer Programming for Engineering Applications II 3 Credits, 5 Contact Hours 2 lecture periods 3 lab periods
Continuation of ENG 175IN . Advanced programming in C for engineering applications. Includes review of C programming, memory concepts, algorithms and analysis, and an introduction to C++
Prerequisite(s): ENG 175IN
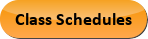
Course Learning Outcomes
- Demonstrate the ability to develop, debug, and test large software projects in both the C and C++ programming languages to solve engineering problems.
- Demonstrate the ability to analyze, compare, and select appropriate data structures and associated algorithms for engineering applications.
- Demonstrate software engineering best practices and object-oriented design and programming.
Performance Objectives:
- Preprocess, compile and/or link programs.
- Use project management tools (e.g. IDEs(Integrated Development Environment), Cmake, etc).
- Use libraries and code re-use across executables.
- Debug compiled programs.
- Understand and use applications with pointers and memory addresses.
- Pass by value and pass by reference.
- Allocate and manage memory.
- Distinguish between stack and heap in software programs.
- Utilize trees, queues, stacks, heaps, and graphs.
- Use appropriate algorithms for sorting, searching, hashing, traversals, and shortest path.
- Design, analyze and implement algorithms.
- Asymptotic analysis of algorithms.
- Construct/delete objects in C++.
- Use basic C++ operations and commands.
- Use Standard Template Library (STL) Classes.
Outline:
- Review of C Programming
- Create source code
- Link and/or compile main with other functions
- Execute programs
- Debugging errors in program execution
- Memory Concepts
- Allocating memory using malloc and/or calloc, realloc, and free
- Determining memory requirements from sizing of data
- Using the stack and heap
- Algorithms and Analysis
- Construction /use of binary trees, stacks, heaps, graphs
- Algorithms/methods of data for sorting, searching, traversing, hashing, shortest path analysis
- Design and implementation of applied algorithms
- C++ Introduction
- Objects and classes
- Commands unique to C++ ie, cin, cout, others.
- STL classes
|
|