|
Jul 02, 2025
|
|
|
|
2022-2023 College Catalog [ARCHIVED CATALOG]
|
CIS 129 - Programming and Problem Solving I 4 Credits, 4 Contact Hours 4 lecture periods 0 lab periods
Introduction to personal and business computer systems. Includes components of a computer system; advantages and disadvantages of programming languages; traditional
languages, native code and object-oriented concepts; source code versus executable code; and data structures and data representation. Also includes language statements; expressions
components; control structures; problem-solving techniques; program test data, debugging and termination; and solving simple problems and creating programs.
Prerequisite(s): MAT 095 or MAT 097 or concurrent enrollment, or placement into MAT 151 . Gen-Ed: Meets AGEC-S Options requirement
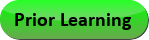.png)
Course Learning Outcomes
- Develop programs using control structures.
- Develop programs using repetition structures.
- Design programs using arrays/lists.
- Create programs, using functions to solve problems.
- Demonstrate the ability to use object-oriented concepts such as encapsulation, constructors, methods, and properties
Outline:
- Components of a Computer System
- Types of computers
- Hardware
- CPU
- Input/output devices
- Storage
- Memory
- Computer codes and numeric representation – simple conversions
- Advantages and Disadvantages of Programming Languages
- Machine code
- Low level programming languages, assemblers, assembly languages
- Overview of Traditional Interpreted and Compiled
- C and C++
- Java
- Python
- Source Code Versus Executable Code
- Language interpreters (run-time translators)
- Assemblers (1-to-1 translators)
- Compilers (1-to-N translators)
- Data Structures and Data Representation
- Variables
- Global
- Local
- Elementary data types
- Character
- String
- Integer
- Floating
- Boolean
- Identify and explain the difference between:
- Data types
- Memory addresses
- Variables types
- Literals
- Constants
- Number base systems (binary, decimal and hexadecimal)
- Floating point
- Decimal data representation
- Direct addressing
- Relative addressing
- Identify and explain structured data types and data abstraction
- File
- Record
- Array/list
- Single dimension
- Multidimensional
- Language Statements
- Computer language statements, syntax, and semantics
- Input/output statements
- Assignment statements
- Elementary language statements and structured language statement
- Assignment and unconditional statements
- Selection and looping statements
- Expressions Components
- Operators, operands, and results
- Unary
- Binary
- Simple types
- Arithmetic
- Logical
- Relational
- Result
- Unconditional (not Boolean)
- Conditional (Boolean)
- True
- False
- Control Structures
- Three basic control structures and their sub-constructs
- Sequence
- Selection (Decision, If Then/Else) – case statement available in some languages
- Repetition (Looping, Iteration) – for statement available in some languages
- Problem Solving Techniques
- Top-down design – unitizing a problem into modules
- Step-wise refinement of modules
- Control modules
- Process modules
- Cohesion and coupling concept
- Systems and program mapping tools
- System flow
- Structure chart
- Detail program logic
- Program hierarchy – tracing design output to its source
- Input data
- Algorithm
- Elementary program design structure model
- Setup
- Process
- Wrap-up
- Solving simple problems using algorithms and a program design language
- Flowcharting
- Pseudo code
- Translating Pseudo Code into Python
- Program Test Data and Termination
- Program test data
- Limits/range testing
- Max/min data type testing
- Logic (selection) testing all paths
- Program errors
- Design errors
- Syntax compilation errors
- Semantic run-time errors
- Program termination and return codes
- Normal termination
- Abnormal program termination
- Return codes and use of return codes
- Desk Checking and Debugging
- Desk check on paper
- Breakpoint
- Step into
- Step over
- Variable watch
- Solve Simple Problems and Create Programs Using Java, Python, or C#
- Solve simple problem into PDL
- Keyboard data entry to print, keyboard entry to array/list, and array/list to print
- Arithmetic calculations, counters, accumulators
- Cross footing and accumulator totaling
- Conditional statements
- Boolean expressions
- Print using:
- Unformatted output
- Formatted output
- Report headings
- Report footers
- Translate the PDL into code
- Test the program code
- Debug the program
- Explain Object-Oriented Concepts such as Encapsulation, Constructors, Methods, and Properties
- Object-oriented programming
- Objects
- Methods
- Properties
- Private
- Public
- Constructors
- Encapsulation
|
|