|
May 10, 2025
|
|
|
|
2023-2024 College Catalog [ARCHIVED CATALOG]
|
ENG 209IN - Introduction to MATLAB and Python 3 Credits, 5 Contact Hours 2 lecture periods 3 lab periods
Fundamental knowledge and practical abilities in MATLAB and Python utilizing technical numerical computations in engineering courses. Includes script files and functions; creating and performing operations on arrays; creating two and three-dimensional plots; implementation of programming and algorithms; applying numerical methods including integration and differentiation; linear and nonlinear problem-solving.
Prerequisite(s): MAT 220
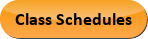
Course Learning Outcomes
- Use MATLAB and Python for interactive computations.
- Use MATLAB and Python’s built-in computational and logical operators and functions
- Implement mathematical operations on one and two-dimensional arrays, including solving linear systems of equations, using Matlab and Python.
- Apply programming skills and techniques to solve computational and engineering problems.
- Evaluate various methods for algorithm implementation.
- Write program code that reads and writes data.
- Generate 2-dimensional and 3-dimensional plots in MATLAB and Python and export them for use in reports and presentations.
- Create functions in MATLAB and Python.
- Implement various numerical analysis techniques, such as regression, interpolation, differentiation, and integration.
- Use Python objects including strings, lists, tuples, and loops.
- Compare strengths of MATLAB and Python to decide which is more useful for a given situation.
Outline:
- Introduction to MATLAB
- Starting MATLAB and MATLAB windows
- Working in the command window
- Arithmetic operations with scalars
- Relational and logical operations
- Elementary built-in math functions
- Variables, Arrays, and Data Types
- Single-value variables
- Constructing one and two-dimensional arrays
- Array indexing and referencing
- Operations on arrays
- Arithmetic, relational, and logical operators
- Built-in math functions
- Vectorized computations
- Character strings
- Struct and cell arrays
- Functions and Script Files
- Creating, saving, and running a script file
- User-defined functions
- Global and local variables
- Anonymous functions
- Function name as input
- Programming in MATLAB
- Branching
- Iteration
- Recursion
- Sorting and searching algorithms
- [Optional] Complexity
- Operation counting and Big-O notation
- Measuring performance
- Reading and Writing Data
- Importing and exporting data
- Output commands
- Two and Three-Dimensional Plots
- Two-dimensional plots
- The plot and flplot commands
- Continuous and discrete plots
- Plotting multiple plots in the same graph
- Plotting multiple graphs in the same figure
- Plots with logarithmic axes
- Three-dimensional plots
- Mesh and surface plots
- The view command
- Customization and annotation
- Linear Algebra
- Operations on vectors
- Norm
- Dot and cross products
- Inverse
- Determinant
- Matrix multiplication
- Solving systems of linear equations
- Regression and Curve Fitting
- Least squares regression theory
- Applying regression
- Nonlinear curve fitting
- Exponential curve
- Linear interpolation
- Polynomial interpolation
- Numerical Differentiation and Integration
- Difference quotients for first and second derivatives
- Built-in MATLAB differential operators
- Built-in MATLAB integrators
- Numerical integration with vectorized computation
- Introduction to Python
- Installing Python
- Working in the command line and the Python shell
- Computing with numbers and variables
- Relational and logical operators
- Working with Python objects
- Strings
- Lists
- Tuples
- Loops
- Control flow
- File input/output
- Functions
- Basic plotting in Python
- Line and scatter plots
- Annotation and customization
- Special plots: polar, histograms
- Matplotlib
- Line and scatter plots
- Annotation and customization
- Multiple plots
- Special plots
- Contour plots
- Three-dimensional plots
- Optional Python topics
- IPython and Jupyter Notebook
- Numpy
Effective Term: Fall 2023
|
|