|
Dec 03, 2024
|
|
|
|
2024-2025 College Catalog
|
CIS 269 - Data Structures 3 Credits, 3 Contact Hours 3 lecture periods 0 lab periods Advanced topics in computer science and programming in C++ Includes software engineering concepts and theory, memory management, inheritance, overloading, abstract classes, review of C++ stacks, queues, recursion, and dynamic abstract data structures. Also includes source control, templates, hash tables, sort and search algorithms, file handling and streams, trees, graphs and networks.
Prerequisite(s): CIS 131 and CIS 278 . Gen-Ed: Meets AGEC Options requirement; Meets CTE - Options requirement
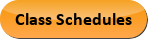
Course Learning Outcomes
- Implement composite data types, structures, and classes.
- Code search algorithms.
- Determine relative timing complexity (Big O Notation) relating to the sort and search algorithms.
- Code various complex algorithms with memory management components, classes, and pointers.
- Code breadth first and depth first algorithms.
- Create templates.
- Write to multiple file handles and streams in a program.
Outline:
- Software Engineering Concepts and Theory
- Review of C++
- Classes, types and declarations
- Operators
- Control flow statements
- Pointers – single and multi-level indirection
- Functions – including function pointers
- Inheritance, overloading
- Abstract classes
- Streams and file handles.
- Stacks and Queues
- Add an element to a stack or queue
- Delete an element from a stack or queue
- Use a circular array to simulate a stack or queue
- Simulate a stack or queue using a linked list
- Stack overflow
- Recursion
- Divide and conquer
- Backtracking
- Removal of
- Dynamic Abstract Data Structures
- Linked lists
- Trees
- Binary
- Binary search trees
- AVL trees (balanced)
- Sort and Search Algorithms
- Quick sort
- Merge sort
- Heap sort
- Radix sort
- Hash tables
- Introduction to Big O notation and analysis
- Memory Management
- Destructor calling order
- Memory leaks
- Garbage collection
- Hash Tables
- Hash functions
- Types of hash tables
- Addressing schemes
- Analysis of complexity
- Templates
- Creating a template
- Extending an existing template
- Graphs, Trees and Networks
- Adjacency matrix
- Depth-first and breadth-first search
- Shortest path algorithm
Effective Term: Fall 2023
|
|