|
2022-2023 College Catalog [ARCHIVED CATALOG]
|
ENG 175IN - Computer Programming for Engineering Applications I 3 Credits, 5 Contact Hours 2 lecture periods 3 lab periods
Programming in C with emphasis on numerical applications in engineering. Includes structure of C programs; data types, operations, and basics of C; selection, repetition, arrays, functions, and data files.
Prerequisite(s): MAT 189
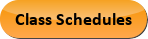
Course Learning Outcomes
- Demonstrate the ability to develop and test software projects using the C programming language to solve engineering problems.
- Demonstrate the ability to apply the compilation process, tools, and basic debugging techniques.
- Demonstrate the ability to design and organize programs into separate functions to maximize code reuse and maintainability.
Performance Objectives:
- Define integer, floating point, double precision, character, string, array, and structure data types and describe their storage methods.
- List the arithmetic, assignment, relational, logical, and increment/decrement operators and demonstrate their application.
- Demonstrate the use of the #define and #include preprocessor commands.
- Write statements using the print f() function for formatted output including the use of escape sequences.
- Write statements using the scan f() function for data input.
- Write comment statements.
- Write statements using standard C library mathematical, character, and string processing functions.
- Write user defined functions.
- Write if-else and switch selection statements.
- Write while, for, and do repetition statements.
- Explain the scope of variables in a program.
- Explain auto, register, and static variable classes.
- Write program segments to declare, open, read, write, and close files using standard C file library functions.
- Distinguish between text and binary files.
- Define the term pointer and demonstrate use of the & address operator and the * indirection operator.
- Develop structured programs, applying a top-down approach, to solve practical engineering problems by numerical methods.
- Analyze errors inherent in floating point representation of data.
- Analyze error propagation in floating point calculations.
- Discuss cost effectiveness considerations of program complexity, efficiency, maintainability, and programmer times versus execution time tradeoffs.
- Demonstrate use of the conditional operators and their application.
- Demonstrate use of the bit shift operators and their application.
- Distinguish between random and sequential file access.
- Demonstrate the use of macros.
- Explain the use of recursively called functions.
- Demonstrate the use of linked lists.
- Demonstrate the use of binary trees.
Outline:
- Structure of C Programs
- Functions and program modularity
- Main() function
- Print f() function
- Scan f() function
- Top-down program development
- Data Types, Operations, and Basics of C
- Integer
- Floating point and double precision
- Character
- Escape sequences and conversion control sequences
- Arithmetic operations
- Operator precedence and associativity
- Variables and declaration statements
- Assignment statements
- Formatted output
- Mathematical library functions
- Type conversion rules
- Symbolic constants
- Selection
- Relational expressions and logical operators
- If-else statements
- Nested if statements and if-else chains
- Switch statements
- Repetition
- Increment/decrement operators
- While statements
- Break, continue, and null statements
- For statements
- Do statements
- Nested loops
- Arrays
- One dimensional arrays
- Input, output, and initialization of array values
- Multidimensional arrays
- Functions
- Definition, declaration, and calling of functions
- Standard library functions
- Arrays as arguments
- Variable scope
- Variable storage classes
- Data Files
- Opening, reading, writing, and closing files
- Standard device files
- Random access files (optional)
- Text and binary files (optional)
|
|