|
Jul 02, 2025
|
|
|
|
2021-2022 College Catalog [ARCHIVED CATALOG]
|
CIS 265 - The C Programming Language 3 Credits, 3 Contact Hours 3 lecture periods 0 lab periods
Principles and syntax of ANSI Standard C and many of the common library functions. Includes writing C programs in portable code to facilitate systems programming concepts.
Prerequisite(s): CIS 131 Recommendation: CIS 250 . If any recommended course is taken, see a financial aid or Veteran’s Affairs advisor to determine funding eligibility as appropriate.
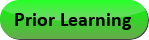.png)
Course Learning Outcomes
- Identify the difference between ANSI C, C99, and C11.
- Write programs in C with various scope (local, global, and external).
- Write programs in C with multi-dimensional arrays.
- Write programs in C with pointers, and structs.
- Write programs using several files.
- Solve complex problems using C as the language for implementation.
Performance Objectives:
- Contrast ANSI C, C99, and C11.
- Discuss the syntax of the C language, including the type specifications and operators and their order of precedence.
- Write programs in C to demonstrate scope, multi-dimensional arrays, pointers, and structs.
- Contrast C with other procedural programming languages.
- Design solution to systems level problems using C as the language for implementation.
Outline:
- History of the C language
- UNIX
- ANSI C
- C99
- C11
- C-based languages
- Syntax of the C language
- Constants and variables
- Local variables
- Global variables
- External variables
- Operators and expressions
- Precedence
- Associativity
- Control flow operators
- Selection statements
- Repetition
- Scope and Storage Classes in C
- Declarations
- Invocation
- Functions - parameter passing (by value and by reference)
- Typing Aspects of C
- Built-in types
- Derived types
- Arrays (single and double-dimensional; fixed and variable length)
- Structs (including anonymous)
- Unions (including anonymous)
- Singly Linked Lists
- Pointers
- Single and multiple reference levels
- Passing pointers as arguments
- Pointer arithmetic
- Pointers and arrays (including changes made in C11 to make pointers safer)
- Preprocessor
- Defines and macros
- Include files and header
- Input/Output
- Keyboard and terminal
- Disk I/O
- Formatted input/output
Effective Term: Full Academic Year 2021/22
|
|