|
Dec 03, 2024
|
|
|
|
2024-2025 College Catalog
|
CIS 278 - C++ and Object-Oriented Programming 3 Credits, 4 Contact Hours 2 lecture periods 2 lab periods Concepts and implementation of object-oriented programming and design using C++ Includes the language syntax of C++ applications using C++ objects to solve information systems problems, and class libraries created for reuse and inheritance.
Prerequisite(s): CIS 131 Gen-Ed: Meets AGEC Options requirement; Meets CTE - Options requirement
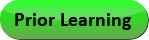.png)
Course Learning Outcomes
- Develop programs using both C++ built-in classes and user-defined classes.
- Integrate the concepts of abstraction, inheritance, composition and polymorphism into C++ programs.
- Write programs in C++ which solve information systems problems and which show increased productivity by taking an object-oriented approach.
- Create and use collections (arrays and vectors) of user-defined objects.
- Demonstrate Class Libraries
- Write data to and from files.
Outline:
- Introduction and Overview
- Benefits of object-oriented (O-O) methods
- Structured vs. O-O approaches
- O-O design improvements
- Reusability
- Reliability
- Maintainability
- Encapsulation: integrating object state data, as represented by instance variables, with the code that operates upon it
- Features of object-oriented programs
- Strong typing and type hierarchies
- Classes for encapsulation and information hiding
- Using C++
- Introduction to C++
- Design goals of C++
- C++ = C + strong typing + classes
- C++ syntax
- C++ structs as a step on the path towards classes
- Classes as user-defined types in C++
- O-O programming in C++
- Using C++ built-in classes such as strings
- C++ types, references, and friends
- Object creation (constructors, copy constructors, destructors)
- Inheritance and derived classes
- Composition: using objects of other classes as instance variables within a class
- Dynamic storage allocation of objects
- Polymorphism
- Collections of objects
- Dynamic function binding using virtual and pure virtual functions
- Advanced C++ Features and the Future of O-O
- Reusable libraries
- Files, stream, and I/O libraries
- Designing a library
- The Standard Template Library
- Container classes
- Iterators
- Algorithms
- Generic Libraries
- Using a container class
- Class categories as mechanisms
Effective Term: Fall 2023
|
|